How to Make Tkinter Text Widget Read Only
-
Set the
Text
State todisable
to Make TkinterText
Read Only -
Bind Any Key Press to the
break
Function to Make TkinterText
Read Only
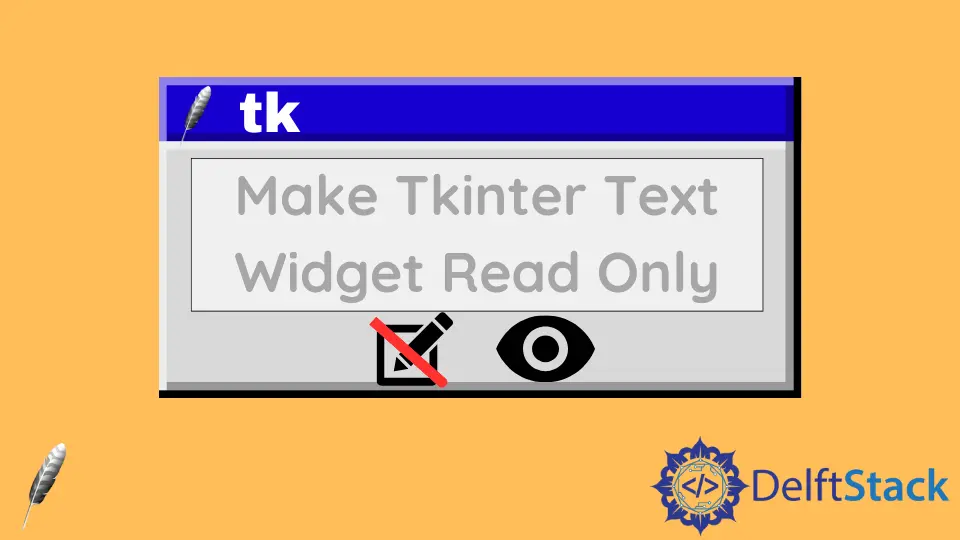
We will introduce methods to make the Tkinter Text widget read-only,
- Set the
Text
state to bedisable
- Bind any key press to the
break
function
Set the Text
State to disable
to Make Tkinter Text
Read Only
The Text
widget becomes read-only after its state is set to be disable
.
import tkinter as tk
root = tk.Tk()
readOnlyText = tk.Text(root)
readOnlyText.insert(1.0, "ABCDEF")
readOnlyText.configure(state="disabled")
readOnlyText.pack()
root.mainloop()
The default state of a Text
widget is NORMAL
, that means the user could edit, append, insert or edit text content in it.
readOnlyText.configure(state="disabled")
You need to change the Text
widget state to DISABLED
to make it read-only. Any attempt to change the text inside that widget will be silently ignored.
disabled
to normal
if you intend to update the Text
widget content, otherwise, it keeps read-only.Bind Any Key Press to the break
Function to Make Tkinter Text
Read Only
If we bind any key stroke to the function that only return break
to the Text
widget, we could get the same result that the Text
becomes read-only.
import tkinter as tk
root = tk.Tk()
readOnlyText = tk.Text(root)
readOnlyText.insert(1.0, "ABCDEF")
readOnlyText.bind("<Key>", lambda a: "break")
readOnlyText.pack()
root.mainloop()
The difference between this solution and the above solution is that the CTRL+C doesn’t work here. It means you could neither edit the content nor copy it.
We need to make the exception of CTRL+C to the function binding to the Text
if CTRL+C is desired.
import tkinter as tk
def ctrlEvent(event):
if 12 == event.state and event.keysym == "c":
return
else:
return "break"
root = tk.Tk()
readOnlyText = tk.Text(root)
readOnlyText.insert(1.0, "ABCDEF")
readOnlyText.bind("<Key>", lambda e: ctrlEvent(e))
readOnlyText.pack()
root.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook