在 JavaScript 中為一個元素設定多個屬性
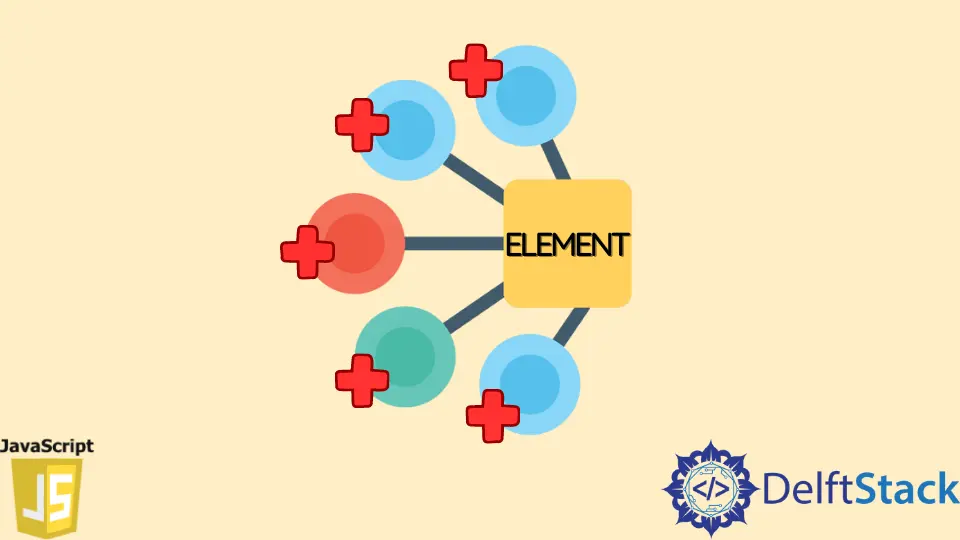
在本教程文章中,我們將討論一種藉助 JavaScript 為元素設定多個屬性的方法。
在 JavaScript 中為一個元素設定多個屬性
要使用 JavaScript 一次為一個元素設定多個屬性,你需要:
-
將屬性名稱和值新增到物件;
-
使用
Object.keys()
方法獲取物件鍵的陣列; -
使用
forEach()
方法迭代鍵陣列; -
使用
setAttribute()
方法將每個屬性及其值新增到元素中。
下面,你可以找到本文所有示例中使用的 HTML 程式碼。
<html lang="en">
<head>
<meta charset="UTF-8" />
</head>
<body>
<button id="btn">Button 1</button>
<script src="index.js"></script>
</body>
</html>
你可以在下面找到相關 JavaScript 程式碼的示例。
function setAttributes(element, attributes) {
Object.keys(attributes).forEach(attr => {
element.setAttribute(attr, attributes[attr]);
});
}
const attributes = {
name: 'example',
title: 'Box 1',
disabled: '',
style: 'background-color: salmon; color: white;',
};
const button = document.getElementById('btn');
setAttributes(button, attributes);
將建立一個可重用函式來獲取元素和屬性名稱和值的物件並將它們新增到元素中。我們將使用 Object.keys()
方法來獲取屬性名稱的陣列。
const attributes = {
name: 'example',
title: 'Box 1',
disabled: '',
style: 'background-color: salmon; color: white;',
};
// ['name', 'title', 'disabled', 'style']
console.log(Object.keys(attributes));
輸出:
將函式與陣列中的每個元素一起傳遞給 Array.forEach
方法。然後,使用 setAttribute
方法在每次迭代時為元素設定一個屬性。
如果該屬性已存在於元素中,則該值將被更新。否則,將新增具有指定值和名稱的新屬性。
使用 setAttribute
方法時,你可以設定或替換元素中存在的屬性。例如,如果元素具有 style
屬性並使用 setAttribute
方法更新其現有 style
,你將使用提供的值替換當前樣式。
要記住的重要一點是,當布林屬性的值設定為 disabled
時,你可以為該屬性指定任何值,並且它將起作用。但是,如果該屬性存在,則無論其值如何,其值都將被視為真。
假設不存在布林屬性,例如 false。在這種情況下,屬性的值被認為是假的。這就是為什麼最好將布林屬性設定為空字串,這將在元素上設定屬性而不需要值。
從 JavaScript 中的元素中刪除多個屬性
你可以利用 removeAttribute()
方法從元素中刪除屬性。
const button = document.getElementById('btn');
button.removeAttribute('name');
如果要從一個元素中刪除多個屬性,可以在新增各種屬性時使用相同的方法。
這一次,事情有點簡單,因為你只需要刪除屬性的名稱;因此,你可以使用陣列而不是物件。
function removeAttributes(element, attributes) {
attributes.forEach(attr => {
element.removeAttribute(attr);
});
}
const attributes = ['name', 'title', 'disabled', 'style'];
const button = document.getElementById('btn');
removeAttributes(button, attributes);
將屬性名稱儲存在陣列中,並使用 forEach
方法遍歷陣列。最後,使用 removeAttribute
方法在每次迭代時從元素中刪除屬性。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.