How to Run Python in Java Using Jython
- Run Python in Java Using Jython
- Install the Jython Library
- Link the Jython Library With an IDE
- Write Python Codes in Java and Compile It
- Python Code to Add Two Numbers Compiled in Java
- Python Code to Find Last Day of Month Compiled in Java
- Some Python Libraries That Do Not Run When Compiled in Java
- Conclusion
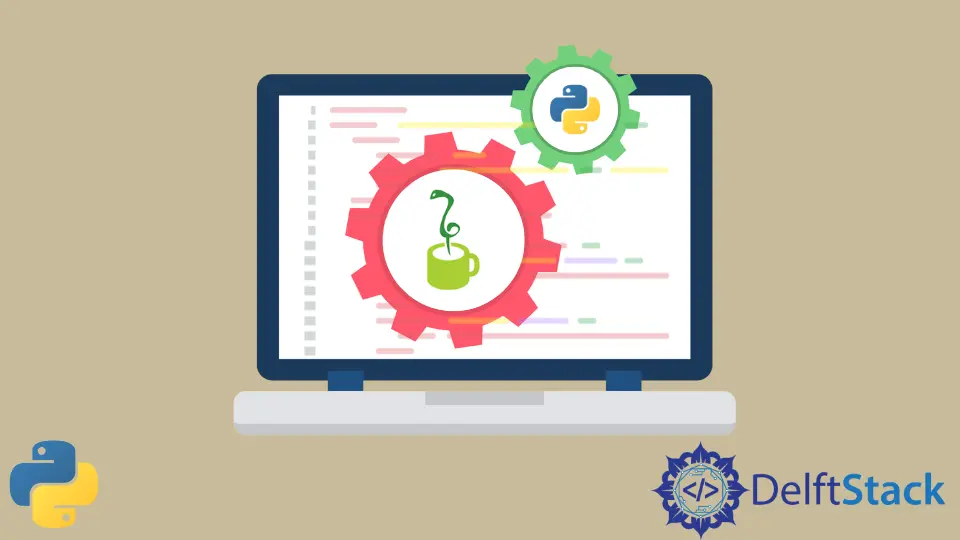
This article will thoroughly explain the steps to run python in Java programs using a Jython library. It would be a detailed step-by-step process with lots of images so that you don’t have to worry about “how he did that” moments.
Run Python in Java Using Jython
Running Python in Java requires three prerequisites.
- Python code.
- Jython Library.
- An IDE that can link the Jython Library.
Create the Python Code
Let’s take a simple python program that calls a method to add two numbers. Method add()
has two parameters and returns the sum of these two parameters and prints them.
def add(a, b):
s = a + b
return s
print(add(5, 6))
Running this program would print the sum of a
and b
.
Let’s look at another python program that uses an inbuilt library. This program uses the datetime
built-in library to find the last day of any given month.
import datetime
def last_day_of_month(date):
if date.month == 12:
return date.replace(day=31)
return date.replace(month=date.month + 1, day=1) - datetime.timedelta(days=1)
print(last_day_of_month(datetime.date(2022, 12, 25)))
In the last line of this program, it sends the date to the method last_day_of_month
, returns the last day of that month and gets printed.
Let’s also consider another program that uses a third-party library in the code.
from datetime import datetime
import pandas as pd
def check_month_end(date):
return date + pd.offsets.MonthEnd(0) # == date
print(check_month_end(datetime(2005, 2, 6)))
print(check_month_end(datetime(2004, 2, 29)))
This program uses pandas
inside the code. As some codes are prepared that will be used to run Python in Java programs, let’s learn how to install Jython.
Install the Jython Library
Jython makes it simple to call Python functions from Java code, provided that the Python code itself runs under Jython and doesn’t make use of any unsupported C-extensions.
It’s undoubtedly the simplest answer that can be found if that works for you. If not, the new Java6 interpreter can be utilized, which supports the org.python.util.PythonInterpreter
syntax.
The Jython library can be downloaded from here. The reader should download the standalone library version, which would help easily link it with an IDE.
The package will get installed on your machine. Make sure to note down the path where it gets downloaded.
In most machines, it can be found under “Downloads” inside the main library.
Link the Jython Library With an IDE
Using an IDE is the current norm, and the reader must switch to it if that’s not the case. This article will further demonstrate the steps in IntelliJ IDE, a very popular software for running java codes.
If the reader has never used IntelliJ before, worry not. Everything will be covered from scratch.
First, Install IntelliJ from here. After IntelliJ is installed and ready to use, a new project needs to be created.
A project will create a separate space for the files needed for demonstration in this article. Then the project will be linked to the Jython library so we can run python in java.
-
Create a New Project Inside IntelliJ IDE
Open the IntelliJ IDE, and click on ‘New Project’. If a project is already opened, go to File>New>Project.
-
Choose a Language
In this step, the right language must be chosen for the project. Select the Java Language with no extra packages (Both Groovy and Kotlin checkboxes must be kept unchecked).
The ‘Project SDK’ at the top of the dialog box shows the Java version. If nothing is displayed there, download a new one by clicking on a small triangle on the far right side.
-
Enable the Command Line Prompt
Tick the Checkbox to enable the ‘Command Line App’ and click Next.
-
Give a Name for the Project
Give an appropriate name for the project. In this case, the project is named
Python_in_Java
.Clicking on Finish will create a new project.
