How to Find Row Number in R
-
Find Row Numbers for a Value in R Using the
which()
Function -
Find Row Numbers for a Value in R Using the
match()
Function - Conclusion
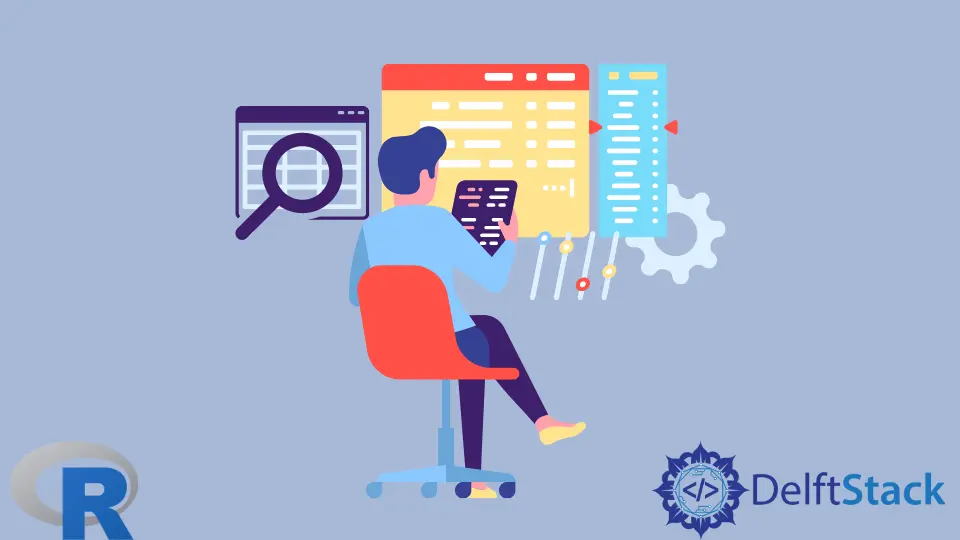
R is a powerful programming language and environment for statistical computing and graphics.
When working with large datasets, it’s common to need to locate the position or row number of a specific value within a particular column. Whether you’re a data analyst, statistician, or researcher, knowing how to find a row number for a value in R is a significant skill.
In this article, we’ll explore various methods to achieve this task. We’ll delve into the syntax and applications of the which()
and match()
functions, offering comprehensive insights into how they can be effectively used to find row numbers for specific values.
Find Row Numbers for a Value in R Using the which()
Function
The which()
function in R is a powerful tool for locating the row number associated with a specific value within a data frame.
The which()
function in R is designed to identify the indices or positions of elements in a logical vector that are TRUE
. This function is particularly useful when searching for specific conditions within a data frame.
The basic syntax of the which()
function is as follows:
which(logical_vector, arr.ind = FALSE, useNames = TRUE)
Where:
logical_vector
: A logical vector or condition that is evaluated to determine the positions ofTRUE
values.arr.ind
: An optional argument, if set toTRUE
, the result will be an array of indices rather than a vector.useNames
: An optional argument, if set toFALSE
, the names of the logical vector are ignored during the evaluation.
The which()
function returns the row number where the condition is met, and if not found, it returns 0
.
Code Example 1: Find Row Number Based on Designation
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
cat("The Original DataFrame:\n")
print(Delftstack)
# Find the row number where Designation is 'Senior Dev'
cat("DataFrame Row Number Where Designation value is Senior Dev:\n")
seniorDevRow <- which(Delftstack$Designation == "Senior Dev")
print(seniorDevRow)
In this example, we create a data frame named Delftstack
containing information about individuals, such as their names, last names, IDs, and designations. The goal is to find the row number where the designation is Senior Dev
using the which()
function.
We first display the original data frame using print()
. Then, we apply the which()
function to the Designation
column of the data frame, seeking rows where the designation is Senior Dev
.
The result, stored in the variable seniorDevRow
, is then printed.
Output:
[1] "The Original DataFrame:"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
[1] "DataFrame Row Number Where Designation value is Senior Dev"
[1] 3
The output shows that the Senior Dev
designation is located in the third row of the data frame.
Code Example 2: Find Row Number Based on Row Name
DelftstackNew <- data.frame(Column1 = 1:20, Column2 = 20:1, Column3 = 9)
numberofrows <- nrow(DelftstackNew)
rownames(DelftstackNew) <- LETTERS[1:numberofrows]
cat("The Original DataFrame:\n")
print(DelftstackNew)
# Find the row number for the row named 'S'
cat("DataFrame Row Number Where value is S:\n")
rowS <- which(
rownames(DelftstackNew) ==
"S"
)
print(rowS)
In this example, a new data frame, DelftstackNew
, is created with columns named Column1
, Column2
, and Column3
. Row names are assigned using uppercase letters from A
to T
.
