How to Omit NA in R
-
Use
na.omit()
to RemoveNA
Values From a Vector in R -
Use
na.omit()
to Remove Rows WithNA
Values From a Data Frame in R -
Use
na.omit()
to Remove Rows WithNA
Values From Specific Columns in R
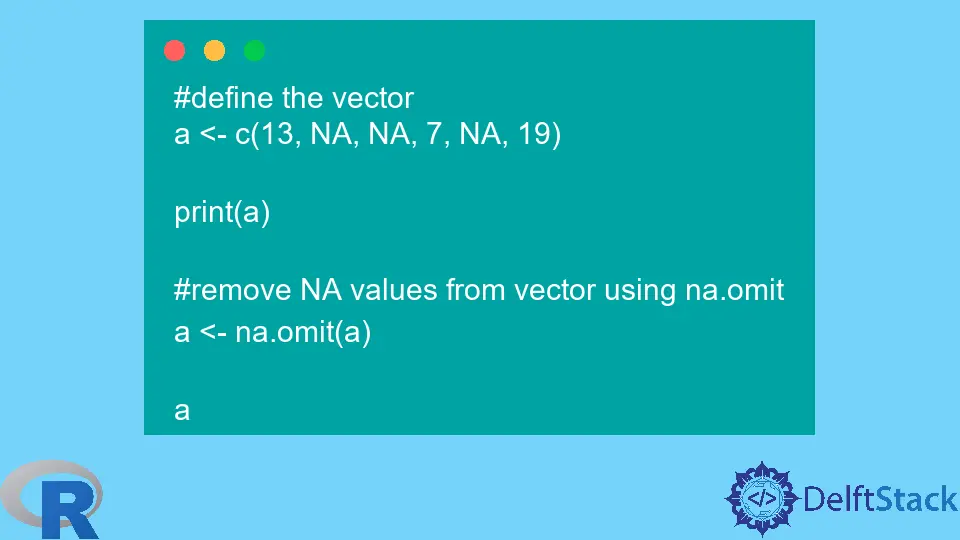
The na.omit()
method from R omits all unnecessary values from a data frame in R. NA
denotes these values.
This tutorial demonstrates how to use na.omit
in R.
Use na.omit()
to Remove NA
Values From a Vector in R
na.omit()
can remove NA
values from a vector; see example.
#define the vector
a <- c(13, NA, NA, 7, NA, 19)
print(a)
#remove NA values from vector using na.omit
a <- na.omit(a)
a
The code first prints the vector with NA
values and then omits the NA
values. See output:
[1] 13 NA NA 7 NA 19
[1] 13 7 19
attr(,"na.action")
[1] 2 3 5
attr(,"class")
[1] "omit"
The output for na.omit
is the remaining values and the index numbers of NA
values; we can get the simple remaining values by using the code below.
#define the vector
a <- c(13, NA, NA, 7, NA, 19)
print(a)
#remove NA values from vector using na.omit, as.numeric
a <- as.numeric(na.omit(a))
a
The output will be simple.
[1] 13 NA NA 7 NA 19
[1] 13 7 19
Use na.omit()
to Remove Rows With NA
Values From a Data Frame in R
na.omit()
can remove the rows with NA
values from a data frame. See example:
Delftstack = data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c(NA, 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, NA, 104, NA),
Designation=c('CEO', 'Project Manager', NA , 'Junior Dev', 'Intern'))
# Data frame before omit
Delftstack
# Use omit
Delftstack <- na.omit(Delftstack)
# Data frame after omit
Delftstack
The code above will remove all the rows with NA
values from the given data frame. See output:
Name LastName Id Designation
1 Jack <NA> 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler NA <NA>
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro NA Intern
Name LastName Id Designation
2 John Cena 102 Project Manager
4 Michelle McCool 104 Junior Dev
Use na.omit()
to Remove Rows With NA
Values From Specific Columns in R
na.omit()
can be specified based on the columns; we can pass the column name to remove rows with NA
values based on that specific column. See example:
Delftstack = data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c(NA, 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, NA, 104, NA),
Designation=c('CEO', 'Project Manager', NA , 'Junior Dev', 'Intern'))
# Data frame before omit
Delftstack
# Use omit
Delftstack <- Delftstack[!(is.na(Delftstack$Id)), ]
# Data frame after omit
Delftstack
The code removes the rows with NA
values based on the Id
column. See output:
Name LastName Id Designation
1 Jack <NA> 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler NA <NA>
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro NA Intern
Name LastName Id Designation
1 Jack <NA> 101 CEO
2 John Cena 102 Project Manager
4 Michelle McCool 104 Junior Dev
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook