How to Convert DataFrame Column to String in Pandas
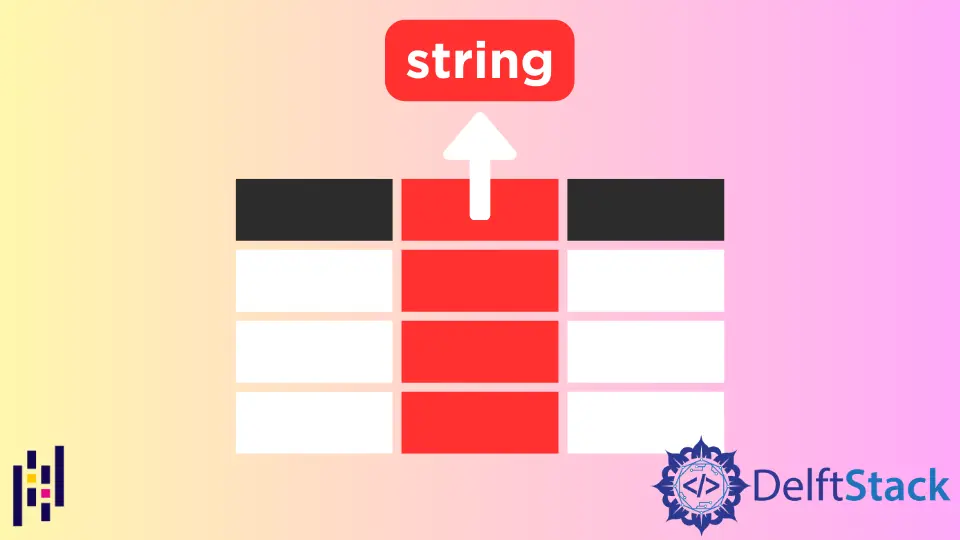
We will introduce methods to convert Pandas DataFrame column to string
.
- Pandas DataFrame Series
astype(str)
method - DataFrame
apply
method to operate on elements in column
We will use the same DataFrame below in this article.
import pandas as pd
df = pd.DataFrame({"A": [1, 2, 3], "B": [4.1, 5.2, 6.3], "C": ["7", "8", "9"]})
print(df)
print(df.dtypes)
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
A int64
B float64
C object
dtype: object
Pandas DataFrame Series astype(str)
Method
Pandas Series astype(dtype)
method converts the Pandas Series to the specified dtype
type.
pandas.Series.astype(str)
It converts the Series, DataFrame column as in this article, to string
.
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df['A'] = df['A'].astype(str)
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B float64
C object
dtype: object
astype()
method doesn’t modify the DataFrame
data in-place, therefore we need to assign the returned Pandas Series
to the specific DataFrame
column.
We could also convert multiple columns to string simultaneously by putting columns’ names in the square brackets to form a list.
>>> df[['A','B']] = df[['A','B']].astype(str)
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B object
C object
dtype: object
DataFrame apply
Method to Operate on Elements in Column
apply(func, *args, **kwds)
apply
method of DataFrame
applies the function func
to each column or row.
We could use lambda
function in the place of func
for simplicity.
>>> df['A'] = df['A'].apply(lambda _: str(_))
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B float64
C object
dtype: object
You couldn’t use apply
method to apply the function to multiple columns.
>>> df[['A','B']] = df[['A','B']].apply(lambda _: str(_))
Traceback (most recent call last):
File "<pyshell#31>", line 1, in <module>
df[['A','B']] = df[['A','B']].apply(lambda _: str(_))
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\frame.py", line 3116, in __setitem__
self._setitem_array(key, value)
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\frame.py", line 3144, in _setitem_array
self.loc._setitem_with_indexer((slice(None), indexer), value)
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\indexing.py", line 606, in _setitem_with_indexer
raise ValueError('Must have equal len keys and value '
ValueError: Must have equal len keys and value when setting with an iterable
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum