How to Count Number of Observations in R
-
How to Count Number of Observations in R Using the
with()
andsum()
Functions -
How to Count Number of Observations in R Using the
nrow()
Function -
How to Find Number of Observations in R Using
dplyr
Package’scount()
Function - Conclusion
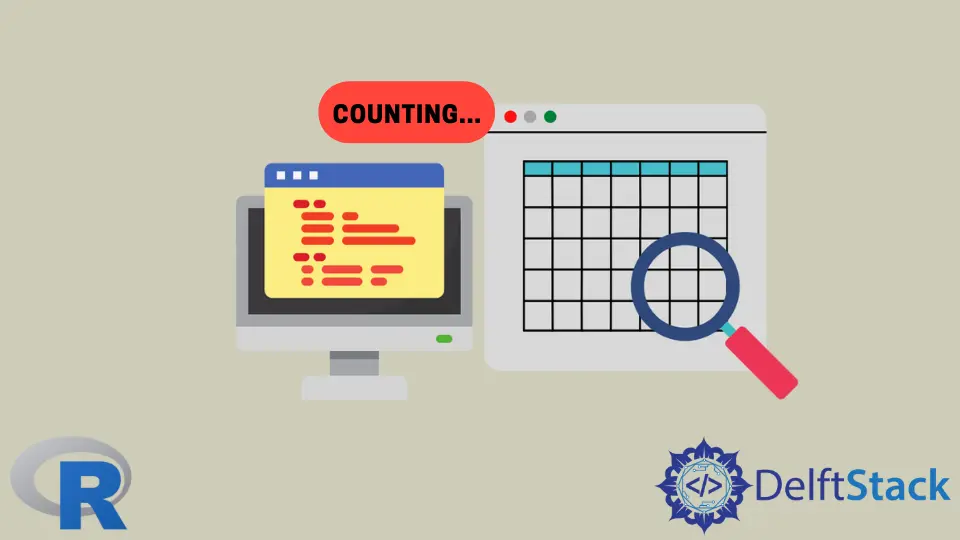
Counting the number of observations is a fundamental step in the analysis of datasets in the R programming language. Whether you are exploring the characteristics of a dataset, preparing for statistical analyses, or cleaning your data, understanding how to count observations efficiently is important.
In this article, we will delve into various methods and functions available in R to count the number of observations, each catering to different scenarios and preferences. From base R functions to specialized packages like dplyr
, we will explore syntax and examples to provide you with the knowledge needed to confidently handle the task of counting observations in your R projects.
How to Count Number of Observations in R Using the with()
and sum()
Functions
To determine the number of observations (rows) in a particular data frame, we can use an approach that leverages the with()
and sum()
functions.
The with()
function in R is used to evaluate an expression within the context of a specified environment. This can simplify the code by allowing you to refer to variables directly without the need to repeatedly prefix them with the data frame name.
The sum()
function, on the other hand, computes the sum of a set of values. When applied to logical vectors, it counts the number of TRUE
values.
Here’s how we can use this approach:
n_obs <- sum(with(data_frame, 1))
Where:
data_frame
: Replace this with the name of your data frame.with(data_frame, 1)
: Thewith()
function is used to create a temporary environment where the expression1
is evaluated within the context of the specified data frame. The result is a logical vector ofTRUE
values, one for each observation.sum()
: Finally, thesum()
function counts the number ofTRUE
values, giving us the total number of observations.
Here’s an example code to better understand how it works.
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28)
)
# Counting observations using with() and sum()
n_obs <- sum(with(your_data_frame, 1))
cat("Number of Observations:", n_obs, "\n")
In the provided example, we start by creating a sample data frame named your_data_frame
. The with()
function is then used to evaluate the expression 1
within the context of this data frame.
This results in a logical vector of TRUE
values, where each TRUE
corresponds to an observation in the data frame.
Next, the sum()
function is applied to this logical vector, effectively counting the number of TRUE
values. The result, stored in the variable n_obs
, represents the total number of observations in the data frame.
Finally, the output statement uses cat()
to display the number of observations clearly and concisely.
Code Output:
Number of Observations: 1
Let’s consider another scenario where you want to count the number of observations in an R data frame based on a specific condition using the with()
and sum()
functions.
sample_data <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28),
Passed_Exam = c(TRUE, FALSE, TRUE, TRUE, FALSE)
)
condition <- with(sample_data, Passed_Exam == TRUE)
count_passed <- sum(condition)
cat("Number of observations where Passed_Exam is TRUE:", count_passed, "\n")
In this example, we have a data frame named sample_data
with a column Passed_Exam
indicating whether a person passed an exam (TRUE
) or not (FALSE
).
We use the with()
function to evaluate the condition Passed_Exam == TRUE
within the context of the sample_data
data frame. The result is a logical vector, which is then passed to the sum()
function.
sum()
counts the number of TRUE
values, providing the total number of observations where Passed_Exam
is TRUE
.
Code Output:
Number of observations where Passed_Exam is TRUE: 3
How to Count Number of Observations in R Using the nrow()
Function
While the combination of with()
and sum()
functions provides a flexible approach, another straightforward method for counting observations in R involves the use of the nrow()
function. The nrow()
function directly returns the number of rows in a data frame or matrix, eliminating the need for additional logical manipulations.
Here’s the syntax of the nrow()
function:
n_obs <- nrow(data_frame)
Here, data_frame
is the name of your data frame.
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28)
)
n_obs <- nrow(your_data_frame)
cat("Number of Observations:", n_obs, "\n")
In this example, the nrow()
function is applied directly to the data frame your_data_frame
, returning the total number of rows. The result is stored in the variable n_obs
, representing the count of observations.
This method is particularly straightforward because nrow()
eliminates the need for additional logical operations or temporary variables. It directly provides the count of observations, making the code concise and easy to understand.
Code Output:
Number of Observations: 5
Let’s consider another example where we count the number of observations in an R data frame based on a specific condition using the nrow()
function.
sample_data <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28),
Passed_Exam = c(TRUE, FALSE, TRUE, TRUE, FALSE)
)
condition <- sample_data$Passed_Exam == TRUE
count_passed <- nrow(sample_data[condition, , drop = FALSE])
cat("Number of observations where Passed_Exam is TRUE:", count_passed, "\n")
In this example, we have a data frame named sample_data
with a column Passed_Exam
indicating whether a person passed an exam (TRUE
) or not (FALSE
). We create a logical vector condition
to identify rows where Passed_Exam
is TRUE
.
We then use this condition to subset the data frame, and finally, the nrow()
function is applied to count the number of rows in the subset, providing the total number of observations where Passed_Exam
is TRUE
.
Code Output:
Number of observations where Passed_Exam is TRUE: 3
How to Find Number of Observations in R Using dplyr
Package’s count()
Function
In addition to the base R functions discussed earlier, the dplyr
package offers a powerful and intuitive method for counting observations using the count()
function. The count()
function in the dplyr
package is used to quickly count the occurrences of unique combinations of variables in a data frame.
The basic syntax of the count()
function is as follows:
count(data, ..., wt = NULL, sort = FALSE, name = "n", sort_desc = FALSE, drop = TRUE)
Here is a breakdown of the main arguments:
data
: The data frame, data frame extension, or lazy data frame to be used....
: Variables to group by. You can specify one or more variables here.wt
: An optional argument to specify a variable that contains weights for weighted counting.sort
: A logical value indicating whether the result should be sorted by frequency.name
: The name of the column to store the count values.sort_desc
: A logical value indicating whether to sort the result in descending order.drop
: A logical value specifying the handling of factor levels that don’t appear in the data. Ifdrop
isTRUE
, it will exclude counts for empty groups (levels of factors that don’t exist in the data). Ifdrop
isFALSE
, it will include counts for empty groups.
You can use the ...
argument to allow you to specify variables for group-wise counts. The optional wt
argument allows for weighted counting.
The result is a data frame containing the unique combinations and their corresponding counts. It will have the same groups as the input data frame, specifically the grouping variables specified in the ...
argument.
Example 1: Basic Usage
library(dplyr)
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28)
)
counted_data <- your_data_frame %>%
count(ID, Name, Age)
cat("Number of observations:\n")
print(counted_data)
In this example, we load the dplyr
library and use the count()
function directly on the data frame. The result, stored in counted_data
, is a data frame with columns representing the unique values in the original data frame and a count column n
indicating the frequency of each unique combination.
Code Output:
Number of observations:
ID Name Age n
1 1 Alice 25 1
2 2 Bob 30 1
3 3 Charlie 22 1
4 4 David 35 1
5 5 Eva 28 1
Example 2: Counting Based on a Specific Variable
library(dplyr)
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28)
)
counted_data <- count(your_data_frame, Name)
cat("Number of observations:\n")
print(counted_data)
In this example, we count observations based on the Name
variable. The count()
function is applied to the data frame, specifying the variable of interest.
The resulting data frame includes the unique names and their corresponding usage count.
Code Output:
Number of observations:
Name n
1 Alice 1
2 Bob 1
3 Charlie 1
4 David 1
5 Eva 1
Example 3: Counting Based on Multiple Variables
library(dplyr)
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28)
)
counted_data <- count(your_data_frame, Name, Age)
cat("Number of observations:\n")
print(counted_data)
Here, we extend the functionality by counting observations based on both the Name
and Age
variables. The resulting data frame provides counts for unique combinations of these variables.
Code Output:
Number of observations:
Name Age n
1 Alice 25 1
2 Bob 30 1
3 Charlie 22 1
4 David 35 1
5 Eva 28 1
Example 4: Weighted Count
library(dplyr)
your_data_frame <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
Age = c(25, 30, 22, 35, 28),
Weight = c(0.8, 1.2, 0.5, 1.5, 1)
)
# Perform weighted counts based on the 'Name' variable
weighted_count <- count(your_data_frame, wt = Weight)
print(weighted_count)
In this example, we introduce a new variable named Weight
to the data frame, representing the weights assigned to each observation. The count()
function is then applied to the data frame, specifying the weight variable using the wt
argument.
The resulting data frame, stored in weighted_count
, includes the unique values along with their weighted counts.
Code Output:
# A tibble: 5 × 5
# Groups: ID, Name, Age [5]
ID Name Age Weight n
<dbl> <chr> <dbl> <dbl> <dbl>
1 1 Alice 25 0.8 0.8
2 2 Bob 30 1.2 1.2
3 3 Charlie 22 0.5 0.5
4 4 David 35 1.5 1.5
5 5 Eva 28 1 1
In this output, the n
column represents the weighted count of observations.
Note that the statement:
df %>%
count(a, b)
is roughly equivalent to:
df %>%
group_by(a, b) %>%
summarise(n = n())
The count()
function in the dplyr
package is designed to simplify the process of grouping by specific variables and summarizing the counts. Choose the example that suits your analysis needs and modify the code accordingly for your dataset.
Conclusion
Counting the number of observations in R is a fundamental task in data analysis, and several methods can be employed based on the specific requirements of your analysis. We explored three distinct approaches in this article: the use of base R functions such as with()
and sum()
, the nrow()
function, and the dplyr
package’s count()
function.
Whether you prefer concise base R syntax, direct row counting, or the advanced features of dplyr
, each method provides an efficient way to obtain the total number of observations in your dataset. Choose the method that aligns with your analysis needs and enhances the clarity and readability of your code.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn