How to Get onClick Button ID in JavaScript
-
Get Clicked Button ID With the
This.id
Method in JavaScript -
Get Clicked Button ID With the
Event.target.id
Method in JavaScript -
Get Clicked Button ID With the
addEventListener
Function in JavaScript - Get Clicked Button With jQuery
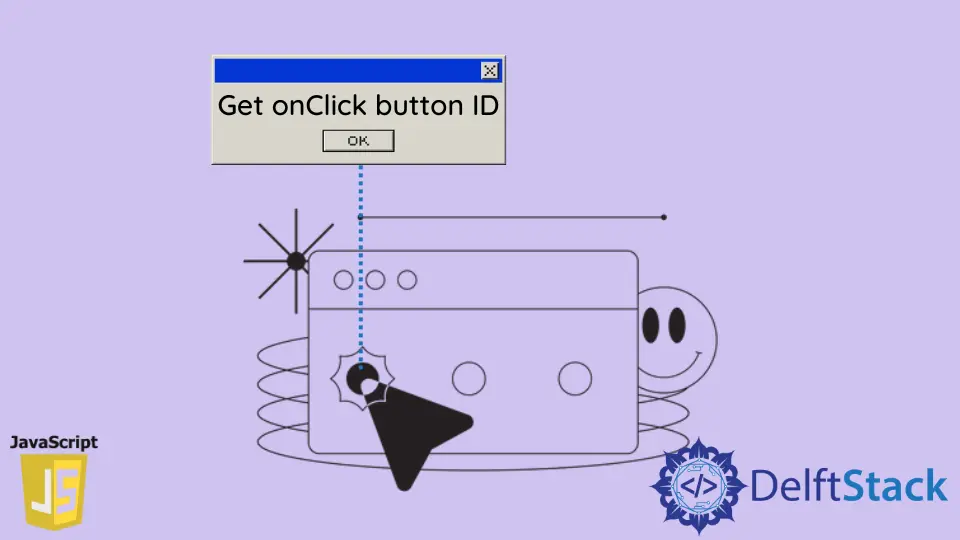
This tutorial presents how to get the ID of a clicked button in four different methods. These methods are this.id
, event.target.id
, addEventListener
, and jQuery.
Get Clicked Button ID With the This.id
Method in JavaScript
You’ll do this by creating a function that gets activated when you click the button. So when you click the button, the function will receive the button ID via this.id
.
Although the value of this
depends on how it’s called. In this case, it’ll refer to the button and its properties, like the button ID.
<body>
<main>
<button id="first_button" onclick="getClickID(this.id)">First Button</button>
<button id="second_button" onclick="getClickID(this.id)">Second Button</button>
<button id="third_button" onclick="getClickID(this.id)">Third Button</button>
</main>
<script type="text/javascript">
function getClickID(clickID) {
alert(clickID);
}
</script>
</body>
Output:
We have three buttons with different IDs with an onclick
event attribute in the code block above. The value of the onclick
event attribute is a function whose argument is this.id
or the button ID.
Get Clicked Button ID With the Event.target.id
Method in JavaScript
You can get a button ID during a click event thanks to the target
property of the Event
interface. The target
property refers to the button element that got the click event from the user.
At the same time, you can get the ID of the button from the target
property via target.id
. In the code example below, we’ve created a function that uses event.target.id
to show the ID of the clicked button.
<body>
<main>
<button id="button_1" onclick="getClickID()">Button_1</button>
<button id="button_2" onclick="getClickID()">Button_2</button>
<button id="button_3" onclick="getClickID()">Button_3</button>
</main>
<script type="text/javascript">
function getClickID() {
alert(event.target.id);
}
</script>
</body>
Output:
Get Clicked Button ID With the addEventListener
Function in JavaScript
You can implement a custom function that utilizes an event listener to get the ID of an element. This will be the element that fires an event.
Put the custom function in your web page’s <head>
section. This way, it becomes available before the rest of the web page downloads.
In the following code, we’ve used the custom function to add click events to the set of buttons. So, when you run the code in your web browser, you’ll get a JavaScript alert message that shows the button ID.
<head>
<script type="text/javascript">
const customEvent = (documentObject) => {
return {
on: (event_type, css_selector, callback_function) => {
documentObject.addEventListener(event_type, function (event) {
if (event.target.matches(css_selector) === false) return;
callback_function.call(event.target, event);
}, false);
}
}
}
customEvent(document).on('click', '.html-button', function (event) {
alert(event.target.id);
});
</script>
</head>
<body>
<main>
<button id="btn_1" class="html-button">Code-1</button>
<button id="btn_2" class="html-button">Code-2</button>
<button id="btn_3" class="html-button">Code-3</button>
</main>
</body>
Output:
Get Clicked Button With jQuery
This approach is like the first example in this article, but we’ll use jQuery. jQuery provides the click
function that you can attach to an element to get the element’s ID via this.id
.
The code below has buttons that have IDs and class attributes. We use jQuery to grab the button class names, and we attach a click event to all, and when you click any button, you’ll get its ID in an alert
window in your web browser.
<body>
<main>
<button id="btn_one" class="clicked-button">CK-button-1</button>
<button id="btn_two" class="clicked-button">CK-button-2</button>
<button id="btn_three" class="clicked-button">CK-button-3</button>
</main>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"
integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ==" crossorigin="anonymous"
referrerpolicy="no-referrer"
>
</script>
<script>
$('.clicked-button').click(function(){
alert(this.id);
})
</script>
</body>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn