How to Visualize Trees in Python
- Visualize Trees in Python
-
Install the
Graphviz
Package - Convert a Tree to a Dot File
- Convert a Dot File to an Image
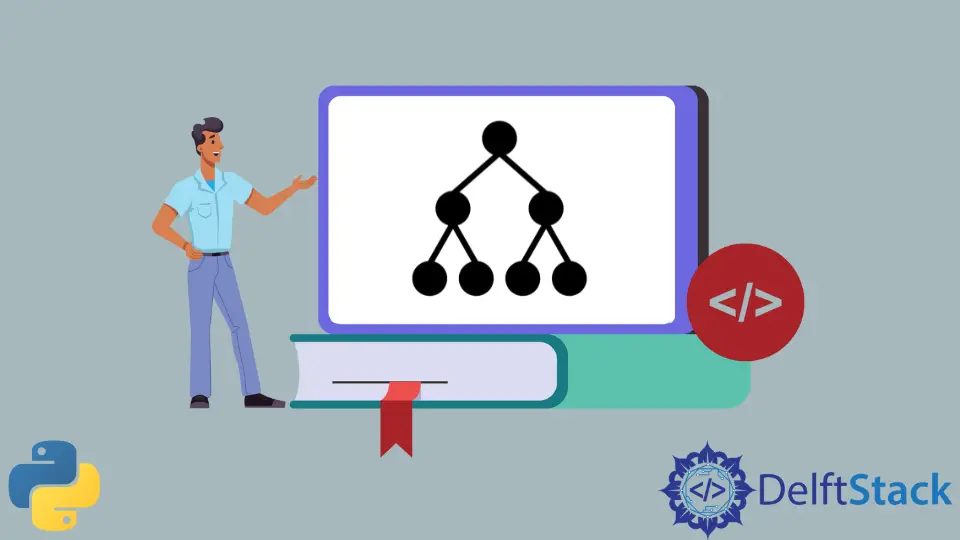
For many different reasons, decision trees are a common supervised learning technique. The advantages of decision trees include that we can use them for both classification and regression, that they don’t require feature scaling, and that decision trees are straightforward to read.
This article demonstrated Python’s Graphviz
to display decision trees.
Visualize Trees in Python
Graphviz
, or graph visualization, is open-source software that represents structural information as diagrams of abstract graphs and networks. For example, one use of Graphviz
in data science is visualizing decision trees.
Install the Graphviz
Package
Installing Graphviz
is often necessary to convert the dot file into an image file (PNG, JPG, SVG, etc.), which depends on your operating system and several other factors.
If you have received the error dot: command not found
, it is possible that you have not installed the dot
language as well.
To install Graphviz
, run the following.
-
Windows:
winget install graphviz
-
Mac:
brew install graphviz
-
Ubuntu:
sudo apt install graphviz
For more installation procedures like a manual download of executable files and other versions, you may go to the official Graphviz
documentation.
Once installed, verify if your Graphviz
installation has the dot.exe
file inside its installation directory, as we will need it later to generate a visualization of dot
files. Commonly, the default installation path is under C:\Program Files\Graphviz\bin
.
Convert a Tree to a Dot File
One of the requirements of Graphviz
is the tree in dot
format, but first, we need a sample tree. We may go to this article on how trees work in Python, or we can use the command below for the convenience of going through this article.
Example Code:
tree = "digraph G {Hello->World}"
open("sample.dot", "w").write(tree)
The snippet of code above writes a simple Hello->World
2-node tree structure to a sample.dot
file that sufficed our requirement. However, if we want a more complex tree, we can use this online tree maker that also conveniently lets us download it directly in dot
format.
Convert a Dot File to an Image
Now that we have our dot
file, we will need to run it inside Graphviz
using the dot
application. Earlier, we asked you to take note of our Graphviz
dot.exe
path as we will need it in this section.
Go to the dot.exe
path using the command cd
inside the command prompt.
cd C:\Program Files\Graphviz\bin
We are doing this because, most of the time, the Graphviz
installation doesn’t include dot
in our environment variables, so we can use the dot.exe
to run the following snippet of code.
# dot.exe -Tpng <path of the dot file> -o <destination path of the png file>
dot.exe -Tpng C:\PS\sample.dot -o C:\PS\sample.png
Once executed, this should yield the use of the visual representation of our tree in PNG
format.
For the complete information on dot
parameters, we may go to the dot
command official documentation.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn