Angular 中的物件陣列
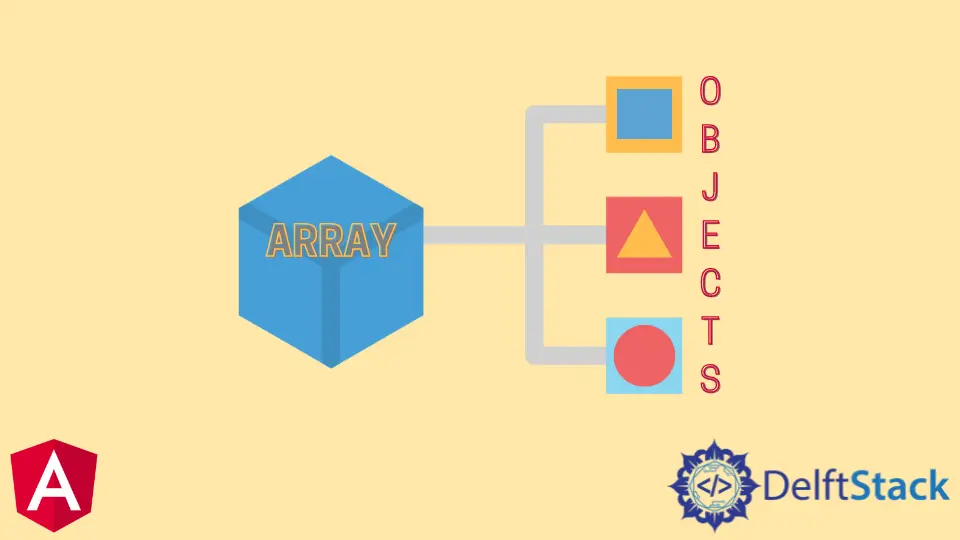
在本文中,我們通過示例討論 Angular 中的物件陣列及其工作原理。
Angular 中的物件陣列
在處理 Angular 應用程式時,在許多情況下我們必須建立物件陣列。讓我們從 TypeScript 中的物件陣列的示例開始。
我們必須通過賦予物件陣列的值和型別來宣告物件陣列,以儲存物件陣列。填充物件陣列並顯示單選按鈕顯示或下拉選單。
有很多方法可以建立陣列的物件。我們可以使用宣告的任何物件型別來宣告和重置陣列。
物件具有包含鍵和值的屬性。我們將展示如何在給定的語法中宣告和重置字串陣列。
# Angular
shapes:Array<string> = ['Triangle','Square','Rectangle'];
輸出:
在 typescript 中,我們可以使用任何型別宣告一個 Object。讓我們在以下示例中建立一個 fruits
物件。
# Angular
public fruits: Array<any> = [
{ title: "Banana", color: "Yellow" },
{ title: "Apple", color: "Red" },
{ title: "Guava", color: "Green" },
{ title: "Strawberry", color: "Red" }
];
object(<any>
) 型別可以被替換。
# Angular
public fruits: Array<object> = [
{ title: "Banana", color: "Yellow" },
{ title: "Apple", color: "Red" },
{ title: "Guava", color: "Green" },
{ title: "Strawberry", color: "Red" }
];
Angular 中的別名物件型別陣列
在 typescript 中操作 type 關鍵字允許為自定義型別建立新別名。設計了 Fruit
物件別名並組裝了一個別名型別陣列。
# AngularJs
type Fruit = Array<{ id: number; name: string }>;
在下一個示例中,我們建立陣列型別的 Fruit
別名並使用物件值初始化 Array。
程式碼 - app.component.ts
:
# Angular
import { Component } from '@angular/core';
type Fruit = Array<{ id: number; name: string }>;
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
fruits: Fruit = [
{ id: 1, name: "Apple" },
{ id: 2, name: "Banana" },
{ id: 3, name: "Guava" },
{ id: 4, name: "Strawberry" }
];
constructor() {}
ngOnInit() {}
}
現在,我們在前端檔案中顯示物件。
程式碼 - app.component.html
:
# angular
<ul>
<li *ngFor="let fruit of fruits">{{fruit.name}}</li>
</ul>
輸出:
我們可以使用介面型別宣告一個物件陣列。如果物件具有多個屬性並且難以處理,則開銷方法存在一些缺陷,並且這種方法建立了一個介面來儲存 Angular 和 typescript 中的物件資料。
通過 REST API(RESTful APIs
)處理從後端/資料庫到達的資料很有用。我們可以使用如下所示的命令來製作介面。
# Angular
ng g interface Fruit
它開發了一個 fruit.ts
檔案。
# Angular
export interface fruits {
id: number;
name: string;
constructor(id,name) {
this.id = id;
this.name = name;
}
}
在 Angular TypeScript 元件中,我們可以為水果製作一個介面。
# Angular
import { Component, OnInit } from "@angular/core";
import {Fruit} from './Fruit'
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class appComponent implements OnInit {
public fruits: Fruit[] = [
{ id: 1, name: "Apple" },
{ id: 2, name: "Banana" },
{ id: 3, name: "Guava" },
{ id: 4, name: "Strawberry" }
];
constructor() {}
ngOnInit() {
console.log(this.fruits)
}
}
輸出:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn