How to Convert Column Values to String in Pandas
Suraj Joshi
Feb 02, 2024
-
Convert the Data Type of Column Values of a DataFrame to String Using the
apply()
Method -
Convert the Data Type of All DataFrame Columns to
string
Using theapplymap()
Method -
Convert the Data Type of Column Values of a DataFrame to
string
Using theastype()
Method
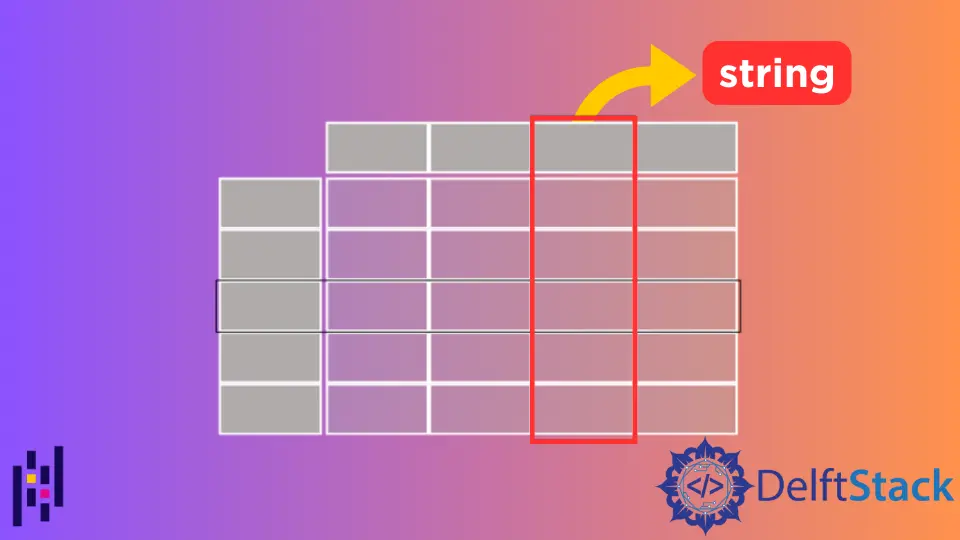
This tutorial explains how we can convert the data type of column values of a DataFrame to the string.
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print(employees_df)
Output:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
We will use the DataFrame displayed in the above example to explain how we can convert the data type of column values of a DataFrame to the string.
Convert the Data Type of Column Values of a DataFrame to String Using the apply()
Method
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Age"] = employees_df["Age"].apply(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
Output:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score int64
Age object
dtype: object
It changes the data type of the Age
column from int64
to object
type representing the string
.
Convert the Data Type of All DataFrame Columns to string
Using the applymap()
Method
If we want to change the data type of all column values in the DataFrame to the string
type, we can use the applymap()
method.
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df = employees_df.applymap(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
Output:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
zeppy@zeppy-G7-7588:~/test/Week-01/taddaa$ python3 1.py
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age object
dtype: object
It converts the datatype of all DataFrame columns to the string
type denoted by object
in the output.
Convert the Data Type of Column Values of a DataFrame to string
Using the astype()
Method
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Score"] = employees_df["Score"].astype(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
Output:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age int64
dtype: object
It converts the data type of the Score
column in the employees_df
Dataframe to the string
type.
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn