How to Remove an HTML Element Using JavaScript
- What Is Element in JavaScript
- Two Types of Tags in an HTML Element
- How to Add Element in JavaScript
- How to Remove an Element in JavaScript
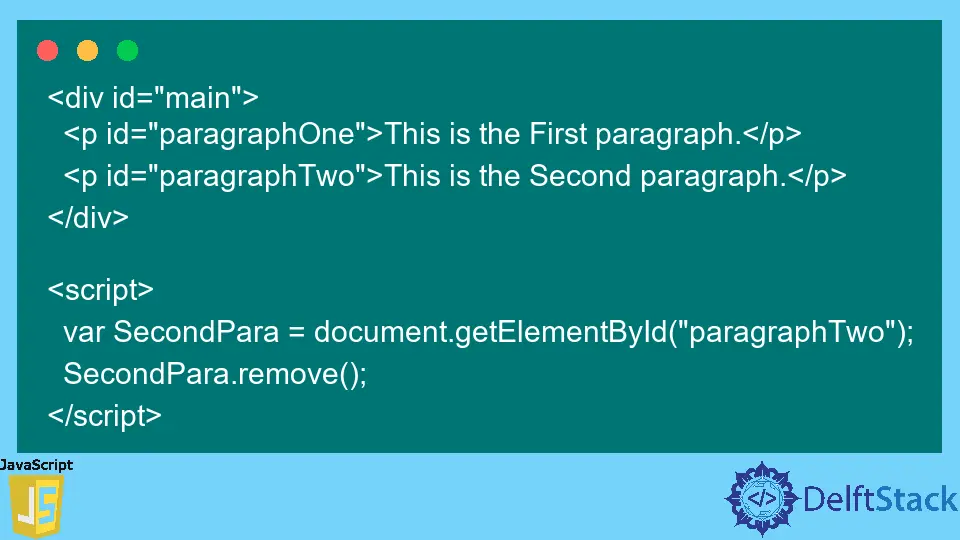
This tutorial article will discuss an element in JavaScript, how to add an element in JavaScript, and how to remove an existing element in JavaScript.
The following questions will be addressed in this article:
- What is an element in JavaScript
- How to add elements in JavaScript
- How to remove an element in JavaScript
What Is Element in JavaScript
A JavaScript element is a Node in the HTML Document. HTML elements are displayed using Tags.
Two Types of Tags in an HTML Element
A tag is used to add an HTML Element into the document. There are two types of tags which are:
- Double Tags are composed of an opening tag and closing tag.
<div>
Some Content here
</div>
<div>
represents the opening tag, and </div>
represents the closing tag.- Self Closing Tag is a single tag. There are no separate opening and closing tags.
<img src="SomeLinkHere" alt="" />
How to Add Element in JavaScript
A new HTML element can be inserted into the Document object model using JavaScript.
To do this, we will need to create a new element on the document and then create a text node for it (if needed) append that to the created element.
Finally, append the created element in an element already existing in the Document Object Model.
<div id="main">
<p id="paragraphOne">This is the First paragraph.</p>
<p id="paragraphTwo">This is the Second paragraph.</p>
</div>
<script>
const ThirdParagraph = document.createElement("p");
ThirdParagraph.id = "paragraphThree";
const TextNode = document.createTextNode("This is the Third paragraph.");
ThirdParagraph.appendChild(TextNode);
const newElement = document.getElementById("main");
newElement.appendChild(ThirdParagraph);
</script>
The main container with an id of main
has two paragraphs with paragraphOne
and paragraphTwo
.
A new paragraph element is created on the document, and an id of paragraphThree
has been attached to the JavaScript.
Furthermore, a text Node has been created and appended to the Paragraph element. The ThirdParagraph
will be added to the main
element.
<div id="main">
<p id="paragraphOne">This is the First paragraph.</p>
<p id="paragraphTwo">This is the Second paragraph.</p>
<p id="paragraphThree">This is the Third paragraph.</p>
</div>
Output. See the working code segment.
The same process can be repeated with JavaScript libraries, e.g., jQuery.
To add a new element into the DOM using jQuery, you would access the container you want to add the element to and use the append
keyword to add it to the container i.e
<div id="main">
<p id="paragraphOne">This is the First paragraph.</p>
<p id="paragraphTwo">This is the Second paragraph.</p>
</div>
<script>
$("#main").append("<p id='paragraphThree'>This is the Third paragraph.</p>");
</script>
The output of the code mentioned above segment can be seen with this link.
How to Remove an Element in JavaScript
To remove an element from the Document Object Model, you need to access the element and remove it.
<div id="main">
<p id="paragraphOne">This is the First paragraph.</p>
<p id="paragraphTwo">This is the Second paragraph.</p>
</div>
<script>
var SecondPara = document.getElementById("paragraphTwo");
SecondPara.remove();
</script>
The output of the code segment can be seen with this link.
The element is accessed by the id
, and the remove function an element is invoked on it, which will remove it from the DOM.
Similarly, the above result can be achieved using jQuery.
<div id="main">
<p id="paragraphOne">This is the First paragraph.</p>
<p id="paragraphTwo">This is the Second paragraph.</p>
</div>
<script>
$("#paragraphTwo").remove();
</script>