How to Validate IP Address in Python
- Validate an IP Address in Python
-
Use the
ipaddress
Module to Validate an IP Address in Python -
Use the
RegEx.search()
Method to Validate an IP Address in Python - Validate IP Address Manually in Python
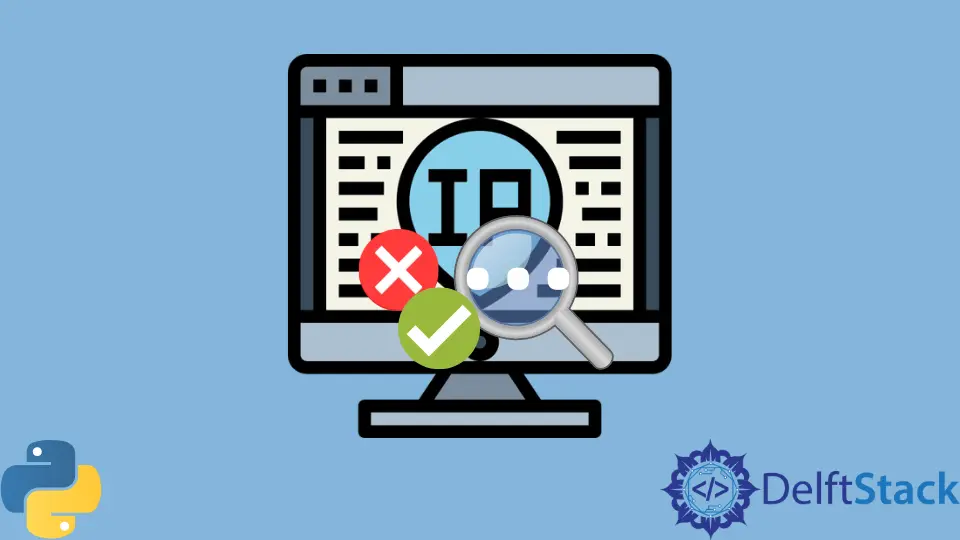
IP stands for internet protocol, a unique address assigned to every computer connected to the internet. There are two versions of IP addresses named IPv4
and IPv6
.
The IPv4 is represented in dotted-decimal notation and consists of four octets, with each octet containing a number ranging from 0 to 255. IPv6 addresses contain eight groups of four hexadecimal digits, each representing 16 bits.
Colons separate the groups (:
). In this guide, we will validate an IPv4 address.
Validate an IP Address in Python
Validating an IP address means checking if the input IP is valid or not.
For example:
Input: 192.168.52.124
Output: Valid IP address
Input: 656.1.2.3
Output: Invalid IP address
Use the ipaddress
Module to Validate an IP Address in Python
The ipaddress
is a module in Python used to check and classify IP addresses based on their types (IPv4 or IPv6). It also performs different operations such as arithmetic operations, comparison, etc.
This module has a method named ip_address()
that validates an IP address. This function takes a string IP as an input, and if it can successfully convert the string IP into an object of IPv4 or IPv6 address, it means that the input IP address is valid.
Else, it will raise a value error that the IP does not appear to be an IPv4 or IPv6 address. We imported the ipaddress
module in the following code and wrote the whole code in the try-except
block.
If the IP is valid, no exception will raise, and if not, the value error exception will be handled by the except
block.
Case 1: For Valid IP Address
# Python 3.x
import ipaddress
try:
ip = ipaddress.ip_address("192.168.1.1")
print("Valid IP Address")
except ValueError:
print("Invalid IP Address")
Output:
#Python 3.x
Valid IP Address
Case 2: For Invalid IP Address
# Python 3.x
import ipaddress
try:
ip = ipaddress.ip_address("992.168.1.1")
print("Valid IP Address")
except ValueError:
print("Invalid IP Address")
Output:
#Python 3.x
Invalid IP Address
Use the RegEx.search()
Method to Validate an IP Address in Python
Another way to validate an IP address is to match it with a predefined pattern of a valid IP address through a regular expression. We have validated an IP address in the following code by calling the search()
method of the re
module and passed the regex and IP as an argument.
This method returns True
if the IP is valid. Else, it returns False
.
Example Code:
# Python 3.x
import re
ip = "192.168.2.3"
regex = "^((25[0-5]|2[0-4][0-9]|1[0-9][0-9]|[1-9]?[0-9])\.){3}(25[0-5]|2[0-4][0-9]|1[0-9][0-9]|[1-9]?[0-9])$"
if re.search(regex, ip):
print("Valid IP Address")
else:
print("Invalid IP Address")
Output:
#Python 3.x
Valid IP Address
Validate IP Address Manually in Python
We can validate an IP address without any built-in library regular expression. We have made a function that validates the IP address by checking these three conditions in the following code.
The IP will be invalid if any following conditions are True
in the code.
- The number of octets is not four.
- Any octet contains anything other than an integer.
- Any number within an octet lies outside the range of 0-255.
Example Code:
# Python 3.x
def validate_ip_address(ip):
octects = ip.split(".")
if len(octects) != 4:
return "Invalid IP"
for number in octects:
if not isinstance(int(number), int):
return "Invalid IP"
if int(number) < 0 or int(number) > 255:
return "Invalid IP"
return "Valid IP"
validate_ip_address("192.168.-1.1")
Output:
#Python 3.x
'Invalid IP'
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn