How to Parse JSON Strings in React
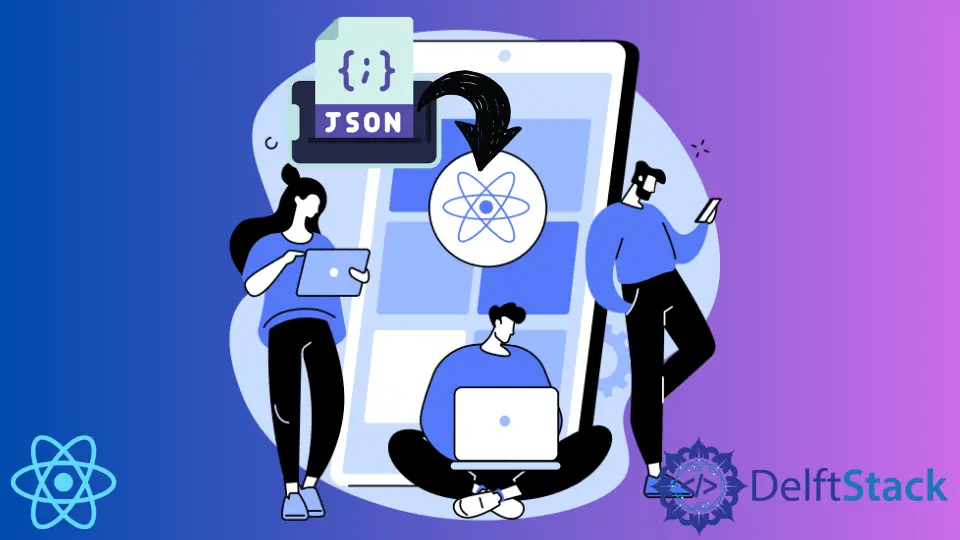
JavaScript is the language of the web. Data on the internet is often exchanged in JSON format.
Sometimes, you want to convert raw JavaScript data into JSON. Other times, you need to parse JSON data to get JavaScript data.
This article will describe the process of parsing JSON data to get valid JavaScript data.
Parse JSON Strings in React
JSON is the most common format to exchange data on the web. For this reason, JavaScript has built-in JSON.parse(str)
method to transform JSON strings to valid JavaScript objects.
Parse Local JSON Data in React
Let’s take a look at the example where we take a normal JavaScript object and format it as JSON:
export default function App() {
const sampleData = JSON.stringify({
name: "Irakli",
messages: ["hello", "goodbye", "good day"],
age: 24
});
console.log(sampleData);
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
We used the JSON.stringify()
method to store data in JSON format. If you go and check the console on CodeSandbox, data is saved as JSON.
Then, we can use the JSON.parse(str)
method to convert this data from JSON back into valid JavaScript objects.
export default function App() {
const sampleData = JSON.stringify({
name: "Irakli",
messages: ["hello", "goodbye", "good day"],
age: 24
});
console.log(sampleData);
const parsedData = JSON.parse(sampleData);
console.log(parsedData);
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
Once again, we console.log()
the data so you can see the difference between the two formats.
Use JSON.parse()
Within JSX
It is more readable to parse JSON data outside JSX. However, you can also do it within the return
statement.
Let’s take a look:
<div className="App">
<h1>{JSON.parse(sampleData).name}</h1>
</div>
You can use this code to display the name
property in the sampleData
variable.
JSX is only a templating language. It compiles into Top-level React API, which is entirely written in JavaScript, so you can freely use JSON.parse(str)
and other JavaScript methods within JSX.
Parse External JSON Data in React
Most of the time, you will receive JSON data from an external source. For these occasions, you can use the Fetch API and associated syntax to turn JSON data into regular JavaScript data.
export default function App() {
fetch("https://api.chucknorris.io/jokes/random?category=dev")
.then((res) => res.json()) // the .json() for parsing
.then((data) => console.log(data));
return (
<div className="App">
<h1></h1>
</div>
);
}
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn