C# Parse JSON in C#
-
C# Program to Parse
JSON string
UsingJsonConvert.DeserializeObject()
Method -
C# Program to Parse
JSON
String UsingJObject.Parse()
Method -
C# Program to Parse
JSON
String UsingJavaScriptSerializer().Deserialize()
Method
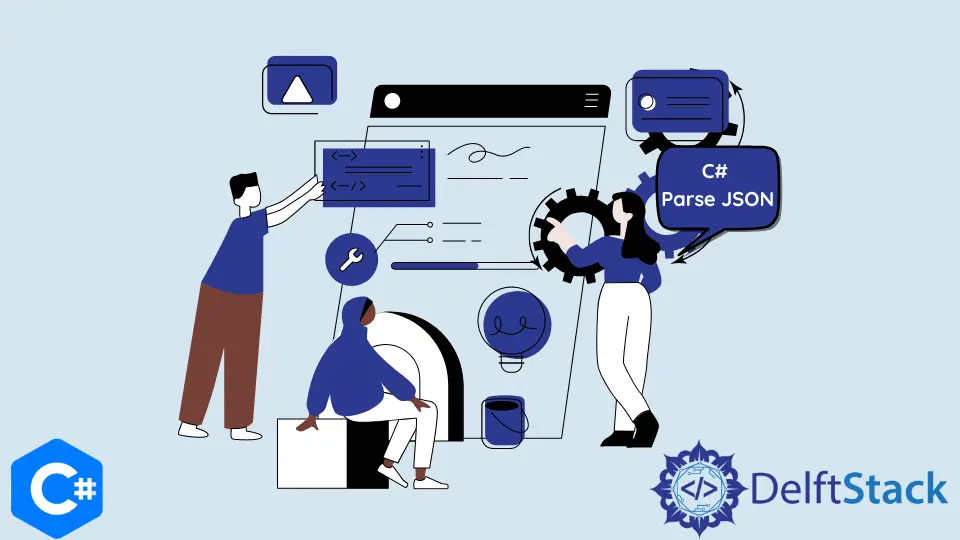
JavaScript Object Notation (JSON
) is a format for exchanging data. It is easier to write for humans and simpler to process for machines. In C#, there are many methods to process JSON
data.
In this article, we are going to discuss the methods by which you can parse JSON
into a C# object.
C# Program to Parse JSON string
Using JsonConvert.DeserializeObject()
Method
The method JsonConvert.DeserializeObject()
belongs to the JsonConvert
class. It is used to convert a JSON
string to a C# object. The object is of a custom class that is created by analyzing the JSON
string.
The correct syntax to use this method is as follows:
JsonConvert.DeserializeObject<CustomClassName>(JsonStringName);
Example Code:
using System;
using Newtonsoft.Json;
namespace JSONParsing {
public class Parsing {
public static void Main(string[] args) {
var jsonString = @"{'FirstName': 'Olivia', 'LastName': 'Mason'}";
// Use of the method
var NameObject = JsonConvert.DeserializeObject<Name>(jsonString);
Console.WriteLine(string.Concat("The First Name and Last Name are, ", NameObject.FirstName,
" " + NameObject.LastName, "."));
}
// Creating custom class after analyzing JSON string
public class Name {
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
}
Output:
The First Name and Last Name are: Olivia Mason.
This method throws JsonSerializationException
if the conversion from JSON
to C# object is not successful. This exception is then handled by using a try-catch
block.
C# Program to Parse JSON
String Using JObject.Parse()
Method
The method JObject.Parse()
is a JObject
class method. This parse method is used to parse a JSON
string into a C# object. It parses the data of string based on its key
value. This key value
is then used to retrieve the data.
The correct syntax to use this method is as follows:
Jobject.Parse(jsonStringName);
Example Code:
using System;
using Newtonsoft.Json.Linq;
namespace JSONParsing {
public class Parsing {
public static void Main(string[] args) {
string jsonString =
@"{
'FirstName':'Olivia',
'LastName':'Mason'
}";
// Use of the method
var Name = JObject.Parse(jsonString);
Console.WriteLine(string.Concat("The First Name and Last Name is: ", Name["FirstName"],
" " + Name["LastName"], "."));
}
}
}
Output:
The First Name and Last Name is: Olivia Mason.
JObject.Parse()
method throws exceptions that could be handled by using a try-catch
block.
C# Program to Parse JSON
String Using JavaScriptSerializer().Deserialize()
Method
This method can be implemented in the later versions of .NET
. For earlier versions, the above two methods are the best. This method is used to convert a JSON
string to a C# object.
The correct syntax to use this method is as follows:
JavaScriptSerializer().Deserialize<CustomClassName>(jsonString);
Example Code:
using System;
using System.Web.Script.Serialization;
class Parsing {
static void Main() {
var json = @"{""name"":""Olivia Mason"",""age"":19}";
var ObjectName = new JavaScriptSerializer().Deserialize<MyInfo>(json);
Console.WriteLine("The name is:", ObjectName.name, ".");
}
}
class MyInfo {
public string name { get; set; }
public int age { get; set; }
}
Output:
The name is: Olivia Mason.