The which()
function is then applied to find the row number where the row name is S
within the row names of the data frame. The result, stored in the variable rowS
, is printed.
Output:
[1] "The Original DataFrame: "
Column1 Column2 Column3
A 1 20 9
B 2 19 9
C 3 18 9
D 4 17 9
E 5 16 9
F 6 15 9
G 7 14 9
H 8 13 9
I 9 12 9
J 10 11 9
K 11 10 9
L 12 9 9
M 13 8 9
N 14 7 9
O 15 6 9
P 16 5 9
Q 17 4 9
R 18 3 9
S 19 2 9
T 20 1 9
[1] "DataFrame Row Number Where value is S:"
[1] 19
This illustrates how which()
can be utilized to locate row numbers based on assigned row names.
Code Example 3: Find Multiple Row Numbers Based on Condition
grades <- data.frame(
Student = c("Alice", "Bob", "Charlie", "David", "Eve"),
Math = c(90, 85, 78, 92, 87),
English = c(88, 90, 75, 94, 80),
History = c(82, 88, 70, 90, 85)
)
cat("The Original DataFrame:\n")
print(grades)
# Find row numbers where the Math grade is greater than 80
cat("Row Numbers where Math grade is greater than 80:\n")
mathAbove80 <- which(grades$Math > 80)
print(mathAbove80)
Here, a data frame named grades
is created to represent student grades in different subjects. The code begins by displaying the original data frame.
The objective is to find the row numbers where the Math grade is greater than 80
. The which()
function is applied to the Math
column, searching for the condition where the Math grade exceeds 80
.
The resulting row numbers are stored in the variable mathAbove80
, and they are printed to the console.
Output:
[1] "The Original DataFrame:"
Student Math English History
1 Alice 90 88 82
2 Bob 85 90 88
3 Charlie 78 75 70
4 David 92 94 90
5 Eve 87 80 85
[1] "Row Numbers where Math grade is greater than 80:"
[1] 1 2 4 5
This example demonstrates how which()
can be applied to identify multiple row numbers based on a specific condition in a data frame.
Code Example 4: Find the Row Number for a Specific Value in a Vector
gradesMath <- c(90, 85, 78, 92, 87)
cat("The Original Vector:\n")
print(gradesMath)
# Find the row number where the grade is 78
cat("Row Number where the grade is 78:\n")
grade78Row <- which(gradesMath == 78)
print(grade78Row)
In this final code example, a vector named gradesMath
is created to represent Math grades. The original vector is displayed, and the goal is to find the row number where the grade is equal to 78
.
The which()
function is applied to the vector, searching for the condition where the grade is equal to 78
. The resulting row number is stored in the variable grade78Row
, and it is printed to the console.
Output:
[1] "The Original Vector:"
[1] 90 85 78 92 87
[1] "Row Number where the grade is 78:"
[1] 3
The output shows the position of the value 78
in the vector.
Find Row Numbers for a Value in R Using the match()
Function
In addition to the which()
function, the match()
function in R provides another method to find the row number for a specific value in a data frame or vector.
The match()
function in R is designed to locate the positions of values within a vector or other data structures. Unlike which()
, which primarily deals with logical conditions, match()
directly compares values.
This makes it particularly useful for finding row numbers associated with specific values.
The basic syntax of the match()
function is as follows:
match(x, table, nomatch = NA, incomparables = NULL)
Where:
x
: A vector of values to be matched.table
: A vector or a list of values to be matched against.nomatch
: A value to be returned when no match is found (default isNA
).incomparables
: A vector of values that should be treated as ’not comparable'.
The match()
function returns the position of the first occurrence of the value in the vector. If the value is not found, it returns NA
.
Code Example 1: Find Row Number Based on Designation Using match()
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
cat("The Original DataFrame:\n")
print(Delftstack)
# Find the row number where Designation is 'Senior Dev' using match()
cat("DataFrame Row Number Where Designation value is Senior Dev:\n")
seniorDevRowMatch <- match("Senior Dev", Delftstack$Designation)
print(seniorDevRowMatch)
In this example, a data frame named Delftstack
is created, and the original data frame is displayed. The goal is to find the row number where the designation is Senior Dev
using the match()
function.
The match()
function is applied to the Designation
column, searching for the position of the value Senior Dev
. The resulting row number is stored in the variable seniorDevRowMatch
, and it is printed to the console.
Output:
The Original DataFrame:
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
DataFrame Row Number Where Designation value is Senior Dev:
[1] 3
Code Example 2: Find the Row Number for a Specific Value in a Vector Using match()
gradesMath <- c(90, 85, 78, 92, 87)
cat("The Original Vector:\n")
print(gradesMath)
# Find row number where the grade is 78 using match()
cat("Row Number where the grade is 78 using match():\n")
grade78RowMatch <- match(78, gradesMath)
print(grade78RowMatch)
In this example, a vector named gradesMath
representing Math grades is created, and the original vector is displayed. The objective is to find the row number where the grade is equal to 78
using the match()
function.
The match()
function is applied to the vector, searching for the position of the value 78
. The resulting row number is stored in the variable grade78RowMatch
, and it is printed to the console.
Output:
[1] "The Original Vector:"
[1] 90 85 78 92 87
[1] "Row Number where the grade is 78 using match():"
[1] 3
Code Example 3: Find Row Number Based on Row Name Using match()
DelftstackNew <- data.frame(Column1 = 1:20, Column2 = 20:1, Column3 = 9)
numberofrows <- nrow(DelftstackNew)
rownames(DelftstackNew) <- LETTERS[1:numberofrows]
cat("The Original DataFrame:\n")
print(DelftstackNew)
# Find the row number for the row named 'S' using match()
cat("DataFrame Row Number Where value is S using match():\n")
rowSMatch <- match("S", rownames(DelftstackNew))
print(rowSMatch)
In this example, a new data frame named DelftstackNew
is created with three columns. Row names are assigned using uppercase letters from A
to T
.
The original data frame is displayed, and the goal is to find the row number where the row name is S
using the match()
function. The match()
function is applied to the row names, searching for the position of the value S
.
The resulting row number is stored in the variable rowSMatch
, and it is printed to the console.
Output:
[1] "The Original DataFrame:"
Column1 Column2 Column3
A 1 20 9
B 2 19 9
C 3 18 9
D 4 17 9
E 5 16 9
F 6 15 9
G 7 14 9
H 8 13 9
I 9 12 9
J 10 11 9
K 11 10 9
L 12 9 9
M 13 8 9
N 14 7 9
O 15 6 9
P 16 5 9
Q 17 4 9
R 18 3 9
S 19 2 9
T 20 1 9
[1] "DataFrame Row Number Where value is S using match():"
[1] 19
Code Example 4: Find Multiple Row Numbers for Specific Values in a Vector Using match()
gradesEnglish <- c(88, 90, 75, 94, 80)
cat("The Original English Grades Vector:\n")
print(gradesEnglish)
# Find row numbers where the grade is either 75 or 94 using match()
cat("Row Numbers where the grade is 75 or 94 using match():\n")
grades75and94RowsMatch <- match(
c(75, 94),
gradesEnglish
)
print(grades75and94RowsMatch)
In this example, a vector named gradesEnglish
representing English grades is created, and the original vector is displayed. The goal is to find the row numbers where the grade is either 75
or 94
using the match()
function.
The match()
function is applied to the vector, searching for the positions of the values 75
and 94
. The resulting row numbers are stored in the variable grades75and94RowsMatch
, and they are printed to the console.
Output:
[1] "The Original English Grades Vector:"
[1] 88 90 75 94 80
[1] "Row Numbers where the grade is 75 or 94 using match():"
[1] 3 4
These examples show the versatility of the match()
function for finding row numbers based on specific values, whether in row names, vectors, or data frames. The function provides a convenient way to locate positions corresponding to given values.
Conclusion
The process of finding a row number for a specific value in R is a fundamental task in data manipulation. Two commonly used functions for this purpose are which()
and match()
. The which()
function is versatile and can be applied to data frames, enabling users to identify row numbers based on conditions.
On the other hand, the match()
function provides a concise way to find the position of a value in a vector, column, or row name. Both functions offer valuable tools for different scenarios, and the choice between them depends on the specific requirements of the analysis.
The presented examples demonstrated the practical application of these functions using diverse datasets. Whether searching for a specific designation in a data frame, row names in a data frame, or multiple values in a vector, the combination of which()
and match()
functions showcase their flexibility and efficiency in addressing various use cases.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook