使用 Java 批量更新 MongoDB 中的文件
- 先決條件
- 使用 Java 批量更新 MongoDB 中的文件
-
使用
updateMany()
通過向現有文件新增新欄位來執行批量更新 -
使用
updateMany()
對匹配過濾器的多個文件中的現有欄位執行批量更新 -
使用
updateMany()
對匹配過濾器的嵌入文件執行批量更新 -
使用
bulkWrite()
API 更新與過濾查詢匹配的現有文件
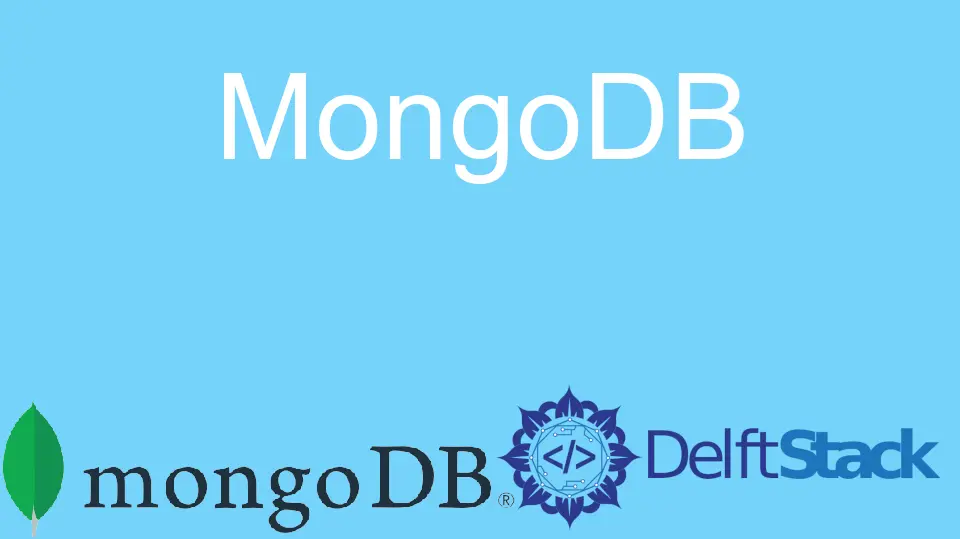
本教程是關於使用 Java 驅動程式在 MongoDB 中執行文件的批量更新。我們還將學習如何通過新增新欄位、更改現有欄位或陣列的值或刪除文件來進行更新。
先決條件
對於本教程,我們將使用你必須遵循的以下工具。
- Java(我們使用的是 Java 18.0.1.1)
- MongoDB 伺服器(我們使用的是 MongoDB 5.0.8)
- 程式碼編輯器或任何 Java IDE(我們使用的是 Apache NetBeans IDE 13)
- 使用 Maven 或 Gradle 的 Mongo Java Driver Dependencies; 我們在 Maven 中使用它。
使用 Java 批量更新 MongoDB 中的文件
當我們需要更新資料庫中的多個文件時,批量更新很有用。現在,問題是,我們要如何更新文件?
我們是否想通過新增新欄位、更新現有欄位或陣列的值或刪除文件來進行更新?要了解所有這些場景,讓我們建立一個集合並插入兩個文件。
建立集合:
db.createCollection('supervisor');
插入第一個文件:
db.supervisor.insertOne(
{
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact" : {
"email":"mehvishofficial@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123456789"
},
{
"type" : "personal",
"number" : "124578369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 1,3,4,5,6,7 ] },
{ "_id" : 2, "itemperday" : [ 2,3,4,5,2,7 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,4,0,1 ] }
]
}
);
插入第二個檔案:
db.supervisor.insertOne(
{
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact" : {
"email":"tahirraza@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123478789"
},
{
"type" : "personal",
"number" : "122378369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 4,5,6,7,4,6 ] },
{ "_id" : 2, "itemperday" : [ 2,3,2,7,5,2 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,1,0,0 ] }
]
}
);
填充集合後,你可以使用 db.supervisor.find().pretty();
檢視插入的文件。我們將從簡單的解決方案開始,然後轉向一些棘手但有趣的解決方案。
使用 updateMany()
通過向現有文件新增新欄位來執行批量更新
示例程式碼:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.BasicDBObject;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_1
public class Example_1 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update documents by adding a new field and its value
collection.updateMany(
new BasicDBObject(), new BasicDBObject("$set", new BasicDBObject("status", "Good")));
// print a new line
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_1
輸出:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
我們已經為你格式化了輸出(如上所示),但你將在 IDE 上每行看到一個文件。我們也可以使用 db.supervisor.find().pretty();
在 mongo shell 上檢視更新的文件。
對於此示例程式碼,我們使用 mongo 集合物件的 updateMany()
方法,該方法接受 filter
查詢和 update
語句來更新匹配的文件。在這裡,我們不是過濾文件,而是向所有現有文件新增一個名為 status
的新欄位(參見上面給出的輸出)。
使用 updateMany()
對匹配過濾器的多個文件中的現有欄位執行批量更新
示例程式碼:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import static com.mongodb.client.model.Filters.eq;
import static com.mongodb.client.model.Updates.combine;
import static com.mongodb.client.model.Updates.set;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_2
public class Example_2 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update the filtered documents by updating the existing field
collection.updateMany(eq("gender", "Female"), combine(set("gender", "F")));
