Z-Index in React Native
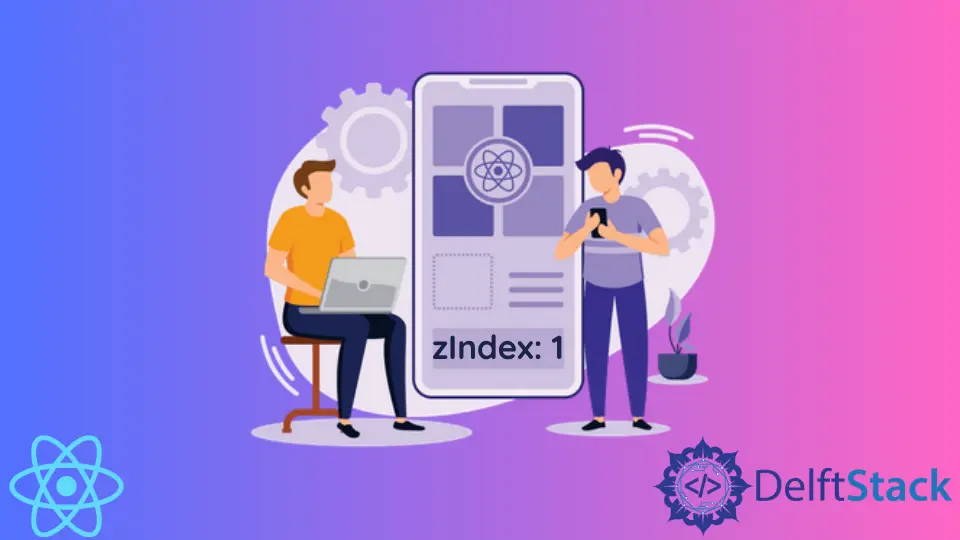
We will introduce an example of how to use zIndex
in react native applications.
Use the zIndex
to Specify the Stack Order of an Element in React Native
The zIndex
is used to specify the stack order of an element in react native applications.
The elements can be placed in front of the other elements by giving them the higher value of the zIndex
. If we want to display an element between 2 elements, we can assign a larger zIndex
value.
That will be at the top and one low zIndex
value to the element. It requires to be displayed in the middle and the lowest value.
It will be displayed beneath both of the elements. There are some rules in which zIndex
works. It only works on the elements positioned as absolute
, sticky
, fixed
, or relative
.
Let’s go through an example with 3 cards and try them positioning with different zIndex
values. So let’s create a new react native application using expo cli
.
We will install expo-cli
using the npm command.
# react native
npm install -g expo-cli
If you use yarn
instead of npm
, you can use the following command.
# react native
yarn global add expo-cli
Now we will create a new React Native project using the expo
command.
# react native
expo init MyApp
This command will create a new project, MyApp
. The same command is used on npm
and yarn
users.
We will go into our project directory and start the app using the npm command.
# react native
cd MyApp
npm start
If you are using yarn
.
# react native
cd MyApp
yarn start
We can also start our app by running the expo command.
# react native
cd MyApp
expo start
Now, in our App.js
, we will import Text
, View
, Stylesheet
. We will also import constants and cards.
# react native
import * as React from 'react';
import { Text, View, StyleSheet } from 'react-native';
import Constants from 'expo-constants';
import { Card } from 'react-native-paper';
In return
, we will create our view that will contain 3 cards.
# react native
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.paragraph}>
Z Index Example
</Text>
<Card style={styles.card}>
<Text style={styles.paragraph}>
This is a card
</Text>
</Card>
<Card style={styles.card2}>
<Text style={styles.paragraph}>
This is a card
</Text>
</Card>
<Card style={styles.card3}>
<Text style={styles.paragraph}>
This is a card
</Text>
</Card>
</View>
);
}
We will style our cards to overlap but not completely cover each other. We will need to assign negative margins to get the result we want.
So our code will look like below.
# react native
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: Constants.statusBarHeight,
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
card: {
margin: 24,
marginBottom: 0,
height: 240,
},
card2: {
margin: 24,
marginBottom: 0,
height: 200,
width: 140,
marginTop: -150,
backgroundColor: '#900',
},
card3: {
margin: 24,
marginBottom: 12,
height: 100,
marginTop: -100,
backgroundColor: '#6ED4C8',
},
});
Output:
So, As you can see in the above example, by changing the width and height of the three cards, we can make them overlap each other.
Let’s play with zIndex
to place elements on screen using zIndex. So, we will first assign zIndex:1
value to the white card.
# react native
card: {
margin: 24,
marginBottom: 0,
height: 240,
zIndex: 1
},
Output:
So, if we assign the zIndex
of 1 to the white card, it is displayed at the top of all elements. Only a little portion of blue is displayed because the height of the white card is small.
Assigning the value of just 1
makes the white card with the higher z index value because the rest of the 2
cards don’t have any value yet. So, the White card is displayed at the top.
Now, let’s change the z index value of the brown card and set it to 2
.
# react native
card2: {
margin: 24,
marginBottom: 0,
height: 200,
width: 140,
marginTop: -150,
backgroundColor: '#900',
zIndex: 2
},
Output:
As you can see in the above result, the brown card appears at the top of both white and blue cards because it has the bigger zIndex
value.
Now, let’s assign the value of 3 to the blue card.
# react native
card3: {
margin: 24,
marginBottom: 12,
height: 100,
marginTop: -100,
backgroundColor: '#6ED4C8',
zIndex: 3
},
Output:
Conclusion
Now, you can see in the above result that if we gave the zIndex to all elements according to the sequence in which they are coded. They would keep the structure displayed by default.
But if you want to make any element appear on top of the other elements, we can make the zIndex of that element higher than the element we want it on top of.
So by following these simple rules, we can easily use zIndex as a benefit for our mobile applications and create amazing UI’s and animations.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn