How to Convert Char* to Int in C
-
How to Convert
char*
toint
in C Using thestrtol
Function -
How to Convert
char*
toint
in C Using theatoi
Function - Conclusion
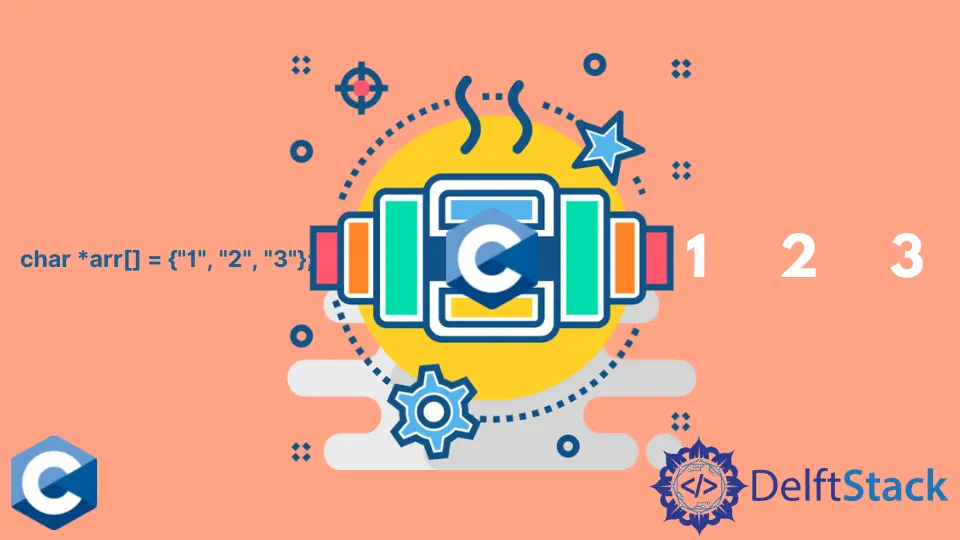
Converting a char*
(a pointer to a string of characters) to an int
in C is a common task in programming, often encountered when dealing with user input, file parsing, or data manipulation. While C provides several methods for this conversion, each with its features and considerations, understanding the most appropriate approach for a given scenario is crucial for writing efficient and reliable code.
In this article, we will explore the process of converting char*
strings to integers in C, focusing on two primary methods: using the strtol
function and the atoi
function.
How to Convert char*
to int
in C Using the strtol
Function
Converting a char*
(a pointer to a string of characters) to an int
in C can be efficiently achieved using the strtol
function. This function is part of the C standard library and provides a robust way to convert strings to long integer values.
The syntax of the strtol
function is as follows:
long strtol(const char *str, char **endptr, int base);
Where:
str
: A pointer to the initial string to be converted.endptr
: A pointer to achar*
where the function stores the address of the first character after the number.base
: The base of the numerical value. It can be between 2 and 36.
The strtol
function parses the initial portion of the string pointed to by str
as an integer.
It stops when it encounters the first character that is not a valid part of the number in the given base. It returns the result as a long integer value.
Example 1: Convert char*
Array to int
Using strtol
Let’s delve into a code example demonstrating how to convert elements from a char*
array to integers and display them on the console.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char *arr[] = {"12", "33", "43", "23", "90"};
size_t len = sizeof(arr) / sizeof(arr[0]);
long int arr3[len];
for (int i = 0; i < len; ++i) {
arr3[i] = strtol(arr[i], NULL, 10);
}
for (size_t i = 0; i < len; i++) {
printf("%4ld | ", arr3[i]);
}
printf("\n");
return 0;
}
In this example, we start by initializing an array of char*
strings called arr
, which contains numeric values represented as strings. We determine the length of this array using the sizeof
operator and division by the size of its first element, which gives us the number of elements.
We then declare an array arr3
of long integers to store the converted values.
Now, we enter a for
loop to iterate over each element of the arr
array. Within this loop, we call the strtol
function for each string element of arr
.
This function converts the string to a long integer, interpreting the string as a base-10 number. We assign the converted value to the corresponding index in the arr3
array.
Finally, we loop over the arr3
array and print each converted integer to the console.
Code Output:
Example 2: Convert Program Arguments to int
Using strtol
Alternatively, program arguments can serve as input strings for conversion into integer types. To access and process program arguments, int argc, char* argv[]
parameters must be utilized within the main
function.
The following code snippet verifies if the user provided at least a single argument to the program; otherwise, it exits, displaying the corresponding error message.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: ./%s int1 int2 int3...\n", argv[0]);
exit(EXIT_FAILURE);
}
long int arr2[argc - 1];
for (int i = 0; i < argc - 1; ++i) {
arr2[i] = strtol(argv[i + 1], NULL, 10);
}
for (int i = 0; i < argc - 1; ++i) {
printf("%4ld | ", arr2[i]);
}
printf("\n");
return 0;
}
In this example, we first check if the user provided at least one argument to the program. If not, we print a usage message and exit with a failure status.
Assuming the user provided arguments, we initialize an array arr2
of long integers to store the converted values. We then enter a for
loop to iterate over each argument passed to the program.
Starting from the second argument (index 1), we call strtol
to convert each argument to a long integer, interpreting it as a base-10 number.
After converting all arguments, we loop over the arr2
array and print each converted integer to the console.
Code Output:
Example 3: Properly Verify strtol
Function’s Results for char*
to int
Conversion
In this example, we will enhance our conversion code to include error-checking routines to ensure robust program execution. We’ll verify whether the converted integer falls within the range of a long integer, whether the conversion itself was successful, and whether there are any characters following the valid integer.
#include <errno.h>
#include <limits.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: ./%s int1 int2 int3...\n", argv[0]);
exit(EXIT_FAILURE);
}
for (int i = 1; i < argc; ++i) {
char *str = argv[i];
char *endptr;
errno = 0;
long num = strtol(str, &endptr, 10);
if (str == endptr) {
fprintf(stderr, "%s: not a decimal number\n", str);
} else if ('\0' != *endptr) {
fprintf(stderr, "%s: extra characters at end of input: %s\n", str,
endptr);
} else if ((LONG_MIN == num || LONG_MAX == num) && ERANGE == errno) {
fprintf(stderr, "%s: out of range of type long\n", str);
} else if (errno != 0 && num == 0) {
fprintf(stderr, "%s: no conversion was performed\n", str);
} else {
printf("Converted %s to integer: %ld\n", str, num);
}
}
exit(EXIT_SUCCESS);
}
Here, we enhance the conversion process by iterating over each argument passed to the program. For each argument, we perform error checking using conditions similar to those in the previous example.
We check if the address of str
is equal to endptr
, indicating that no digits were found in the string, which implies that it’s not a valid decimal number. If this condition holds true, we print an error message to the stderr
stream.
Next, we check if the character pointed to by endptr
is not null, which would imply that there are extra characters after the valid integer. If this condition holds true, we print an error message to the stderr
stream.
We then check if the converted number falls within the range of a long integer by comparing it to LONG_MIN
and LONG_MAX
and checking if errno
is set to ERANGE
.
If this condition holds true, it indicates that the converted number is out of the range of a long integer. We print an error message to the stderr
stream accordingly.
Finally, we check if errno
is set and if the converted number is zero, which indicates that no conversion was performed. If this condition holds true, we print an error message to the stderr
stream.
If none of the error conditions are met, it indicates a successful conversion.
Code Output:
How to Convert char*
to int
in C Using the atoi
Function
Converting a char*
(a pointer to a string of characters) to an int
in C can also be achieved using the atoi
function.
The atoi
function is a part of the C Standard Library (<stdlib.h>
) and stands for ASCII to Integer. It takes a string of characters (char*
) as input and converts it into an integer representation.
Here’s the basic syntax of the atoi
function:
int atoi(const char *str);
The atoi
function parses the initial portion of the string pointed to by str
and interprets it as an integer. It stops parsing the string when it encounters a character that cannot be part of a valid integer (e.g., a non-numeric character).
Example 1: Convert char*
Array to int
Using atoi
Let’s illustrate the usage of the atoi
function with a simple example:
#include <stdio.h>
#include <stdlib.h>
int main() {
char *arr[] = {"12", "33", "43", "23", "90"};
size_t len = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < len; ++i) {
int num = atoi(arr[i]);
printf("%4d | ", num);
}
printf("\n");
return 0;
}
In this code, we begin by initializing an array called arr
containing several string literals, each representing a numeric value. We determine the length of this array using the sizeof
operator and divide it by the size of its first element, which gives us the number of elements in the array.
Next, we enter a for
loop to iterate over each element of the arr
array. Within this loop, we call the atoi
function for each string element.
The atoi
function parses the string as an integer and returns the result. We assign this converted integer value to the variable num
.
Finally, we print the converted integer value to the console using printf
.
Code Output:
Example 2: Convert Program Arguments to int
Using atoi
Let’s take a look at another example where we take program arguments as input strings and convert them into long integers using atoi
.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: ./%s int1 int2 int3...\n", argv[0]);
exit(EXIT_FAILURE);
}
for (int i = 1; i < argc; ++i) {
int num = atoi(argv[i]);
printf("%4d | ", num);
}
printf("\n");
return 0;
}
In this code, we begin by checking if the user provided at least one argument to the program. If not, we print a usage message to indicate how the program should be used and exit with a failure status.
Assuming the user has provided arguments, we enter a for
loop to iterate over each argument passed to the program, starting from index 1 to skip the program name itself (argv[0]
). Within this loop, we call the atoi
function for each argument.
Similar to the previous example, the atoi
function parses the string as an integer and returns the result. We assign this converted integer value to the variable num
.
Finally, we print the converted integer value to the console using printf
.
Code Output:
These code examples demonstrate how to efficiently convert char*
strings to integers in C using the atoi
function.
Note: The
atoi
function does not perform any error checking. If the input string is not a valid representation of an integer, the behavior ofatoi
is undefined. Therefore, it’s advisable to validate the input string before callingatoi
if the possibility of invalid input exists. For more robust error handling and validation, consider using alternative methods likestrtol
discussed in previous examples.
Conclusion
Converting a char*
(a pointer to a string of characters) to an int
in C can be accomplished using various methods provided by the C standard library. Throughout this article, we explored two primary functions for this task: strtol
and atoi
.
The strtol
function offers versatility and robust error handling, allowing for precise control over the conversion process. It allows specifying the base of the number to be converted and provides mechanisms for error detection and reporting.
On the other hand, the atoi
function provides a simpler approach for straightforward conversions. While it lacks error-checking capabilities, it offers ease of use for cases where error handling is not critical.
In practice, the choice between strtol
and atoi
depends on the specific requirements of the application. For scenarios requiring comprehensive error handling and flexibility, strtol
is the preferred option.
However, for simpler use cases where error checking is not a primary concern, atoi
provides a straightforward solution.
Overall, understanding the differences and functionalities of these functions empowers developers to make informed decisions when converting char*
strings to integers in C, ensuring efficient and reliable program execution.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook