How to Import JavaScript File Into ReactJS
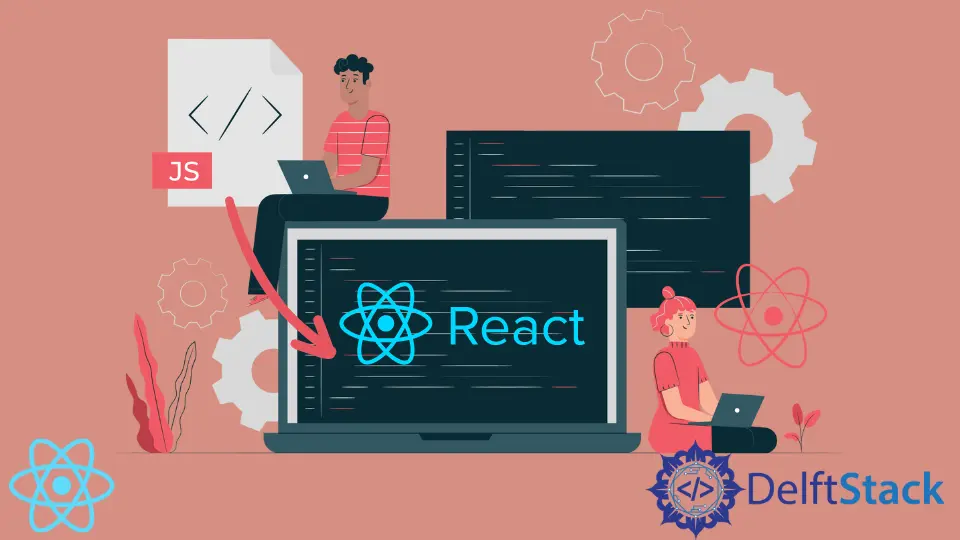
This tutorial demonstrates the use of import/export
(ES6 module) and default
exports to import JavaScript files into ReactJS.
Import JavaScript File Into ReactJS
Using the native ES6 module
system, we can include a JavaScript file in another JavaScript file. It enables us to create code modularity
and code sharing
between various JavaScript files.
Several techniques include a JS file, such as JavaScript include
and Node JS require
.
The native ES6 module
system offers a mechanism to split the code into several files and directories while guaranteeing that all the individual pieces of code may communicate.
We can import a JS file in one of two ways in React:
- Use
import/export
| ES6 module - Use
Default
Exports
Use import/export
(ES6 Module) to Import JS File Into ReactJS
Let’s begin by importing and exporting using the ES6 method. But, first, create the method and constants listed below in a file called helper.js
.
export function greetFunction(name) {
return `Hello, ${name}`;
}
export const appMessage = 'Hello World! Welcome to DefltStack!';
You’ll see that we declared that the function and variable might be used by other files using the export
keyword before them.
Syntax:
import {object1, object2, ...} from 'filename.js'
Create another file, name it main.js
, and then paste the following code inside of it:
import {appMessage, greetFunction} from './helper.js';
export default function App() {
const greetDelftStack = greetFunction('DelftStack');
return (
<Fragment>
{appMessage}
<br>
{greetDelftStack}
</Fragment>
)
}
Output:
Hello World! Welcome to DefltStack!
Hello, DelftStack
By defining greetFunction
and appMessage
within curly braces in the first line, we are importing them from helper.js
. We can now use the imported objects because they are defined in the same file after this line.
The output of both objects was then console-logged.
Use Default
Exports to Import JS File Into ReactJS
The default
keyword automatically allows us to export one object from a file. Why does this matter? Let’s look at an illustration. Add default
before the function greetFunction(name)
in helper.js
to make it a default
export:
export default function greetFunction(name) {
return `Hello, ${name}`;
}
You can now import it into the main.js
similar to this:
import anyFnName from './helper.js';
export default function App() {
const greetDelftStack = anyFnName('DelftStack');
return (
<main>
{greetDelftStack}
</main>
)
}
Output:
Hello, DelftStack
The exact process will operate as before. AnyFnName
is imported from helper.js
during the default
export. Due to the absence of anyFnName
in helper.js
, the default
export is greetFunction()
, in this case is exported as random name.
You can find the demo for these example codes here and practice. But, first, let’s talk about some crucial ES6 module-related aspects that users should keep in mind when utilizing them:
- The curly braces must be removed when importing
default
exports. An error stating that no such export exists would have been generated. - Multiple exports may be present in one file. However, a file can only have one
default
export. - A standard export cannot be imported using an alias name. Therefore, we must use the same name when importing a typical export.
- We can blend
default
and regular exports. For example, for named or standard exports,use {}
andleave {}
for the default export. - Using the
*
, we can import a module’s contents. The module will then be used as anamespace
to access all exports. - We can export all the objects simultaneously at the file’s end.
So, you can choose any of the above approaches to import JavaScript files into ReactJS.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn