Bootstrap Dropdown in React
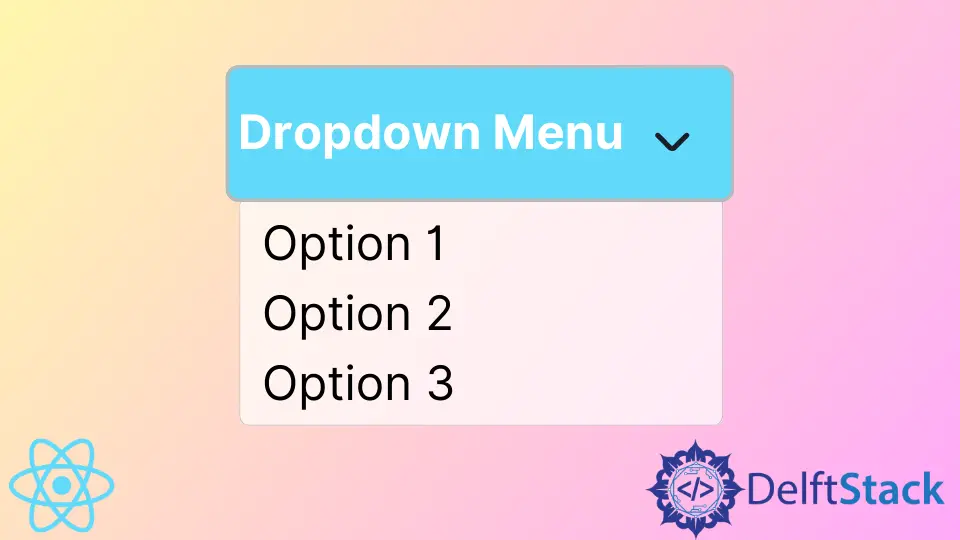
We will demonstrate how to create a Bootstrap dropdown in React and pass values from a list to a Bootstrap dropdown.
Bootstrap Dropdown in React
To create a dropdown in Bootstrap, we must install two libraries, react-bootstrap
and bootstrap
. We can easily install them using CLI.
# CLI
npm i react-bootstrap
npm i bootstrap
After installing these libraries, we must import bootstrap CSS
in index.js
.
# react
import "bootstrap/dist/css/bootstrap.min.css";
After importing bootstrap CSS
in index.js
file. We must import Dropdown
in App.js
.
# react
import Dropdown from "react-bootstrap/Dropdown";
Now, we will create a dropdown using Bootstrap.
# react
<div className="App">
<Dropdown onSelect={this.change}>
<Dropdown.Toggle variant="info" id="dropdown-basic">
Dropdown Menu
</Dropdown.Toggle>
<Dropdown.Menu>
<Dropdown.Item value="1">Option 1</Dropdown.Item>
<Dropdown.Item value="2">Option 2</Dropdown.Item>
<Dropdown.Item value="3">Option 3</Dropdown.Item>
<Dropdown.Item value="4">Option 4</Dropdown.Item>
</Dropdown.Menu>
</Dropdown>
</div>
Output:
We can even change its color by changing the variant
to danger
.
Now we will discuss how to add values from a list to a dropdown. Let’s create a list in App.js
.
# react
const list = [
{
Title: "Books",
Id: "1"
},
{
Title: "Movies",
Id: "2"
},
{
Title: "Comics",
Id: "3"
}
];
Now, let’s pass this list to the dropdown. First, we need to map this list. So, our code in App.js
will look like this.
# react
<div className="App">
<Dropdown onSelect={this.change}>
<Dropdown.Toggle variant="info" id="dropdown-basic">
Dropdown Menu
</Dropdown.Toggle>
<Dropdown.Menu>
{ list.map((item) => {
return(
<Dropdown.Item value={ item.Id }>{ item.Title }</Dropdown.Item>
)
}) }
</Dropdown.Menu>
</Dropdown>
</div>
It will pass the values and keys from our list like this.
Output:
In this way, we can create a Bootstrap dropdown in React.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn