Fonts in React
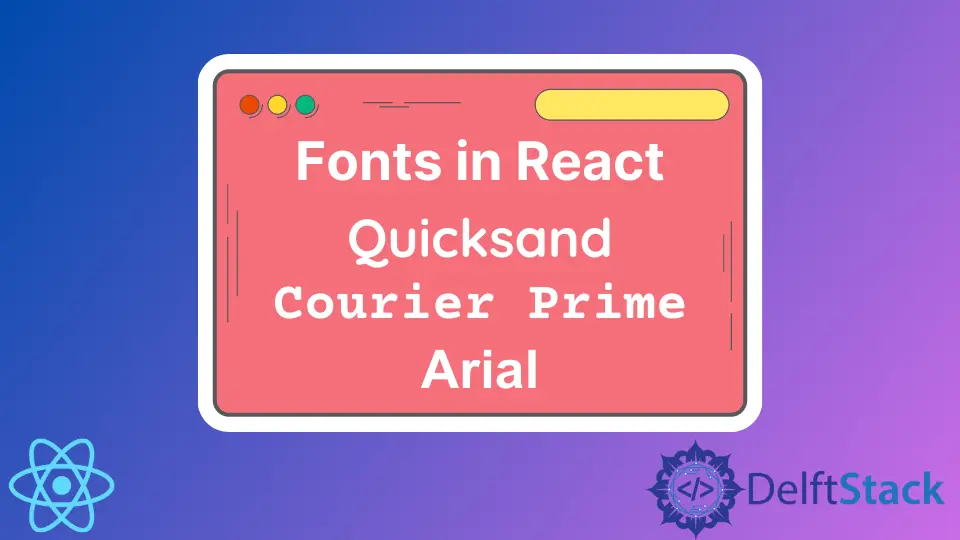
We will introduce how to install fonts and use fonts in react.
Fonts in React
Fonts are the most important part of a website. There are different ways to install fonts in react application.
The most common way is by importing a font. We can import a font using npm
or yarn
in the command prompt. Let’s install Quicksand
as an example.
# react CLI
yarn add typeface-quicksand
or
# react
npm install typeface-quicksand --save
After installing it, we need to import it into the index.tsx
file.
# react
import 'typeface-quicksand';
Now, we can use it in the style.css
file.
# react
h1,
p {
font-family: Quicksand;
}
Output:
Another way to install fonts in react is by directly adding links in index.html
or style.css
file. Let’s install Lato
font as an example.
# react
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Lato&display=swap" rel="stylesheet">
Or,
# react
<style>
@import url('https://fonts.googleapis.com/css2?family=Lato&display=swap');
</style>
Let’s import the Lato
font and change the style for heading and paragraph.
# react
@import url('https://fonts.googleapis.com/css2?family=Lato&display=swap');
h1,
p {
font-family: Lato;
}
Output:
Another way to install fonts in react is by downloading and adding it in our source code.
We can easily download fonts from fonts.google.com
and move them to the fonts
directory inside our src
directory.
Now, in style.css
, add fonts using @font-face
.
# react
@font-face {
font-family: 'Lato';
src: local('Lato'), url(./fonts/Lato-Regular.otf) format('opentype');
}
For ttf
format, we have to mention format('truetype')
, and for woff
format, we have to mention format('woff')
.
Now, we can easily style using this font.
# react
h1,
p {
font-family: Lato;
}
Output:
Another method is by using the web-font-loader
package. We can easily install a web-font-loader
package using npm
or yarn
.
# react
yarn add webfontloader
Or,
# react
npm install webfontloader --save
Now, in index.tsx
, we can import and specify the font we need.
# react
import WebFont from 'webfontloader';
WebFont.load({
google: {
families: ['Lato:300,400,700', 'sans-serif']
}
});
So now, we learned four different ways to install and use fonts in React.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn