How to Fix OverflowError: Math Range Error in Python
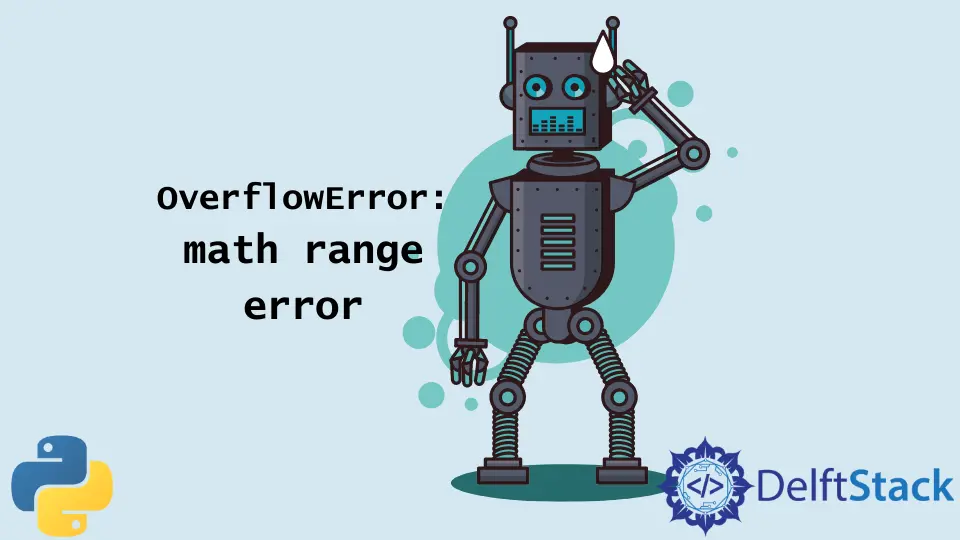
The float type variables are used to represent numbers with a decimal point. It stores values between a given range and uses the inf
string to represent values that exceed this range.
The math
library is used to perform various mathematical operations. This tutorial will discuss OverflowError: math range error
in Python.
Fix the OverflowError: math range error
in Python
An OverflowError
indicates that the raised error is due to exceeding the range of some data type. This specific error occurs when we perform a mathematical operation using the math
library but exceeding the float type’s decimal range.
For example,
import math
print(math.exp(2999))
Output:
OverflowError: math range error
In the above example, we get the error when using the math.exp()
function. This function calculates the exponential value of a given number.
In our example, the calculated value exceeds the range of decimals in float, so we get the error.
We need to be mindful of the range while calculating such calculations. There is no fix, so we can use the try
and except
blocks to work around this error.
We can also use the if-else
statements similarly, which is inefficient as we will have to check the input operands for the function beforehand.
We will put the code that can raise the error in the try
block and the alternative code in the except
block. If no error is raised, the code in the try
block is executed; otherwise, the code in the except
block will run.
For example,
import math
try:
print(math.exp(2999))
except:
print("Error")
Output:
Error
In the above example, the try
code block raised the error, so the except
code block was executed.
We can also use alternative mathematical functions in the numpy
library. The numpy
library returns the inf
constant whenever the range is exceeded.
For example,
import numpy
print(numpy.exp(2999))
Output:
inf
In the above example, the numpy.exp
function returns inf
since the output exceeds the range.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python