collection.updateMany(eq("gender", "Male"), combine(set("gender", "M")));
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_2
輸出:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
此程式碼示例與上一個示例類似,但我們正在更新與過濾器匹配的所有文件中特定欄位的值。
我們使用 updateMany()
對 gender
等於 Female
且 gender
等於 Male
的所有文件執行批量更新。我們將 "gender": "Female"
和 "gender": "Male"
分別更改為 "gender":"F"
和 "gender":"M"
。
到目前為止,我們已經瞭解瞭如何使用 updateMany()
方法新增新欄位或更新第一級現有欄位的值。接下來,我們將學習使用 updateMany()
對滿足過濾器查詢的嵌入式文件進行批量更新。
使用 updateMany()
對匹配過濾器的嵌入文件執行批量更新
示例程式碼:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.UpdateOptions;
import org.bson.Document;
import org.bson.conversions.Bson;
// Example_3
public class Example_3 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// filter
Bson where = new Document().append("contact.phone.type", "official");
// update
Bson update = new Document().append("contact.phone.$.type", "assistant");
// set
Bson set = new Document().append("$set", update);
// update collection
collection.updateMany(where, set, new UpdateOptions());
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_3
輸出:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "assistant", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
在這個 Java 程式中,我們使用 updateMany()
方法對匹配過濾器查詢的嵌入文件執行批量更新。
在這裡,我們將 contact.phone.type
的值從 official
更新為 assistant
。要訪問文件陣列,我們使用位置運算子 ($
) 來更新滿足過濾器查詢的第一個陣列值。
在使用位置運算子時,我們還可以指定應該更新哪些陣列元素。我們可以指定要更新的第一個、所有或特定的陣列元素。
為了通過位置運算子指定陣列元素,我們使用 dot notation
,一種用於導航 BSON
物件的屬性訪問語法。我們通過以下方式使用位置運算子來更新第一個、所有或指定的陣列元素。
-
我們使用位置運算子(
$
)來更新滿足過濾查詢的陣列的第一個元素,而陣列欄位必須是過濾查詢的一部分才能使用位置運算子。 -
我們使用所有位置運算子(
$[]
)來更新陣列的所有專案(元素)。 -
過濾位置運算子 (
$[<identifier>]
) 用於更新與過濾查詢匹配的陣列元素。在這裡,我們必須在更新操作中包含陣列過濾器,以告知哪些陣列元素需要更新。請記住,
<identifier>
是我們為陣列過濾器指定的名稱。此值必須以小寫字母開頭,並且只能包含字母數字字元。
使用 bulkWrite()
API 更新與過濾查詢匹配的現有文件
示例程式碼:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.DeleteOneModel;
import com.mongodb.client.model.UpdateManyModel;
import com.mongodb.client.model.WriteModel;
import java.util.ArrayList;
import java.util.List;
import org.bson.Document;
// Example_4
public class Example_4 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// create object
List<WriteModel<Document>> writes = new ArrayList<>();
// delete the document matching the filter
writes.add(new DeleteOneModel<>(new Document("firstname", "Tahir")));
// update document matching the filter
writes.add(new UpdateManyModel<>(new Document("status", "Good"), // filter
new Document("$set", new Document("status", "Excellent")) // update
));
// bulk write
collection.bulkWrite(writes);
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
catch (MongoException me) {
System.err.println("An error occurred while running a command: " + me);
} // end catch block
} // end main method
} // end Example_4
輸出:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{"type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Excellent"
}
在此示例中,我們使用 bulkWrite()
API 一次執行多個操作(刪除和更新)。DeleteOneModel()
用於刪除匹配過濾器的文件,而我們使用 UpdateManyModel()
更新所有匹配過濾器的文件中已存在欄位的值。