-
Add Jython Library to the Project Created in IntelliJ IDE
When a new project is created, it looks like the picture below. There is the
main
class file with some standard code.Now to link Jython to it, go to File>Project Structure. A new dialog box will pop up.
Inside the libraries section, click on the
+
icon and select ‘Java’ from the menu.Selecting Java will load a new dialog box that lets us browse files from the computer. Now it is time to remember the path of the standalone Jython file we downloaded.
Select the
.jar
file that we downloaded and click on Ok.Once again, click on Ok, and it will load the
Jython Standalone.jar
, and it will be displayed inside the library window. Lastly, click on Ok to finish the loading.As Jython is loaded to this library, we can now write codes and run python in java programs easily.
Write Python Codes in Java and Compile It
As the project is created and linked with the Jython library, Java codes can be written to run python in java.
The Java program uses the sub-function PythonInterpreter
from the Jython library to launch an internal interpreter inside the JVM(Java Virtual Machine).
Note that the Jython library only supports Python 2.7. Scripts that require newer Python versions can take support of micro web services that encapsulate python codes and run it inside other languages, but it can be a complex and lengthy process.
Jython is best suited for Java programmers who want to integrate the Jython libraries into their system to let end users create straightforward or complex scripts that extend the capabilities of the application.
Lastly, Python applications are 2–10 times shorter than their Java counterparts. This directly affects how productively programmers work.
Python and Java communicate well, allowing programmers to freely mix the two languages during product development and compiling.
First, need to create a Java program that runs Python in Java.
package com.company;
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec(""); // <--Python codes must be written inside this double quotes
}
}
Let’s understand what the above code does.
-
The first line of code is a standard IntelliJ header for Java programs.
-
The
PythonInterpreter
sub-package from Jython is imported in the second line. -
A
Main
Class is defined. If you’re new to Java, it must be known that every Java program has one class, at least inside it. -
Inside the class, the
main
method is defined. This program jumps directly to the main method without creating a function to reduce complexity. The class name is the same as theMain
method, but it is not always necessary.Any name can be a class name subject to some naming conventions.
-
An instance of
PythonInterpreter
is created aspythonInterpreter
. These instances are called objects in Java, and after their creation, they can be used inside main functions to call the imported functions. -
Lastly, the codes are written inside the
pythonInterpreter.exec();
syntax using double quotes. Our Python code is not interpreted, despite what thePythonInterpreter
class name suggests.The JVM executes Python programs in Jython. Therefore before running, they have first converted to Java bytecode.
Even though Jython is the Java version of Python, it might not have all the same sub-packages as native Python.
We now have enough understanding of how to use Jython. Let’s test the python codes we prepared at this article’s start.
Python Code to Add Two Numbers Compiled in Java
The Python codes are arranged automatically inside the double quotes when copy-pasted in the IntelliJ IDE.
package com.company;
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("def add(a, b):\n"
+ " s = a + b\n"
+ " return s\n"
+ "\n"
+ "print(add(5, 6))");
}
}
Output:
C:\java-1.8.0-openjdk-1.8.0.322-2.b06.dev.redhat.windows.x86_64\bin\java.exe ...
11
Python Code to Find Last Day of Month Compiled in Java
In the second program, we used a native Python library to find the last day of the month. As we know, Python Interpreter does not load all the native libraries, though the datetime
library is available.
package com.company;
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("import datetime\n"
+ "\n"
+ "\n"
+ "def last_day_of_month(date):\n"
+ " if date.month == 12:\n"
+ " return date.replace(day=31)\n"
+ " return date.replace(month=date.month + 1, day=1) - datetime.timedelta(days=1)\n"
+ "\n"
+ "\n"
+ "print(last_day_of_month(datetime.date(2022, 12, 25)))\n");
}
}
Output:
C:\java-1.8.0-openjdk-1.8.0.322-2.b06.dev.redhat.windows.x86_64\bin\java.exe ...
2022-12-31
Some Python Libraries That Do Not Run When Compiled in Java
When a program is run that uses a third-party library like Pandas, which Java cannot import, it displays an error.
package com.company;
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("from datetime import datetime\n"
+ "\n"
+ "import pandas as pd\n"
+ "\n"
+ "\n"
+ "def check_month_end(date):\n"
+ " return date + pd.offsets.MonthEnd(0) # == date\n"
+ "\n"
+ "\n"
+ "print(check_month_end(datetime(2005, 2, 6)))\n"
+ "\n"
+ "print(check_month_end(datetime(2004, 2, 29)))");
}
}
Output:
C:\java-1.8.0-openjdk-1.8.0.322-2.b06.dev.redhat.windows.x86_64\bin\java.exe ...
Exception in thread "main" Traceback (most recent call last):
File "<string>", line 3, in <module>
ImportError: No module named pandas
Let’s take another inbuilt library, syds
.
package com.company;
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("import syds");
}
}
Output:
C:\java-1.8.0-openjdk-1.8.0.322-2.b06.dev.redhat.windows.x86_64\bin\java.exe ...
Exception in thread "main" Traceback (most recent call last):
File "<string>", line 1, in <module>
ImportError: No module named syds
Conclusion
We have learned how to use Jython to run python in Java. The codes give a major understanding of how to use and when to use Jython.
The reader can easily create programs that run python in java. It is hoped that this article helped in your coding journey.