How to Insert After Element in JavaScript
-
Use the
insertBefore()
Method to Add an Element in JavaScript -
Use the
insertAfter()
Method to Add an Element in JavaScript - Conclusion
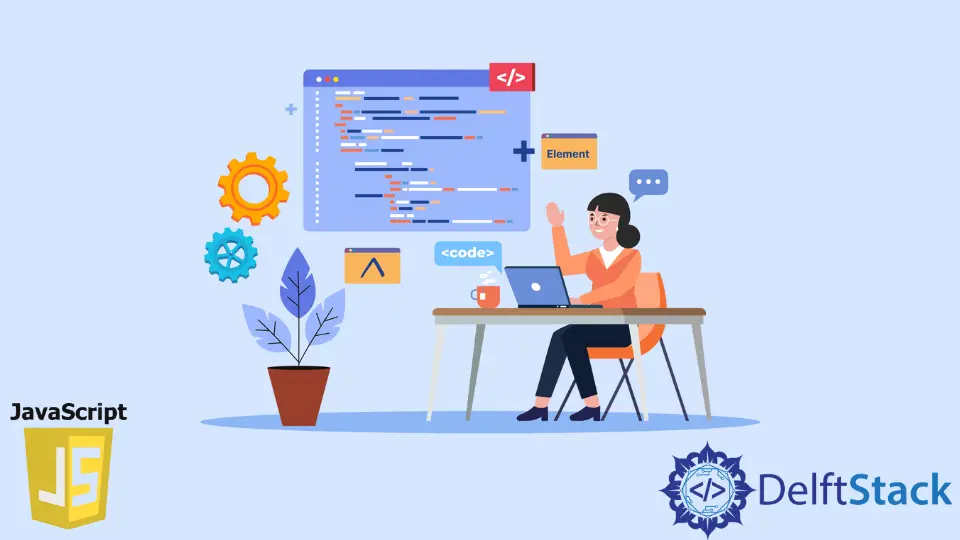
The DOM API in JavaScript does not permit inserting an element after an existing feature. Follow these steps to place an element after an existing element in the DOM tree.
- First, choose the existing element’s parent node.
- Then, insert the new element before (
insertBefore()
) the old element’s next sibling (nextSibling
).
Use the insertBefore()
Method to Add an Element in JavaScript
Syntax:
insertBefore(newNode, existingNode)
There are two parameters to the procedure. The new node or new element is the first parameter.
Before the new node (element), the existing node or element will be put in the second parameter. However, I want the new node to be added after the element. There’s a ruse here.
Let’s pretend I have two section elements on my page, and I want to add an r
element (or any other element) after the first (section).
Code:
<!DOCTYPE html>
<html>
<head>
<style>
* { font-family: Calibri; font-size: 20px; }
section { border: solid 1px #ddd; padding: 10px; margin: 5px 0;}
r { color: green; }
</style>
</head>
<body>
<h2>
A new element is inserted after the "header" section using "insertBefore() method in JavaScript.
</h2>
<section id='header'>
Head Section
</section>
<section id='footer'>
Foot Section
</section>
</body>
<script>
let add_new_element = () => {
let header = document.getElementById('header');
let newElement = document.createElement('r');
newElement.innerHTML = 'Content inside r element';
header.parentNode.insertBefore(newElement, header.nextSibling);
}
add_new_element();
</script>
</html>
On my website, I have a header and a footer. I’m dynamically constructing an r
element and then adding it after the first section or title.
Output:
In most cases, the insertBefore()
technique will place the new r
element before the header element. The r
is added after the header section.
Use the insertAfter()
Method to Add an Element in JavaScript
Let’s see another example. The logic is demonstrated via the insertAfter()
function.
function insertAfter(newNode, existingNode) {
existingNode.parentNode.insertBefore(newNode, existingNode.nextSibling);
}
Assume you have the following items on your list.
<ul id="Fruits">
<li>Apple</li>
<li>Banana</li>
<li>Coconut</li>
</ul>
After the last list item, the following code creates a new node.
let menu = document.getElementById('Fruits');
// creatING a new li node
let li = document.createElement('li');
li.textContent = 'Grapes';
// inserting a new node after the last list item
insertAfter(li, Fruits.lastElementChild);
How it works is as follows.
- To begin, use the
getElementById()
function to select theul
element by its id (Fruits
). - Use the
createElement()
method to create a new list item. - Place a list item element after the last list item element using the
insertAfter()
method.
Full Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript insertAfter() Demo</title>
</head>
<body>
<ul id="Fruits">
<li>Apple</li>
<li>Banana</li>
<li>Coconut</li>
</ul>
<script>
function insertAfter(newNode, existingNode) {
existingNode.parentNode.insertBefore(newNode, existingNode.nextSibling);
}
let menu = document.getElementById('Fruits');
// creatING a new li node
let li = document.createElement('li');
li.textContent = 'Grapes';
// inserting a new node after the last list item
insertAfter(li, Fruits.lastElementChild);
</script>
</body>
</html>
Output:
Conclusion
The insertAfter()
method hasn’t been implemented in JavaScript DOM yet. To insert new node as a child of a parent node, use the insertBefore()
function and the nextSibling
attribute.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn