How to Convert String to Integer Type in Go
-
Atoi()
Function Fromstrconv
Package -
ParseInt()
Function Fromstrconv
Package -
fmt.Sscanf
Method - Comparison of Methods
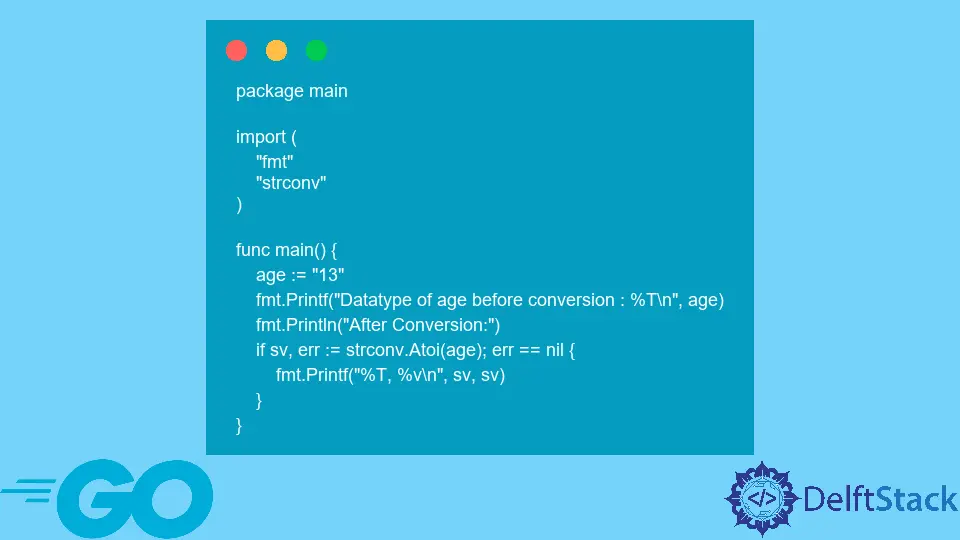
Go is the statically typed language meaning if we define the variable as int
, it can only be int
; we can’t assign the string
to it without converting the string data type of a variable. Go has an inbuilt package called strconv
that provides three functions Atoi()
, Sscanf()
, and ParseInt()
to convert Go string to int.
Atoi()
Function From strconv
Package
Package strconv
implements conversions to and from string representations of basic data types. To convert a string into an integer number, we use the Atoi()
function from strconv
package. Atoi
stands for ASCII to integer
.
package main
import (
"fmt"
"strconv"
)
func main() {
age := "13"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
if sv, err := strconv.Atoi(age); err == nil {
fmt.Printf("%T, %v\n", sv, sv)
}
}
Output:
Datatype of age before conversion : string
After Conversion:
int, 13
If the input string isn’t in the integer format, then the function returns zero.
package main
import (
"fmt"
"strconv"
)
func main() {
age := "abc"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
sv, _ := strconv.Atoi(age)
fmt.Printf("%T, %v\n", sv, sv)
}
Output:
Datatype of age before conversion : string
After Conversion:
int, 0
ParseInt()
Function From strconv
Package
strconv.ParseInt(s, base, bitSize)
method interprets the input string s
in the given base
(0, 2 to 36) and bit size (0 to 64) and returns a corresponding integer. Atoi(s)
is equivalent to ParseInt(s, 10, 0)
.
package main
import (
"fmt"
"reflect"
"strconv"
)
func main() {
x := "123"
fmt.Println("Value of x before conversion: ", x)
fmt.Println("Datatype of x before conversion:", reflect.TypeOf(x))
intStr, _ := strconv.ParseInt(x, 10, 64)
fmt.Println("Value after conversion: ", intStr)
fmt.Println("Datatype after conversion:", reflect.TypeOf(intStr))
}
Output:
Value of x before conversion: 123
Datatype of x before conversion: string
Value after conversion: 123
Datatype after conversion: int64
fmt.Sscanf
Method
fmt.Sscanf()
is great for parsing custom strings holding a number. Sscanf()
function scans the argument string, storing successive space-separated values into successive arguments as defined by the format and returns the number of items successfully parsed.
package main
import (
"fmt"
)
func main() {
s := "id:00234"
var i int
if _, err := fmt.Sscanf(s, "id:%5d", &i); err == nil {
fmt.Println(i)
}
}
Output:
234
Comparison of Methods
The strconv.ParseInt()
is the fastest of all methods, strconv.Atoi()
is a bit slower than strconv.ParseInt()
and fmt.Sscanf()
is slowest of all but most flexible method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn