How to Use the %*% Operator in R
- Matrix and Its Dimensions in R
-
Use the
%*%
Operator to Multiply Matrices in R -
Use the
%*%
Operator to Get the Dot Product of Vectors in R - Conclusion
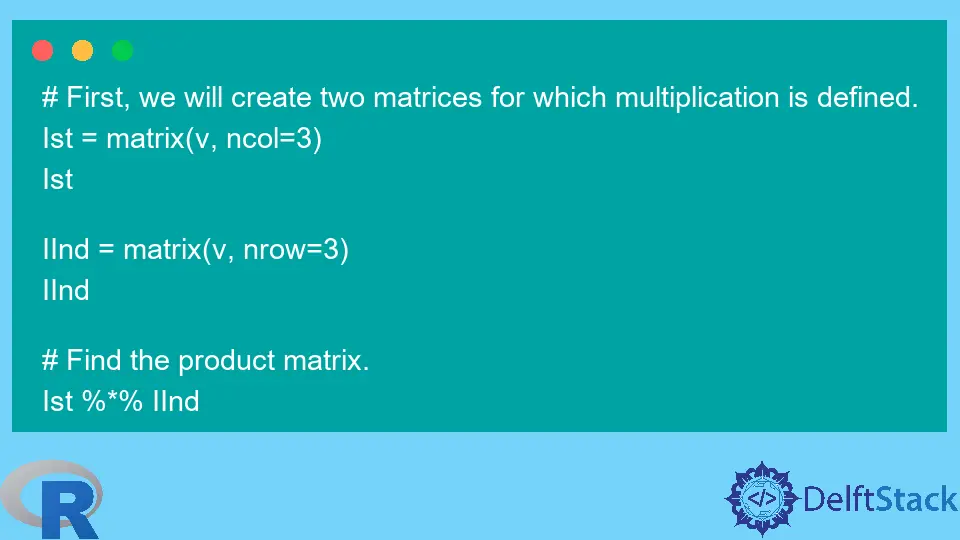
The %*%
operator is used for matrix multiplication. In vectors of the same length, this operator gives the dot product.
In this article, we will explore the use of this operator with some simple examples.
Matrix and Its Dimensions in R
A matrix is a rectangular array of numbers. It is like a table of numbers, having rows and columns.
The following code creates and displays four matrices using the same 12 numbers.
Example Code:
# First, we will create a vector of numbers.
# These 12 numbers are what we will place in our matrices.
v = 7:18
# Matrix with 2 rows and 6 columns.
matrix(v, nrow=2)
dim(matrix(v, nrow=2))
# Matrix with 3 rows and 4 columns.
matrix(v, nrow=3)
dim(matrix(v, nrow=3))
# Matrix with 4 rows and 3 columns.
matrix(v, nrow=4)
dim(matrix(v, nrow=4))
# Matrix with 6 rows and 2 columns.
matrix(v, nrow=6)
dim(matrix(v, nrow=6))
Output:
> # Matrix with 2 rows and 6 columns.
> matrix(v, nrow=2)
[,1] [,2] [,3] [,4] [,5] [,6]
[1,] 7 9 11 13 15 17
[2,] 8 10 12 14 16 18
> dim(matrix(v, nrow=2))
[1] 2 6
> # Matrix with 3 rows and 4 columns.
> matrix(v, nrow=3)
[,1] [,2] [,3] [,4]
[1,] 7 10 13 16
[2,] 8 11 14 17
[3,] 9 12 15 18
> dim(matrix(v, nrow=3))
[1] 3 4
> # Matrix with 4 rows and 3 columns.
> matrix(v, nrow=4)
[,1] [,2] [,3]
[1,] 7 11 15
[2,] 8 12 16
[3,] 9 13 17
[4,] 10 14 18
> dim(matrix(v, nrow=4))
[1] 4 3
> # Matrix with 6 rows and 2 columns.
> matrix(v, nrow=6)
[,1] [,2]
[1,] 7 13
[2,] 8 14
[3,] 9 15
[4,] 10 16
[5,] 11 17
[6,] 12 18
> dim(matrix(v, nrow=6))
[1] 6 2
Each matrix that we created above had a different number of rows and columns.
A matrix is described by the number of its rows and columns; this is called its dimension. A matrix with m
rows and n
columns is referred to as an m x n
matrix, and read as m by n.
The matrices we created had the following dimensions: 2x6
, 3x4
, 4x3
, and 6x2
.
Use the %*%
Operator to Multiply Matrices in R
Matrix multiplication is only defined when the first matrix’s column number equals the second matrix’s number of rows. When this condition is satisfied, we can multiply those two matrices in that order using the %*%
operator, and the product is also a matrix.
The product matrix will have as many rows as the first matrix and as many columns as the second.
Example Code:
# First, we will create two matrices for which multiplication is defined.
Ist = matrix(v, ncol=3)
Ist
IInd = matrix(v, nrow=3)
IInd
# Find the product matrix.
Ist %*% IInd
Output:
> # First, we will create two matrices for which multiplication is defined.
