How to Convert a String to a Number in PHP
- Use Explicit Type Casting to Convert a String to a Number in PHP
-
Use
intval()
Function to Convert a String to a Number in PHP -
Use
settype()
Function to Convert a String to a Number in PHP
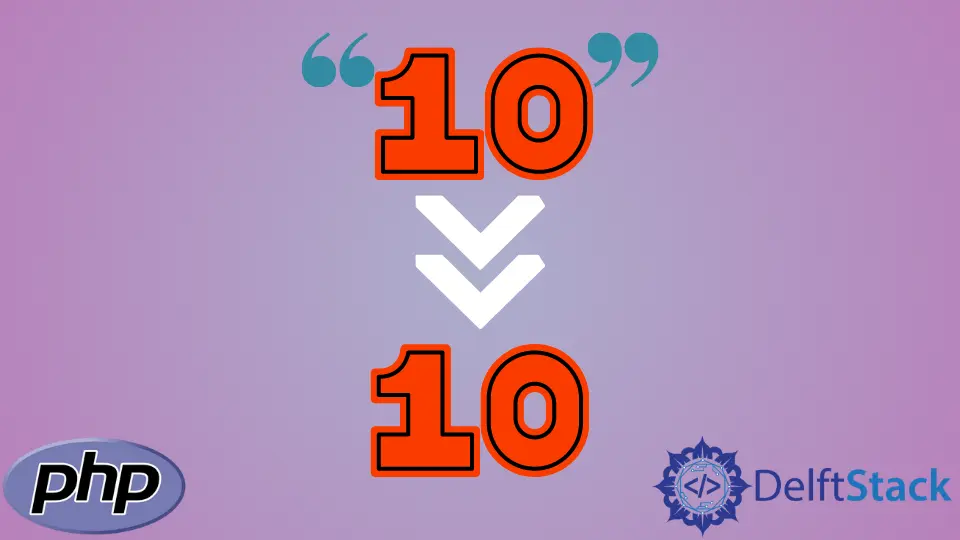
In this article, we will introduce methods to convert a string to a number in PHP.
- Using explicit type casting
- Using
intval()
/floatval()
function - Using
settype()
function
Use Explicit Type Casting to Convert a String to a Number in PHP
Explicit type casting converts a variable of any type to a variable of the required type. It converts a variable to primitive data types. The correct syntax to explicitly typecast a variable to an int
or a float
is as follows
$variableName = (int)$stringName
$variableName = (float)$stringName
The variable $variableName
is the integer variable to hold the value of the number. The variable $stringName
is the numeric string
. We can also convert non-numeric strings to a number.
<?php
$variable = "10";
$integer = (int)$variable;
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "8.1";
$float = (float)$variable;
echo "The variable $variable has converted to a number and its value is $float.";
?>
Output:
The variable 10 has converted to a number and its value is 10.
The variable 8.1 has converted to a number and its value is 8.1.
For non-numeric string, we will get a 0 as the output.
<?php
$variable = "abc";
$integer = (int)$variable;
echo "The variable has converted to a number and its value is $integer.";
?>
Output:
The variable has converted to a number and its value is 0.
Use intval()
Function to Convert a String to a Number in PHP
The intval()
function converts a string
to an int
.
The floatval()
function converts a string
to a float
.
The parameter that those functions accept is a string
and the return value is an integer or a floating number respectively.
intval( $StringName );
The variable StringName
holds the value of the string
.
<?php
$variable = "53";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Output:
The variable 53 has converted to a number and its value is 53.
The variable 25.3 has converted to a number and its value is 25.3.
It the given string is a mixture of the number and string representation, like 2020Time
, intval()
will return 2020
. But if the given string doesn’t start with a number then it returns 0
. So is the floatval()
method.
Example Codes:
<?php
$variable = "2020Time";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "Time2020";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3Time";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
echo "\n";
$variable = "Time25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Output:
The variable 2020Time has converted to a number and its value is 2020.
The variable Time2020 has converted to a number and its value is 0.
The variable 25.3Time has converted to a number and its value is 25.3.
The variable Time25.3 has converted to a number and its value is 0.
Use settype()
Function to Convert a String to a Number in PHP
The settype()
function converts the variable of any datatype to a particular datatype. It accepts two parameters, the variable and the type name.
settype($variableName, "typeName");
The $variableName
is the variable of any datatype that we want to convert to a number. The "typeName"
contains the name of the datatype that we want for our $variableName
variable.
<?php
$variable = "45";
settype($variable, "integer");
echo "The variable has converted to a number and its value is $variable.";
?>
The settype()
function has set the type of $variable
from string
to integer. It is only suitable for primitive datatypes.
Output:
The variable has converted to a number and its value is 45.
Related Article - PHP String
- How to Remove All Spaces Out of a String in PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert an Integer Into a String in PHP
- How to Convert an Array to a String in PHP