How to Print Variable Name in Python
-
Print Variable Name With the
globals()
Function in Python - Print Variable Name With a Dictionary in Python
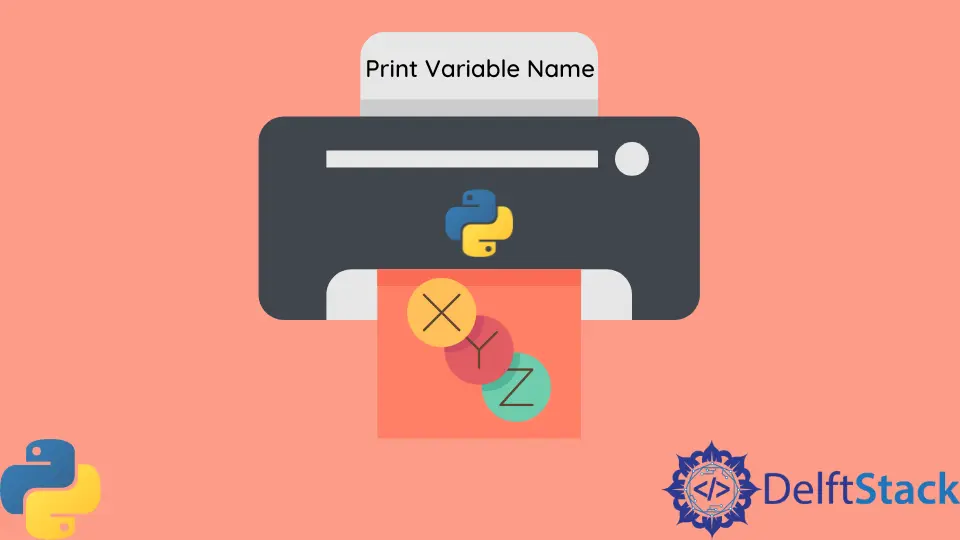
This tutorial will discuss the method to display a variable name in Python.
Print Variable Name With the globals()
Function in Python
The globals()
function returns the current global symbol table’s dictionary. A symbol table is a data structure maintained by a compiler that contains all of the program’s necessary information. This necessary information includes variable names, functions, classes, etc.
Similarly, there is another function called the locals()
function that returns the current local symbol table’s dictionary. The local symbol table contains information related to the local scope of the program, while the global symbol table contains information related to the program’s global scope. The local scope can refer to a specific class or a particular function, while the global scope refers to the whole program. The only difference between the globals()
and the locals()
function is the scope. We can search this dictionary to find a value that matches the value of the desired variable.
The following code example shows how to print a variable’s name with the globals()
function.
variable = 22
vnames = [name for name in globals() if globals()[name] is variable]
print(vnames)
Output:
['variable']
We used list comprehensions to iterate through each element inside the dictionary obtained by the globals()
function and returned the key for the item that matched our variable value. We stored the returned values inside the vnames
list and displayed all the vnames
elements.
Print Variable Name With a Dictionary in Python
As discussed above, both globals()
and locals()
functions return a dictionary that maps variables to their values. We can replicate that same functionality by creating a dictionary that contains variable names and their corresponding values in the form of a (key, value) pair. The following code example shows how to print variable names with a dictionary.
variable1 = 22
variable2 = 23
vnames = {22: "variable1", 23: "variable2"}
print(vnames[variable1])
Output:
variable1
We stored the variable values as keys and the corresponding variable names as the vnames
dictionary values. Whenever we have to print the name of a particular variable, we have to pass the value inside vnames[]
. This implementation is concise but needs to be constantly updated whenever a variable is modified.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn