JavaScript String.endsWith() Method
-
Syntax of JavaScript
string.endsWith()
: -
Example Codes: Find Multiple Characters at the End of a String Using the
string.endsWith()
Method -
Example Codes: Search if String Ends With a Single Character Using the
string.endsWith()
Method -
Example Codes: Search Substring of a String With the
len
Parameter of JavaScriptstring.endsWith()
Method
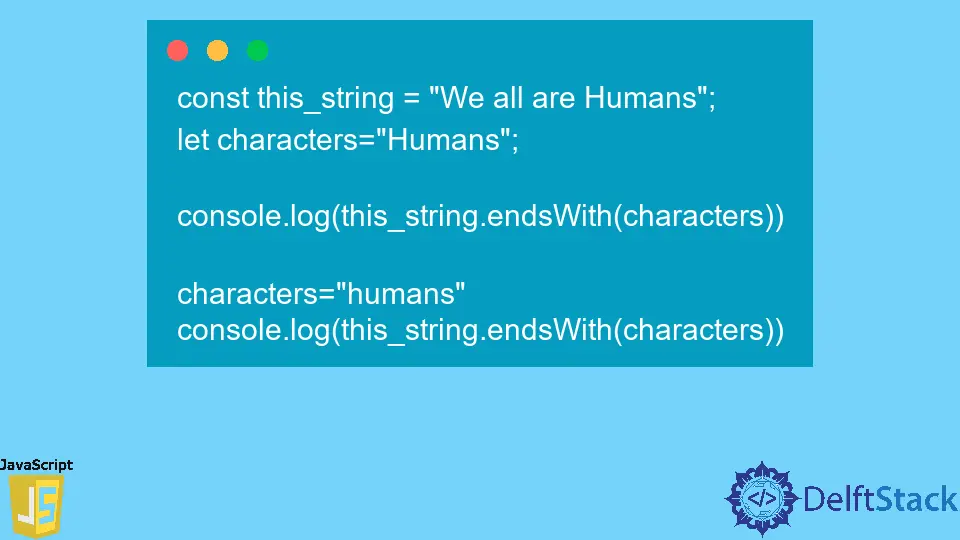
The JavaScript string.endsWith()
method produces a Boolean object that indicates if the provided character(s) are at the end of the original string or not without changing the original string.
Syntax of JavaScript string.endsWith()
:
this_string.endsWith(characters);
this_string.endsWith(characters, len);
Parameters
characters |
characters is a string-type object consisting of characters that need to be looked for at the end of the this_string . |
len (optional) |
If len is specified, the endsWith() method will look for the characters within the set length of characters from left to right in this_string . Its value is the length of this_string by default. |
Return
If the string parameter characters
is located at the end of the string, the endsWith()
function returns true. It returns false otherwise.
Example Codes: Find Multiple Characters at the End of a String Using the string.endsWith()
Method
Suppose we want to find a word at the end of the sentence.
In this example, endsWith()
searches for the term Humans
at the end of this_string
. It prints the result on the console.
const this_string = "We all are Humans";
let characters="Humans";
console.log(this_string.endsWith(characters))
characters="humans"
console.log(this_string.endsWith(characters))
Output:
true
false
We can see that when we replace the Humans
string in the characters
parameter with humans
, the endsWith()
function returns a Boolean of value false.
This demonstrates the case sensitivity of the JavaScript string endsWith()
method.
Example Codes: Search if String Ends With a Single Character Using the string.endsWith()
Method
In this example, it is determined whether a given sentence is a question or not.
let sentence = "What's your name?";
let is_question = sentence.endsWith("?");
console.log(is_question)
When the string sentence
calls the endsWith()
method with ?
as the only parameter, endsWith()
searches from left to right for ?
till the length of the sentence
string.
Output:
true
Example Codes: Search Substring of a String With the len
Parameter of JavaScript string.endsWith()
Method
This example will search for the word all
till the specified length of characters in the this_string
.
Setting the len
parameter in the JavaScript string endsWith()
method causes it to search for the provided word within the given length passed in the len
parameter.
const this_string = "We all are Humans";
const characters="all";
let len=6;
console.log(this_string.endsWith(characters));
console.log(this_string.endsWith(characters,len));
Output:
false
true
When passing characters
parameters as all
with the len
parameter not set, its value is the full length of the this_string
calling object; therefore, the string endsWith()
method returns false.
The JavaScript string endsWith()
method returns a boolean
having a value of true
as the string all
in the characters
parameter is located at the end of the first 6 characters of this_string
string object.