Tkinter Tutorial - Label Widget
-
Python Tkinter
Label
Widget - Change Python Label Font
- Change Python Tkinter Label Color(s)
- Display Image in Python Tkinter Label
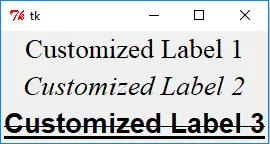
Tkinter Label
widget displays a text string or an image, whose content is normally supposed not to be dynamic. Of course, you could change its content if you like.
Python Tkinter Label
Widget
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
app = tk.Tk()
labelExample = tk.Label(app, text="This is a Label")
labelExample.pack()
app.mainloop()
It generates a window with a text label inside the main window.
labelExample = tk.Label(app, text="This is a label")
labelExample
is a Label instance displaying This is a label
with the parent app
.
labelExample.pack()
pack()
method manages the layout of the particular widget in the parent widget. It has options as below
pack() Method |
Description |
---|---|
after=widget |
pack it after you have packed widget |
anchor=NSEW (or subset) |
position widget according to |
before=widget |
pack it before you will pack widget |
expand=bool |
expand widget if parent size grows |
fill=none or x or y or both |
fill widget if widget grows |
in=master |
use master to contain this widget |
in_=master |
see in option description |
ipadx=amount |
add internal padding in x direction |
ipady=amount |
add internal padding in y direction |
padx=amount |
add padding in x direction |
pady=amount |
add padding in y direction |
side=top or bottom or left or right |
where to add this widget. |
You could change the options to get the different label widget layout.
The dimension of the label is specified by width and height that belong to label’s widget-specific options.
Width and height have the unit of text units when it contains text, and its size is pixels if it displays image.
Refer to this article to check what text unit is and how to set the label size in the unit of pixels.
You could get label properties with the command dict(label)
,
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
from pprint import pprint
app = tk.Tk()
labelExample = tk.Label(app, text="This is a Label", height=15, width=100)
pprint(dict(labelExample))
The properties are listed below
{'activebackground': 'SystemButtonFace',
'activeforeground': 'SystemButtonText',
'anchor': 'center',
'background': 'SystemButtonFace',
'bd': <pixel object at 00000000048D1000>,
'bg': 'SystemButtonFace',
'bitmap': '',
'borderwidth': <pixel object at 00000000048D1000>,
'compound': 'none',
'cursor': '',
'disabledforeground': 'SystemDisabledText',
'fg': 'SystemButtonText',
'font': 'TkDefaultFont',
'foreground': 'SystemButtonText',
'height': 15,
'highlightbackground': 'SystemButtonFace',
'highlightcolor': 'SystemWindowFrame',
'highlightthickness': <pixel object at 00000000048FF100>,
'image': '',
'justify': 'center',
'padx': <pixel object at 00000000048FED40>,
'pady': <pixel object at 00000000048FF0D0>,
'relief': 'flat',
'state': 'normal',
'takefocus': '0',
'text': 'This is a Label',
'textvariable': '',
'underline': -1,
'width': 100,
'wraplength': <pixel object at 00000000048FED70>}
Now you know label’s properties, then you could get different label appearances by changing them.
Change Python Label Font
We will show you different label font configuration methods below.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
import tkFont
app = tk.Tk()
labelExample1 = tk.Label(app, text="Customized Label 1", font=("Times", 20))
labelExample2 = tk.Label(app, text="Customized Label 2", font=("Times", 20, "italic"))
labelFont3 = tkFont.Font(
family="Helvetica", size=20, weight=tkFont.BOLD, underline=1, overstrike=1
)
labelExample3 = tk.Label(app, text="Customized Label 3", font=labelFont3)
labelExample1.pack()
labelExample2.pack()
labelExample3.pack()
app.mainloop()
Set Python Tkinter Label Font With a Tuple
labelExample1 = tk.Label(app, text="Customized Label 1", font=("Times", 20))
labelExample2 = tk.Label(app, text="Customized Label 2", font=("Times", 20, "italic"))
A tuple with font name type as its first element, followed by size, style like weight, italic, underline and/or overstrike.
Set Python Tkinter Label Font With tkFont
Font Object
labelFont3 = tkFont.Font(
family="Helvetica", size=20, weight=tkFont.BOLD, underline=1, overstrike=1
)
labelExample3 = tk.Label(app, text="Customized Label 3", font=labelFont3)
You could also use font object in tkFont
module to specify the label text font properties.
The font type in labelExample3
is font family Helvetica
, size 20, bold, underlined and overstrike.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
import tkFont
from pprint import pprint
app = tk.Tk()
pprint(tkFont.families())
Change Python Tkinter Label Color(s)
You could change label foreground and background color using fg
/bg
properties.
labelExample1 = tk.Label(app, text="Customized Color", bg="gray", fg="red")
Display Image in Python Tkinter Label
The image
property in label
is used to display the image in the label.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
app = tk.Tk()
logo = tk.PhotoImage(file="python.gif")
labelExample = tk.Label(app, image=logo)
labelExample.pack()
app.mainloop()
tk.PhotoImage
could only display colored images in GIF, PPM/PGM format. It generates _tkinter.TclError: couldn't recognize data in image file
if you use other formats of imageFounder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook