How to Make a Grade Converter in Python
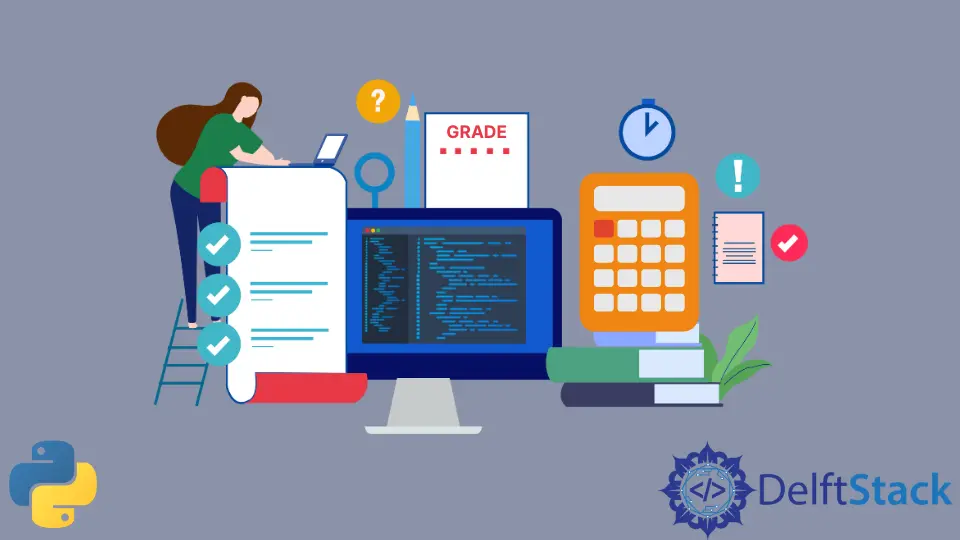
Python, with its simplicity and versatility, serves as an excellent programming language for developing practical tools. In this article, we’ll delve into the creation of two distinct grade calculators using Python – one simple and the other weighted.
These calculators will allow educators to efficiently compute students’ grades based on various criteria.
Create a Simple Grade Calculator in Python
Here, we will walk through the key steps to create a simple grade calculator. The program will take individual scores as input, calculate the average, and assign a letter grade based on predefined score ranges.
-
The first step is to get input from the user for individual scores. We will use a loop to collect scores for each subject.
-
After obtaining scores, we’ll calculate the average by summing all scores and dividing by the number of subjects.
-
Based on the average score, we’ll use
if-else
conditions to assign a letter grade to the student. Different grade ranges will correspond to different letter grades.
Code Example:
total_marks = 0
print("Enter Marks Obtained in 4 Subjects:")
for i in range(1, 5):
marks = int(input("Enter the marks for subject " + str(i) + ": "))
total_marks += marks
avg_marks = total_marks / 4
if 90 <= avg_marks <= 100:
print("Grade: A+")
elif 80 <= avg_marks < 90:
print("Grade: A")
elif 70 <= avg_marks < 80:
print("Grade: B+")
elif 60 <= avg_marks < 70:
print("Grade: B")
elif 50 <= avg_marks < 60:
print("Grade: C+")
elif 40 <= avg_marks < 50:
print("Grade: C")
else:
print("Grade: F")
Output:
Enter Marks Obtained in 4 Subjects:
Enter the marks for subject 1: 90
Enter the marks for subject 2: 80
Enter the marks for subject 3: 60
Enter the marks for subject 4: 70
Grade: B+
Here, as you can see, the variable total_marks
is initialized to zero to keep track of the cumulative sum of scores entered by the user.
total_marks = 0
We then prompt the user to enter the marks obtained in four subjects. The for
loop iterates from 1
to 4
, inclusive, and within each iteration, the user is prompted to input the marks for the corresponding subject.
The entered marks are converted to an integer using int()
, and the total_marks
is updated by adding the entered marks.
print("Enter Marks Obtained in 4 Subjects:")
for i in range(1, 5):
marks = int(input("Enter the marks for subject " + str(i) + ": "))
total_marks += marks
After obtaining all the subject scores, the average (avg_marks
) is calculated by dividing the total_marks
by the number of subjects (4
in this case).
avg_marks = total_marks / 4
We then use a series of if-elif-else
conditions to determine the letter grade based on the calculated average. The conditions check the average score against predefined ranges for each grade (A+
, A
, B+
, B
, C+
, and C
), and the corresponding grade is printed to the console.
if 90 <= avg_marks <= 100:
print("Grade: A+")
elif 80 <= avg_marks < 90:
print("Grade: A")
elif 70 <= avg_marks < 80:
print("Grade: B+")
elif 60 <= avg_marks < 70:
print("Grade: B")
elif 50 <= avg_marks < 60:
print("Grade: C+")
elif 40 <= avg_marks < 50:
print("Grade: C")
else:
print("Grade: F")
Feel free to customize the code to suit your needs or expand it for more advanced grading criteria.
Create a Weighted Grade Converter in Python
A weighted grade calculator in Python is a more sophisticated tool that considers different weightage for various components such as exams, lab tasks, and assignments. Here, we will guide you through the steps to create a weighted grade calculator.
The program will prompt the user for scores in different categories, apply weights, calculate the total average, and assign a letter grade based on predefined score ranges.
-
Begin by prompting the user to input marks obtained in different categories, such as exams, lab tasks, and assignments. Use appropriate prompts within loops to collect this information.
-
Calculate the average for each category, considering the assigned weights. For example, you might assign 10% weight to assignments, 20% to lab tasks, and 70% to exams. Calculate the weighted average using these weights.
-
Combine the weighted averages to calculate the total average. In this example, the total average is calculated by adding the weighted averages of assignments, lab tasks, and exams.
-
Similar to the simple grade calculator, use
if-else
conditions to assign a letter grade based on the calculated total average.
Complete Code Example:
total_marks = 0
exam1 = int(input("Enter Marks Obtained in Exam 1: "))
exam2 = int(input("Enter Marks Obtained in Exam 2: "))
lab_total = 0
assignment_total = 0
for i in range(1, 3):
lab_marks = int(input("Enter Marks Obtained in Lab Task " + str(i) + ": "))
lab_total += lab_marks
for i in range(1, 5):
assignment_marks = int(input("Enter Marks Obtained in Assignment " + str(i) + ": "))
assignment_total += assignment_marks
avg_exam = (exam1 + exam2) / 2
avg_lab = lab_total / 2
avg_assignment = assignment_total / 4
total_avg = 0.1 * avg_assignment + 0.7 * avg_exam + 0.2 * avg_lab
if 90 <= total_avg <= 100:
print("Grade: A+")
elif 80 <= total_avg < 90:
print("Grade: A")
elif 70 <= total_avg < 80:
print("Grade: B+")
elif 60 <= total_avg < 70:
print("Grade: B")
elif 50 <= total_avg < 60:
print("Grade: C+")
elif 40 <= total_avg < 50:
print("Grade: C")
else:
print("Grade: F")
Output:
Enter Marks Obtained in Exam 1: 80
Enter Marks Obtained in Exam 2: 75
Enter Marks Obtained in Lab Task 1: 60
Enter Marks Obtained in Lab Task 2: 70
Enter Marks Obtained in Assignment 1: 90
Enter Marks Obtained in Assignment 2: 85
Enter Marks Obtained in Assignment 3: 80
Enter Marks Obtained in Assignment 4: 75
Grade: B+
In the provided code, we initialized the variable total_marks
to zero, which will later be used to accumulate the user’s scores.
total_marks = 0
User input is collected for the marks obtained in two exams (exam1
and exam2
). The int(input(...))
syntax is used to convert the entered values into integers.
exam1 = int(input("Enter Marks Obtained in Exam 1: "))
exam2 = int(input("Enter Marks Obtained in Exam 2: "))
To calculate the weighted averages, we initialize variables lab_total
and assignment_total
to zero. Subsequently, a loop is employed to gather marks for two lab tasks, and the total is updated accordingly.
lab_total = 0
assignment_total = 0
for i in range(1, 3):
lab_marks = int(input("Enter Marks Obtained in Lab Task " + str(i) + ": "))
lab_total += lab_marks
Another loop is used to obtain marks for four assignments, updating the assignment_total
accordingly.
for i in range(1, 5):
assignment_marks = int(input("Enter Marks Obtained in Assignment " + str(i) + ": "))
assignment_total += assignment_marks
The weighted averages for exams, lab tasks, and assignments are calculated using the appropriate formulas. For instance, avg_exam
is the average of exam1
and exam2
, avg_lab
is the average of the lab tasks, and avg_assignment
is the average of the assignments.
avg_exam = (exam1 + exam2) / 2
avg_lab = lab_total / 2
avg_assignment = assignment_total / 4
The total average (total_avg
) is calculated by combining the weighted averages with their respective weights (10% for assignments, 20% for lab tasks, and 70% for exams).
total_avg = 0.1 * avg_assignment + 0.7 * avg_exam + 0.2 * avg_lab
Finally, the program utilizes a series of if-elif-else
conditions to determine the letter grade based on the calculated total average. The grade is then printed to the console.
if 90 <= total_avg <= 100:
print("Grade: A+")
elif 80 <= total_avg < 90:
print("Grade: A")
elif 70 <= total_avg < 80:
print("Grade: B+")
elif 60 <= total_avg < 70:
print("Grade: B")
elif 50 <= total_avg < 60:
print("Grade: C+")
elif 40 <= total_avg < 50:
print("Grade: C")
else:
print("Grade: F")
Customize the code as needed for your specific grading criteria.
Conclusion
These Python grade calculators offer practical solutions for educators seeking efficient tools to evaluate students’ performance. Whether opting for simplicity or considering weighted criteria, the flexibility of Python enables the creation of tailored solutions to meet diverse grading needs.
Educators can use and customize these calculators based on specific grading policies, ensuring accurate and consistent evaluation of students. As we embrace technology, Python proves to be a valuable ally in streamlining and enhancing traditional processes.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn