How to Create Element With Class in JavaScript
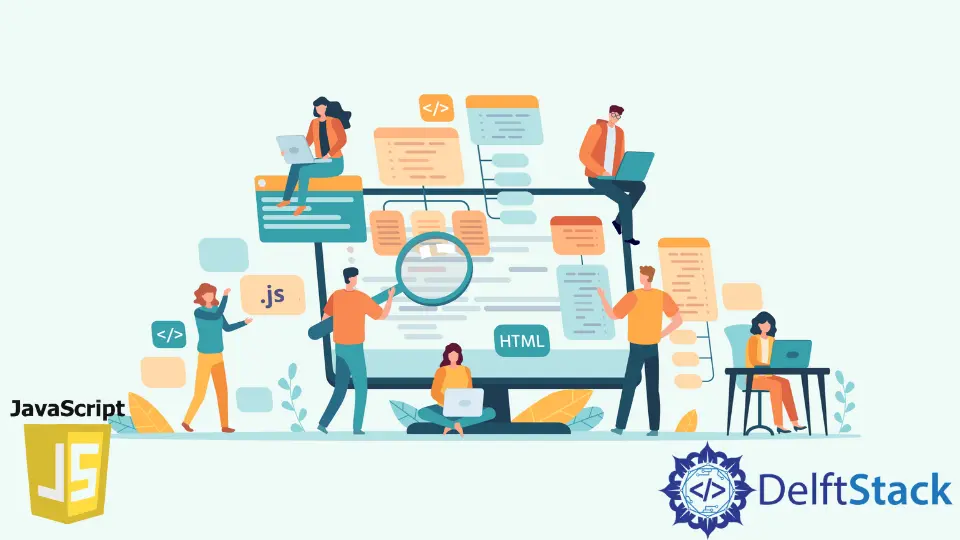
This article will generate an HTML element using a JavaScript function; we will call the function on the button click event and create an element with class.
Create Element in JavaScript
document.createElement()
is a default method which accepts parameter of HTML tag name as string and create an HTML element type node.
We can create any HTML element and attach it to the DOM tree by using this. We can assign id, content, and CSS for styling and append that element to the main body of the HTML page.
Basic syntax:
let myElement = document.createElement(anyTag);
Create Element With Class in JavaScript
We can create an HTML element with class by using classList.add()
method. We can also add more than one class to an element using that method.
Method add()
pass over the class if it is already present on the HTML element. We can use the appendChild()
method to append an element node to the main body of HTML.
Syntax with class:
element.classList.add('bg-red', 'text-lg')
Example:
<!DOCTYPE html>
<html>
<style>
.mystyle {
background-color: yellow;
padding: 10px;
}
</style>
<body>
<h1>Delftstack learning</h1>
<h2>Create element with class using JavaScript</h2>
<p>Click the button to create an element:</p>
<button onclick="createFunction()">Create</button>
<script>
function createFunction() {
let myDiv = document.createElement('div'); // element creation
myDiv.classList.add('mystyle'); // adding class
let content = document.createTextNode('This is a text content of an element!'); // text content
myDiv.appendChild(content); // append text content to element
document.body.appendChild(myDiv) // append element to body
}
</script>
</body>
</html>
In the HTML source above, we have created a button
element, and on the click event of that button, we have called createFunction()
. In that function, we are creating a div
element using document.createElement('div')
method and storing it in variable myDiv
.
Then, we added class mystyle
to that element by using myDiv.classList.add('mystyle')
method and added text content to display using document.createTextNode()
and append to myDiv
.
Finally, we append element to the main body of HTML source using document.body.appendChild(myDiv)
. You can save the above source with the extension of html and open it in the browser and test the example.