> Ist = matrix(v, ncol=3)
> Ist
[,1] [,2] [,3]
[1,] 7 11 15
[2,] 8 12 16
[3,] 9 13 17
[4,] 10 14 18
> IInd = matrix(v, nrow=3)
> IInd
[,1] [,2] [,3] [,4]
[1,] 7 10 13 16
[2,] 8 11 14 17
[3,] 9 12 15 18
> # Find the product matrix.
> Ist %*% IInd
[,1] [,2] [,3] [,4]
[1,] 272 371 470 569
[2,] 296 404 512 620
[3,] 320 437 554 671
[4,] 344 470 596 722
We will look at another example of valid matrix multiplication and two examples where matrix multiplication is not defined.
Example Code:
# A 3 x 2 matrix.
IInd_b = matrix(20:25, nrow=3)
IInd_b
# A 2 x 6 matrix.
Ist_b = matrix(v, nrow=2)
Ist_b
# Matrix multiplication is defined between Ist and IInd_b.
Ist %*% IInd_b
# Multiplication is NOT defined in the following two cases.
IInd_b %*% Ist
Ist_b %*% IInd_b
Output:
> # A 3 x 2 matrix.
> IInd_b = matrix(20:25, nrow=3)
> IInd_b
[,1] [,2]
[1,] 20 23
[2,] 21 24
[3,] 22 25
> # A 2 x 6 matrix.
> Ist_b = matrix(v, nrow=2)
> Ist_b
[,1] [,2] [,3] [,4] [,5] [,6]
[1,] 7 9 11 13 15 17
[2,] 8 10 12 14 16 18
> # Matrix multiplication is defined between Ist and IInd_b.
> Ist %*% IInd_b
[,1] [,2]
[1,] 701 800
[2,] 764 872
[3,] 827 944
[4,] 890 1016
> # Multiplication is NOT defined in the following two cases.
> IInd_b %*% Ist
Error in IInd_b %*% Ist : non-conformable arguments
> Ist_b %*% IInd_b
Error in Ist_b %*% IInd_b : non-conformable arguments
Use the %*%
Operator to Get the Dot Product of Vectors in R
Vectors are described by their length and class (and type).
Example Code:
# Create a vector.
vtr = c(11,22,33)
# Check that it is a vector.
is.vector(vtr)
# Length of the vector.
length(vtr)
# Class of the vector.
class(vtr)
# Type of the vector.
typeof(vtr)
Output:
> # Create a vector.
> vtr = c(11,22,33)
> # Check that it is a vector.
> is.vector(vtr)
[1] TRUE
> # Length of the vector.
> length(vtr)
[1] 3
> # Class of the vector.
> class(vtr)
[1] "numeric"
> # Type of the vector.
> typeof(vtr)
[1] "double"
The length of a vector is the number of elements (numbers) in it.
When we multiply two vectors of the same length using the %*%
operator, we get the dot product of the vectors. R implicitly treats the first vector as a row-matrix and the second vector as a column-matrix and gives us the product matrix.
It returns a 1x1
matrix rather than a scalar. We can verify this using the is.vector()
and is.matrix()
functions.
In the following code, we will first obtain the dot product between two vectors of the same length. We will then get the same result using matrices of conformable dimensions.
Example Code:
# Four-element vectors.
V_I = 22:25
V_II = 2:5
# Dot product of vectors of the same dimension.
V_I %*% V_II
# Check the input and output.
is.vector(V_I)
is.matrix(V_I)
is.vector(V_I %*% V_II)
is.matrix(V_I %*% V_II)
# Create matrices of conformable dimensions (where matrix multiplication is defined).
m_I = matrix(V_I, nrow=1)
m_I
m_II = matrix(V_II, ncol=1)
m_II
# Matrix product.
m_I %*% m_II
Output:
> # Four-element vectors.
> V_I = 22:25
> V_II = 2:5
> # Dot product of vectors of the same dimension.
> V_I %*% V_II
[,1]
[1,] 334
> # Check the input and output.
> is.vector(V_I)
[1] TRUE
> is.matrix(V_I)
[1] FALSE
> is.vector(V_I %*% V_II)
[1] FALSE
> is.matrix(V_I %*% V_II)
[1] TRUE
> # Create matrices of conformable dimensions (where matrix multiplication is defined).
> m_I = matrix(V_I, nrow=1)
> m_I
[,1] [,2] [,3] [,4]
[1,] 22 23 24 25
> m_II = matrix(V_II, ncol=1)
> m_II
[,1]
[1,] 2
[2,] 3
[3,] 4
[4,] 5
> # Matrix product.
> m_I %*% m_II
[,1]
[1,] 334
We cannot compute the dot product if the vectors are of different lengths.
Example Code:
# A three-element vector.
V_II_b = 6:8
# Dot product is not possible.
V_I %*% V_II_b
Output:
> # A three-element vector.
> V_II_b = 6:8
> # Dot product is not possible.
> V_I %*% V_II_b
Error in V_I %*% V_II_b : non-conformable arguments
Conclusion
For conformable matrices for multiplication, %*%
returns the product matrix. For vectors of the same length, it returns the dot product as a 1x1
matrix.