How to Generate a Random Value Between 0 and 1 in Python
-
Generate a Random Value Between 0 and 1 With the
random.randint()
Function in Python -
Generate a Random Value Between 0 and 1 With the
random.random()
Function in Python -
Generate a Random Value Between 0 and 1 With the
random.uniform()
Function in Python
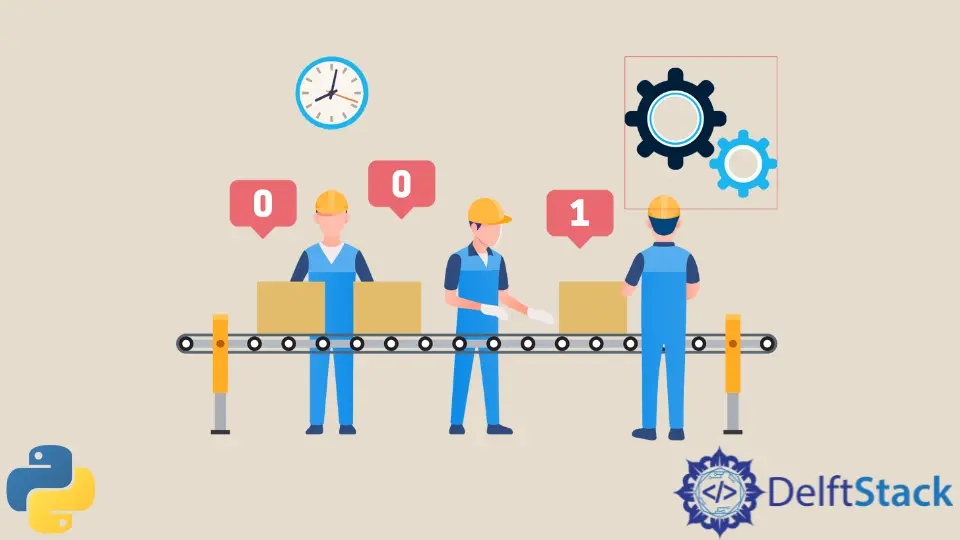
This tutorial will introduce methods to generate a random value between 0 and 1 in Python.
Generate a Random Value Between 0 and 1 With the random.randint()
Function in Python
The random
module provides many methods to generate random numbers in Python. The random.randint(x, y)
function generates a random integer between x
and y
. The following code example shows us how we can generate a random integer between 0 and 1 with the random.randint()
function in Python.
import random
for i in range(10):
print(random.randint(0, 1))
Output:
0
1
1
0
1
1
1
0
0
0
In the above code, we generate random integer values between 0 and 1 with the random.randint()
function in Python. This method is technically random, But it gives 0
output most of the time.
Generate a Random Value Between 0 and 1 With the random.random()
Function in Python
The random
module provides another method for generating a random number called the random.random()
function. The random.random()
function is used to generate random float values between 0 and 1. The following code example shows us how we can generate a random float value between 0 and 1 with the random.random()
function in Python.
import random
for i in range(10):
print(random.random())
Output:
0.825015043001995
0.9094437659082791
0.33868291714283916
0.5351032318837877
0.15110006300577983
0.35633216995962613
0.6230723865215685
0.35906777048890404
0.48989704057931327
0.5862369192498884
In the above code, we generate random float values between 0 and 1 with the random.random()
function in Python. As we can see, there is no repetition in the output.
Generate a Random Value Between 0 and 1 With the random.uniform()
Function in Python
Another method provided by the random
module to generate random float values is the random.uniform()
function. The random.uniform(x, y)
function generates random float values between x
and y
. The following code example shows us how we can generate a random float value between 0 and 1 with the random.uniform()
function in Python.
import random
for i in range(10):
print(random.uniform(0, 1))
Output:
0.013945221722152179
0.5017124648286838
0.877814513722702
0.2272981359909486
0.1752587757552797
0.8360499141768403
0.186039641814587
0.4962755696082156
0.5530128798215038
0.06186876002931674
In the above code, we generate random float values between 0 and 1 with the random.uniform()
function in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn