JavaScript String.localeCompare() Method
-
Syntax of JavaScript
string.localeCompare()
: -
Example Codes: Use the
string.localeCompare()
Method to Compare String With the Required ParametercompareString
-
Example Codes: Use the
string.localeCompare()
Method to Find a String With the Optional Parameterslocales
andoptions
-
Example Codes: Use the
string.localeCompare()
Method to Sort Array of Strings Alphabetically
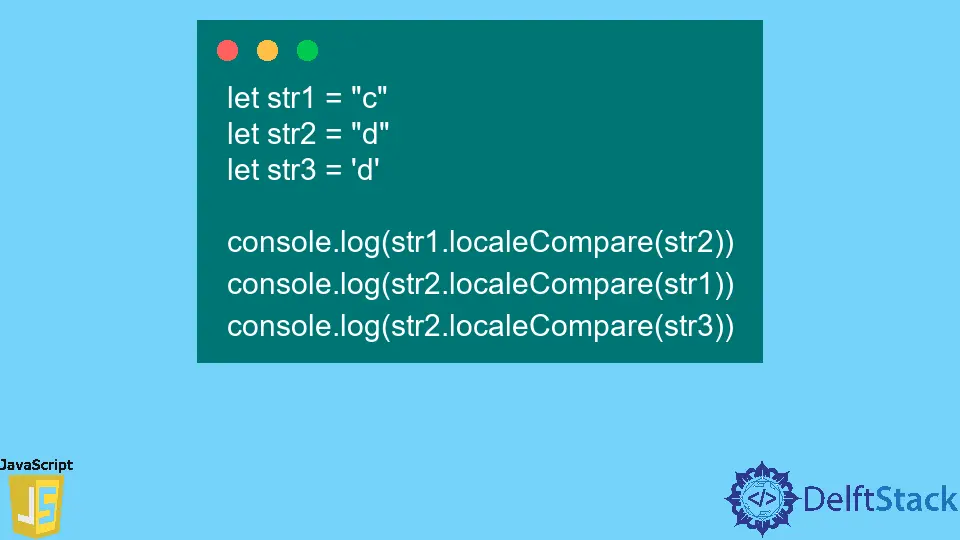
In JavaScript, the string.localeCompare()
method is used to compare two strings. This method follows the sort order and checks whether the reference string comes before, after, or at the same position as the compared string.
Syntax of JavaScript string.localeCompare()
:
referenceString.localeCompare(compareString, locales, options)
Parameters
compareString (required) |
This refers to a string that will be compared with the reference string. |
locales (optional) |
locales refers to the language and different countries’ languages that can be passed. By default, it uses the browser’s default language. |
options (optional) |
It refers to an Object consisting of punctuation, numeric, etc., used to make the result more perfect. |
Return
A numeric value is returned based on comparing the reference string and compared string.
-1
is returned if the reference string comes before the compared string.0
is returned if the reference and compared strings are equal.1
is returned if the reference string comes after the compared string.
Example Codes: Use the string.localeCompare()
Method to Compare String With the Required Parameter compareString
let str1 = "c"
let str2 = "d"
let str3 = 'd'
console.log(str1.localeCompare(str2))
console.log(str2.localeCompare(str1))
console.log(str2.localeCompare(str3))
Output:
-1
1
0
First, str1 (c)
is compared with str2 (d)
. Here, alphabetically sorting order, c
comes before d
, so it returns -1
.
Second, we tweaked these two strings. We provided d
as the reference string and c
as the compared string. Here, d
comes after the c
, so it returns 1
.
Third, we provided the same value to compare. The value of str2
is equal to str3
; as a result, it returns 0
.
Example Codes: Use the string.localeCompare()
Method to Find a String With the Optional Parameters locales
and options
let str1 = "CaT"
let str2 = "cAt"
console.log(str1.localeCompare(str2))
console.log(str1.localeCompare(str2, 'en', { sensitivity: 'base'}))
Output:
1
0
First, we provided only the required parameter, str2
, and compared it with the reference string str1
. Both are in case-sensitive form.
In this case, the reference string comes after the compared string, so it returns 1
.
Second, we provided the optional parameters along with the required parameter. en
refers to English, and sensitivity: 'base'
is used to make the strings case-insensitive.
As a result, they became equal and returned 0
.
Example Codes: Use the string.localeCompare()
Method to Sort Array of Strings Alphabetically
let strArr = [ 'c', 'java', 'JavaScript', 'python', 'Go', 'rust' ];
let result = strArr.sort((a, b) => a.localeCompare(b));
console.log(result)
Output:
[ 'c', 'Go', 'java', 'JavaScript', 'python', 'rust' ]
We provided an array of strings that considered both uppercase and lowercase letters. The string.localeCompare()
method makes them case-insensitive and sorts the array of strings alphabetically.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn