How to CreateElement Method in React
-
Use
React.createElement()
to Create Components in React -
the
React.createElement()
Method Arguments in React
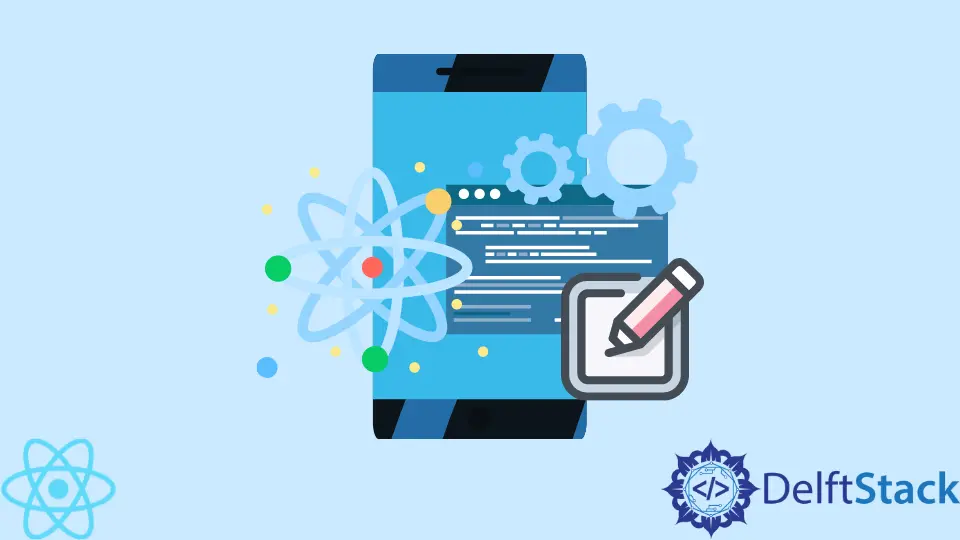
This article will explore React.createElement()
, probably the most important method in React. Many developers get comfortable with JSX but don’t know how JSX works under the hood.
JSX is a special syntax that gets compiled into calls to React.createElement()
and other methods available on top-level API in React.
Use React.createElement()
to Create Components in React
Most React developers use templating language called JSX to declaratively invoke the components and set up a structure for the application.
The team behind React framework correctly decided that JSX provides an easy way to write applications in React.
Typically React developers use JSX to give instructions on what the element should look like, set up events, and customize the content. However, sometimes it’s necessary to call React.createElement()
directly.
the React.createElement()
Method Arguments in React
The method accepts three arguments: the element type, props, and children.
The first argument can be a name of one of the conventional HTML elements as a string (for example, div
) or a custom component (for example, Contact
).
The second argument represents the component’s props
, and it can be either a single object with a key-value pair or an array of similar objects.
The last argument(s) represents children
, which can be any value you want from a parent component to pass to the child.
Let’s see how to use React.createElement()
method in practice.
export default function App() {
return (
<div className="App">
{React.createElement("h1", null, "Some text,", " more words")}
</div>
);
}
We create a simple heading element h1
in this example, which we know from HTML. We pass null
as props because we don’t need props.
The last two arguments are both strings. If you look on CodeSandbox, you’ll notice that these strings automatically become the content between our h1
element.
You can access these arguments by reading props.children
within the child component.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn