How to Convert the Object to a JSON String in C#
-
C# Program to Convert an Object to
JSON
String UsingJavaScriptSerializer().Serialize()
Method -
C# Program to Convert an Object to a
JSON
String UsingJsonConvert.SerializeObject()
Method -
C# Program to Convert an Object to
JSON
String UsingJObject.FromObject()
Method
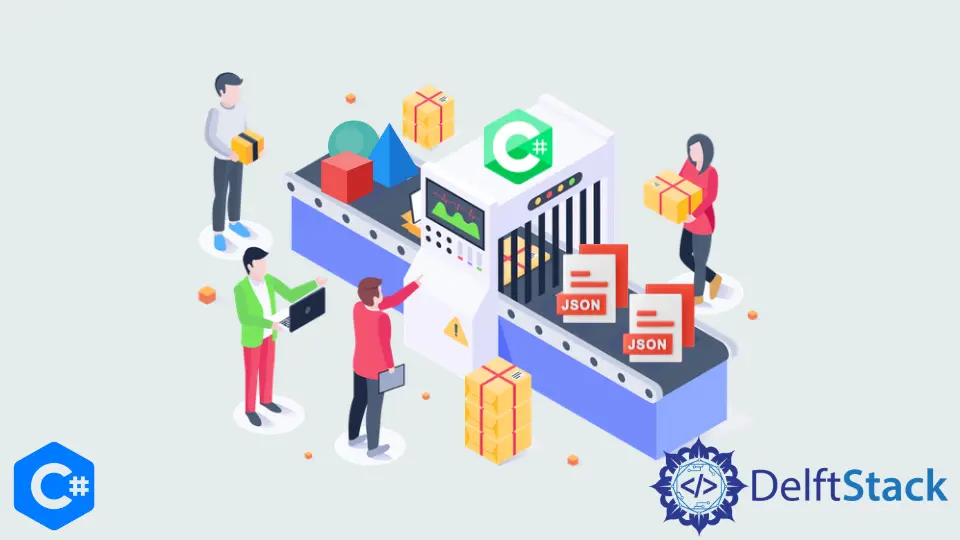
In C#, information can be converted into a useful format called JavaScript Object Notation (JSON
). It is converted because the JSON
format is simpler to understand and easier to process.
This article focuses on the methods to convert a C# object into a JSON
string.
C# Program to Convert an Object to JSON
String Using JavaScriptSerializer().Serialize()
Method
To implement this method, we first create a custom class object that contains the information. This object is then passed as a parameter to JavaScriptSerializer().Serialize()
method. As a result, we get our information converted to a JSON
string.
The correct syntax to use this method is as follows:
var jsonStringName = new JavaScriptSerializer();
var jsonStringResult = jsonStringName.Serialize(ObjectName);
Example Code:
using System;
using System.Web.Script.Serialization;
class Conversion {
static void Main() {
// Creating Custom Class Object
var Object =
new MyInformation { firstName = "Olivia", lastName = "Mason",
dateOfBirth = new DateOfBirth { year = 1999, month = 06, day = 19 } };
// Creating a JavaScriptSerializer Object
var jsonString = new JavaScriptSerializer();
// Use of Serialize() method
var jsonStringResult = jsonString.Serialize(Object);
Console.WriteLine(jsonStringResult);
}
}
// Custom Classes
public class MyInformation {
public string firstName;
public string lastName;
public DateOfBirth dateOfBirth;
}
public class DateOfBirth {
public int year;
public int month;
public int day;
}
Output:
{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}
C# Program to Convert an Object to a JSON
String Using JsonConvert.SerializeObject()
Method
JsonConvert.SerializeObject()
is a JsonConvert
class method. It is used to convert a C# object into a JSON
string. The object is passed as a parameter to this method.
The correct syntax to use this method is as follows:
JsonConvert.SerializeObject(ObjectName);
Example Code:
using System;
using Newtonsoft.Json;
namespace JSONConversion {
public class Conversion {
public static void Main(string[] args) {
// Creating custom class object
Name NewName = new Name { FirstName = "Olivia", LastName = "Mason" };
// Use of JsonConvert.SerializeObject()
string jsonString = JsonConvert.SerializeObject(NewName);
Console.WriteLine(jsonString);
}
// Creating custom class
public class Name {
public string FirstName;
public string LastName;
}
}
}
Output:
{
'FirstName': 'Olivia',
'LastName': 'Mason'
}
C# Program to Convert an Object to JSON
String Using JObject.FromObject()
Method
This method can be implemented in the earlier versions of .Net
. It easily converts a C# object to a JSON
string. The object name is passed as a parameter to this method.
The correct syntax to use this method is as follows:
JObject.FromObject(ObjectName);
Example Code:
using System;
using Newtonsoft.Json.Linq;
namespace JSONConversion {
public class Conversion {
public static void Main(string[] args) {
name Name = new name { firstname = "Olivia", lastname = "Mason" };
// Use of the method
var json = JObject.FromObject(Name);
Console.WriteLine(json);
}
public class name {
public string firstname;
public string lastname;
}
}
}
Output:
{
"firstname": "Olivia",
"lastname": "Mason"
}