How to Fix TypeError: Iteration Over Non-Sequence
-
Recreate
TypeError: iteration over non-sequence
in Python -
Iterate Over a List to Fix
TypeError: iteration over non-sequence
in Python -
Use
__iter__
to FixTypeError: iteration over non-sequence
Error in Python
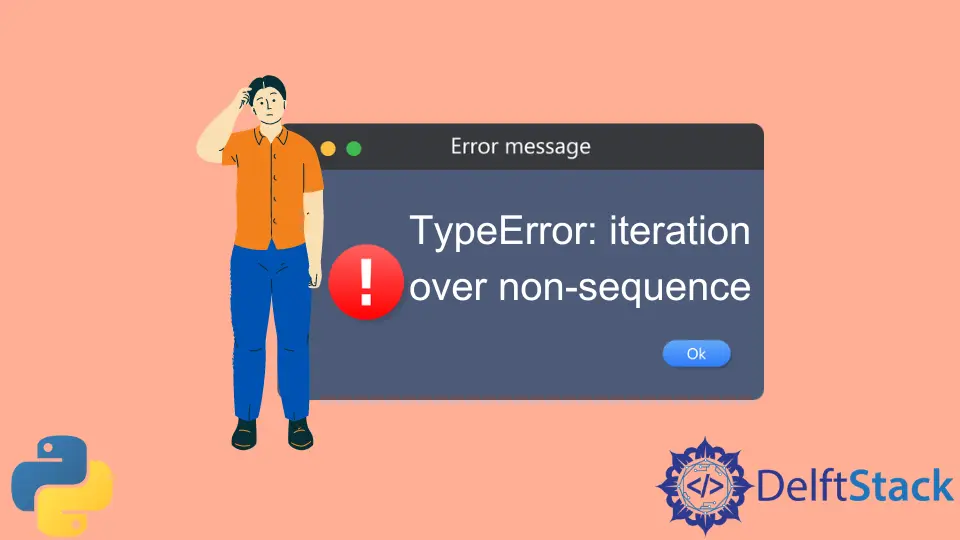
The TypeError
is raised in Python when the function or operation is applied to an object of an inappropriate type. For instance, adding integer and string objects returns TypeError
.
The error TypeError: iteration over non-sequence
occurs when you try to iterate over an object which is not iterable. This tutorial will teach you to fix TypeError: iteration over non-sequence
in Python.
Recreate TypeError: iteration over non-sequence
in Python
Now you have understood the cause of TypeError
, let’s recreate the iteration over non-sequence
error in Python. Running the following script in Python 2 gives TypeError: iteration over non-sequence
.
class Book:
def __init__(self, name, author):
self.name = name
self.author = author
class Collection:
def __init__(self):
self.read = []
def add_book(self, name, author):
self.read.append(Book(name, author))
if __name__ == "__main__":
Books = Collection()
Books.add_book("Romeo and Juliet", "William Shakespeare")
Books.add_book("To Kill a Mockingbird", "Harper Lee")
for book in Books:
print(book.name)
Here, we are trying to iterate over the object Books
instead of the list read
inside it. As a result, it gives TypeError: iteration over non-sequence
in Python 2.
Output:
Traceback (most recent call last):
File "Main.py", line 17, in <module>
for book in Books:
TypeError: iteration over non-sequence
In Python 3, you will get the following output.
Traceback (most recent call last):
File "Main.py", line 17, in <module>
for book in Books:
TypeError: 'Collection' object is not iterable
Iterate Over a List to Fix TypeError: iteration over non-sequence
in Python
Since Books
is an object, you have to iterate over the list inside the object Books
. In this case, it will be for book in Books.read
.
class Book:
def __init__(self, name, author):
self.name = name
self.author = author
class Collection:
def __init__(self):
self.read = []
def add_book(self, name, author):
self.read.append(Book(name, author))
if __name__ == "__main__":
Books = Collection()
Books.add_book("Romeo and Juliet", "William Shakespeare")
Books.add_book("To Kill a Mockingbird", "Harper Lee")
for book in Books.read:
print(book.name)
Output:
Romeo and Juliet
To Kill a Mockingbird
Use __iter__
to Fix TypeError: iteration over non-sequence
Error in Python
The __iter__
method is used when a container requires an iterator. It should return the iterator object that can iterate over all the objects in the container.
Books
is an instance of Collection
. You can use the __iter__
method to iterate over the object Books
.
class Collection:
def __iter__(self):
return iter(self.read)
The following block contains the complete script.
class Book:
def __init__(self, name, author):
self.name = name
self.author = author
class Collection:
def __init__(self):
self.read = []
def add_book(self, name, author):
self.read.append(Book(name, author))
def __iter__(self):
return iter(self.read)
if __name__ == "__main__":
Books = Collection()
Books.add_book("Romeo and Juliet", "William Shakespeare")
Books.add_book("To Kill a Mockingbird", "Harper Lee")
for book in Books:
print(book.name)
Output:
Romeo and Juliet
To Kill a Mockingbird
That’s how you can solve the iteration over non-sequence
error in Python. We hope you find these solutions helpful.
Related Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Solve the TypeError: An Integer Is Required in Python
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python