How to Create Empty Matrix in R
- Create an Empty Matrix Using Rows in R
- Create Empty Matrix Using Columns in R
- Create an Empty Matrix Using Both Rows and Columns in R
-
Create Empty Matrix Using the
array()
Function in R - Conclusion
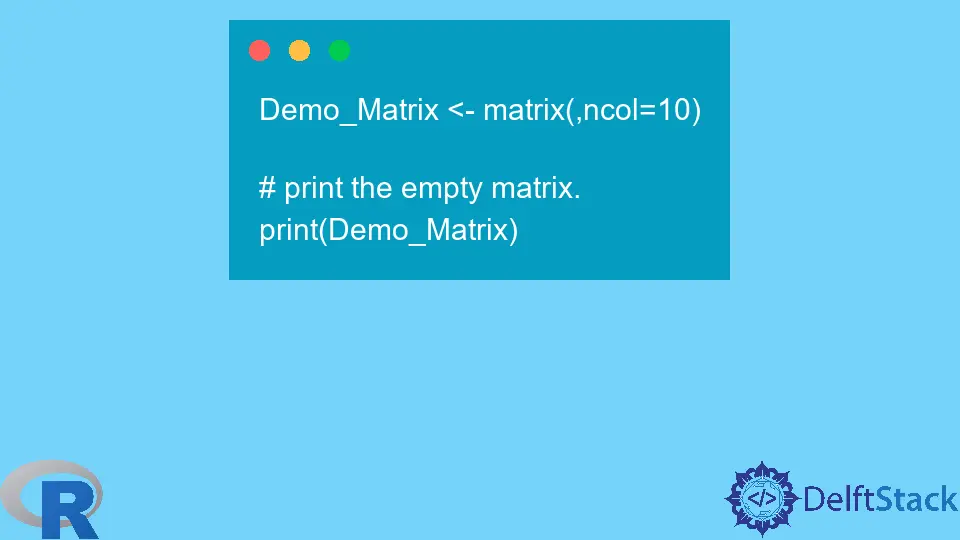
A matrix in R is a two-dimensional data structure with rows and columns. An empty matrix is created the same way we create a regular matrix.
Creating an empty matrix in R involves initializing a matrix with no values or populating it with placeholders. This tutorial demonstrates different ways of creating an empty matrix in R.
Create an Empty Matrix Using Rows in R
Creating an empty matrix using rows in R involves initializing a matrix structure with no values, where the number of rows is specified, and the number of columns may or may not be specified. The matrix is typically populated with placeholders or initialized with default values, such as NA
(Not Available).
Basic Syntax:
matrix(nrow = num_rows, ncol = num_cols)
In the syntax, the nrow
specifies the number of rows in the matrix. The ncol
specifies the number of columns in the matrix.
If the number of rows/columns is not provided, R assumes one row/column by default.
In the following example, we’ll create an empty matrix bypassing rows as an argument in the matrix.
Code:
Demo_Matrix <- matrix(, nrow = 10)
print(Demo_Matrix)
In the code, we are creating an empty matrix named Demo_Matrix
with 10 rows and an unspecified number of columns using the matrix()
function and leaving the matrix elements empty. The argument nrow=10
specifies that the matrix should have 10 rows.
Since we don’t specify the number of columns, R assumes a default of one column.
Output:
[,1]
[1,] NA
[2,] NA
[3,] NA
[4,] NA
[5,] NA
[6,] NA
[7,] NA
[8,] NA
[9,] NA
[10,] NA
The output shows a matrix with 10 rows and 1 column, filled with NA
(Not Available) placeholders since we created an empty matrix. This matrix serves as a placeholder that can later be filled with actual data as needed.
Create Empty Matrix Using Columns in R
Creating an empty matrix using columns in R is the same as above, where it involves initializing a matrix structure with no values, where the number of columns is specified, and the number of rows may or may not be specified.
Code:
Demo_Matrix <- matrix(, ncol = 3)
print(Demo_Matrix)
In the code, we are creating an empty matrix named Demo_Matrix
in R with 3 columns and an unspecified number of rows using the matrix()
function. The argument ncol=3
specifies that the matrix should have 3 columns.
Since we don’t specify the number of rows, R assumes a default of one row.
Output:
[,1] [,2] [,3]
[1,] NA NA NA
The output shows a one-row matrix with 3 columns, and all elements are initialized as NA
(Not Available) since we created an empty matrix.
Create an Empty Matrix Using Both Rows and Columns in R
We can also create an empty matrix with both rows and columns, bypassing both rows and columns as an argument to the matrix.
Code:
Demo_Matrix <- matrix(, nrow = 4, ncol = 4)
print(Demo_Matrix)
In the code, we are creating an empty matrix named Demo_Matrix
in R with dimensions of 4 rows and 4 columns using the matrix()
function. The arguments nrow = 4
and ncol = 4
specifies the number of rows and columns for the matrix.
Since we leave the matrix elements empty, they are initialized as NA
(Not Available).
Output:
[,1] [,2] [,3] [,4]
[1,] NA NA NA NA
[2,] NA NA NA NA
[3,] NA NA NA NA
[4,] NA NA NA NA
The output displays the contents of the Demo_Matrix
, which is a 4x4 matrix created with the matrix()
function in R. Each element in the matrix is initialized as NA
(Not Available) since we didn’t provide specific values during creation.
Create Empty Matrix Using the array()
Function in R
Creating an empty matrix using the array()
function in R involves initializing a multi-dimensional array with no values, specifying the dimensions, and, in this case, defining it as a two-dimensional matrix. The array()
function is flexible and can be adapted for various dimensions, allowing for the creation of empty matrices.
Basic Syntax:
empty_matrix <- array(, dim = c(nrow, ncol))
In the syntax, the array()
is the function that initializes the array structure, and the empty values are denoted by the comma inside the parentheses. The dim
parameter is set to a vector c(nrow, ncol)
representing the desired dimensions of the matrix, where the number of rows is nrow
and the number of columns is ncol
.
Code:
empty_matrix <- array(, dim = c(5, 5))
print(empty_matrix)
In this code, we create an empty matrix named empty_matrix
with dimensions specified as 5 rows and 5 columns. The array()
function initializes the array structure with empty values denoted by the comma inside the parentheses.
The dim
parameter is set to a vector c(5, 5)
to determine the desired dimensions of the matrix.
Output:
[,1] [,2] [,3] [,4] [,5]
[1,] NA NA NA NA NA
[2,] NA NA NA NA NA
[3,] NA NA NA NA NA
[4,] NA NA NA NA NA
[5,] NA NA NA NA NA
The output shows a 5x5 matrix filled with NA
(Not Available) values, indicating an empty matrix with the specified dimensions.
Conclusion
In conclusion, creating an empty matrix in R is crucial for various applications, allowing us to initialize a matrix structure without specific values. We explored methods using the matrix()
function to create empty matrices with specified rows, columns, or both, resulting in flexible structures filled with NA
placeholders.
Additionally, we demonstrated the array()
function’s versatility in initializing a two-dimensional empty matrix. These methods offer adaptability and flexibility for working with matrices in R, providing placeholders ready for future data.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook