How to Remove the Last Character From a String in PHP
-
Use the
rtrim()
Function to Remove the Last Character -
Use the
substr()
Function to Remove the Last Character
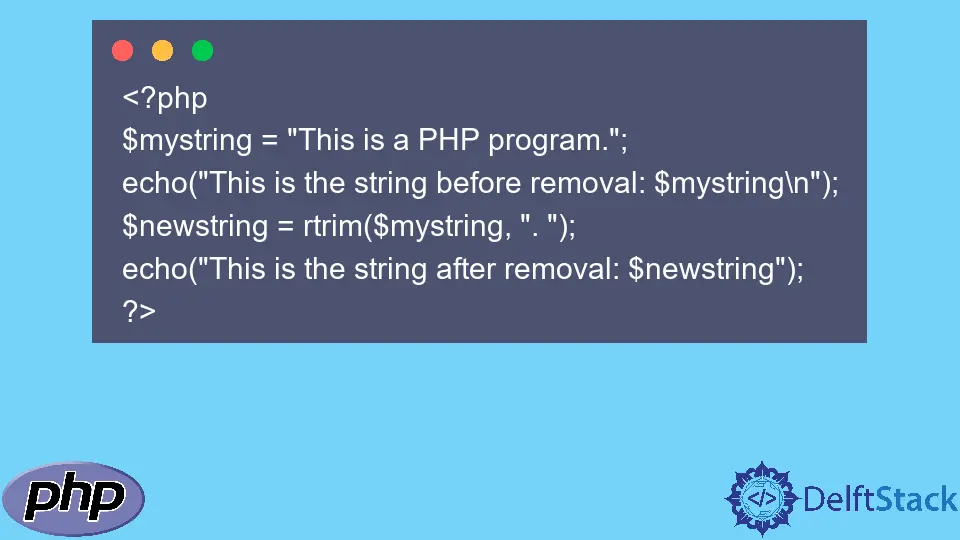
This article will introduce different methods to remove the last character from a string in PHP with the use of functions rtrim()
and substr()
.
Use the rtrim()
Function to Remove the Last Character
The rtrim()
function is used explicitly for removing white spaces or characters from the end of a string. The correct syntax to use this function is as follows:
rtrim($string, $character);
The built-in function rtrim()
has two parameters; the details of each are as follows:
Parameters | Description | |
---|---|---|
$string |
mandatory | It’s the string from which we’re removing the last character. |
$character |
optional | It’s the character that we want to remove from the end. |
This function returns the final string obtained after performing the removal. The program below shows how we can use the rtrim()
function to remove the last character from a string.
<?php
$mystring = "This is a PHP program.";
echo("This is the string before removal: $mystring\n");
$newstring = rtrim($mystring, ". ");
echo("This is the string after removal: $newstring");
?>
Output:
This is the string before removal: This is a PHP program.
This is the string after removal: This is a PHP program
Use the substr()
Function to Remove the Last Character
In PHP, we can also use the substr()
function to remove the last character from a string. This command returns a specified substring, and the correct syntax to execute it is as follows:
substr($string, $start, $length)
The function substr()
accepts three parameters:
Parameters | Description | |
---|---|---|
$string |
mandatory | It’s the string from which we’re removing the last character. |
$start |
mandatory | It’s the start position of a substring. You can read more about it here. |
$length |
optional | If the value is given, then the function will return the substring from the $start parameter to the specified length. |
The program that removes the last character from the string using the substr()
function is as follows:
<?php
$mystring = "This is a PHP program.";
echo substr($mystring, 0, -1);
?>
Output:
This is a PHP program