How to Split a String With Delimiters in Go
Jay Singh
Feb 02, 2024
-
Split a String With Delimiters Using the
Split()
Method in Go -
Split a String With Delimiters Using the
SplitAfter()
Method in Go
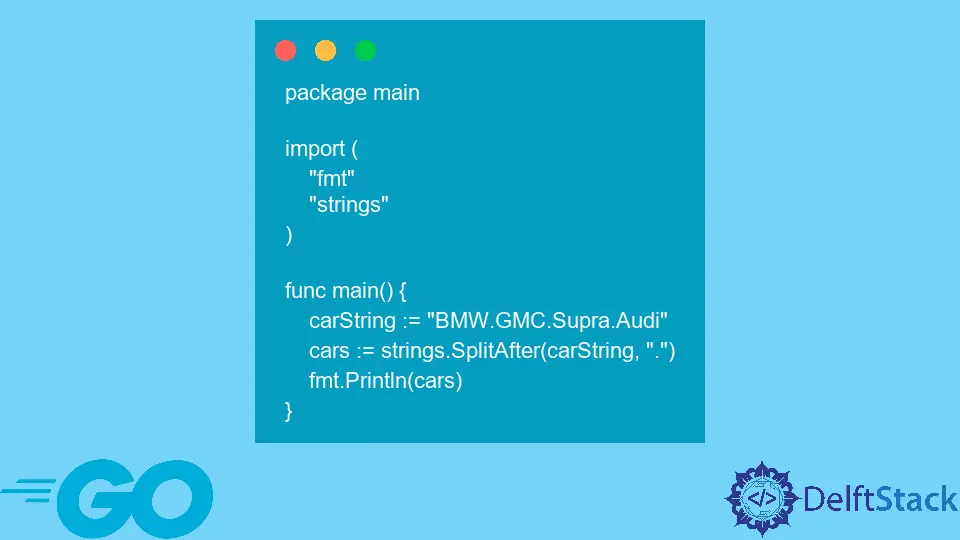
This article will provide methods to divide strings in Go.
Split a String With Delimiters Using the Split()
Method in Go
In Go, the Split()
function (included in the strings package) divides a string into a list of substrings using a separator. The substrings are returned in the form of a slice.
In the following example, we’ll use a string with values separated by commas as delimiters.
Example 1:
package main
import (
"fmt"
"strings"
)
func main() {
var str = "a-b-c"
var delimiter = "-"
var parts = strings.Split(str, delimiter)
fmt.Println(parts)
}
Output:
[a b c]
Example 2:
package main
import (
"fmt"
"strings"
)
func main() {
str := "hi, there!, Good morning"
split := strings.Split(str, ",")
fmt.Println(split)
fmt.Println("Length of the slice:", len(split))
}
Output:
[hi there! Good morning]
Length of the slice: 3
Example 3:
package main
import (
"fmt"
"strings"
)
func main() {
carString := "BMW,GMC,Supra,Audi"
cars := strings.Split(carString, ",")
fmt.Println(cars)
}
Output:
[BMW GMC Supra Audi]
Split a String With Delimiters Using the SplitAfter()
Method in Go
SplitAfter()
separates the original text but leaves the delimiters at the end of each substring, similar to Split()
.
package main
import (
"fmt"
"strings"
)
func main() {
carString := "BMW.GMC.Supra.Audi"
cars := strings.SplitAfter(carString, ".")
fmt.Println(cars)
}
Output:
[BMW. GMC. Supra. Audi]