How to Declare Map or List Type in TypeScript
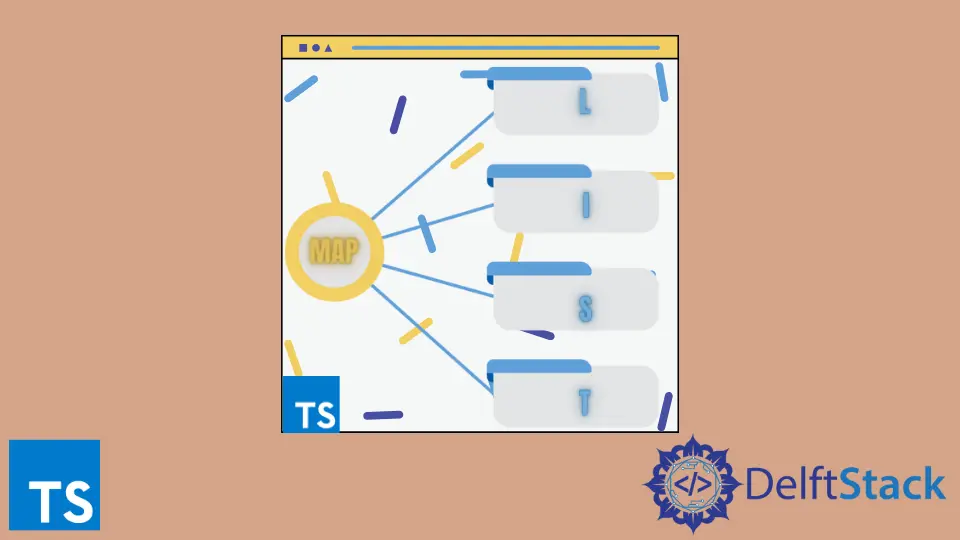
Maps and lists are the basic data structures used in every programming language for writing application logic. A map is used to quickly retrieve data items from a store, while a list is a data structure where data items are stored sequentially.
TypeScript on its own does not support Map or List types; however, it is very easy to create the required Types using the built-in types in TypeScript.
Declare Record
and Map
Type in TypeScript
TypeScript has introduced the Record
type, which can support map types with key-value pairs and has generic types for supporting maps of different types.
More specifically, Record<K,V>
denotes that an object accepts keys of only type K
, and the values corresponding to the keys should be of type V
.
key of Record<K,V>
would yield K
as the type, and Record<K,V>[K]
is equivalent to V. The Record
type is an alias for index signatures like { [ key : K] : V }
.
const colorMap : Record<string, string> = {
'ylw' : 'yellow',
'blk' : 'black',
'bl' : 'blue'
};
Thus using the generic types, we can have multiple types for the Map or Record
type in TypeScript. After compilation and the following typed object becomes a simple object in JavaScript.
Apart from the typed object, native Javascript has the Map
function, which can initialize a new Map
instance. It has quite of supported functions associated with it.
The following code segment will demonstrate how the Map
function can be used to initialize a new Map
object.
const colorMap = new Map<string, string>();
colorMap.set("ylw", "yellow");
colorMap.set("blk", "black");
colorMap.forEach( ( v, k , _) => {
console.log( "key : " + k + ", value : " + v);
})
// keys
var keyList : string[] = [...colorMap.keys()];
console.log(keyList);
// values
var valueList : string[] = [...colorMap.values()];
console.log(valueList);
Output:
"key : ylw, value : yellow"
"key : blk, value : black"
["ylw", "blk"]
["yellow", "black"]
Apart from the functions shown, it also supports other functions like delete
and clear
. These are used for deleting a single entry from the Map or clearing all the entries from the Map, respectively,
Declare List
Type in TypeScript
There is no built-in list
type in TypeScript; however, TypeScript provides the Array
type for storing contiguous data elements. It is easy to create a list data structure ADT using the Array
type.
class List<T> {
private items : Array<T>;
constructor(n? : number, defaultValue? : T){
if ( n === undefined) {
this.items = [];
} else {
if ( n && defaultValue){
this.items = Array(n).fill(defaultValue);
} else {
this.items = Array(n);
}
}
}
push(item : T){
this.items.push(item);
}
pop(item : T){
return this.items.pop();
}
get(index : number) : T | undefined {
return this.items[index];
}
set( index : number, item : T){
this.items[index] = item;
}
getItems() : Array<T> {
return this.items;
}
}
List.prototype.toString = function listToString(){
return JSON.stringify(this.getItems());
}
var list : List<string> = new List(5, "default");
list.set(1, "second");
list.set(0, "first");
console.log(list.toString());
Output:
"["first","second","default","default","default"]"
The above shows a basic example for constructing a list ADT; with some basic functions, it can easily be extended to support any list required in the application logic.