How to Find the Most Common Elements of a List in Python
-
Use the
most_common()
ofCounter
to Find the Most Common Elements of a List in Python -
Use the
max()
Function ofFreqDist()
to Find the Most Common Elements of a List in Python -
Use the
unique()
Function ofNumPy
to Find the Most Common Elements of a List in Python
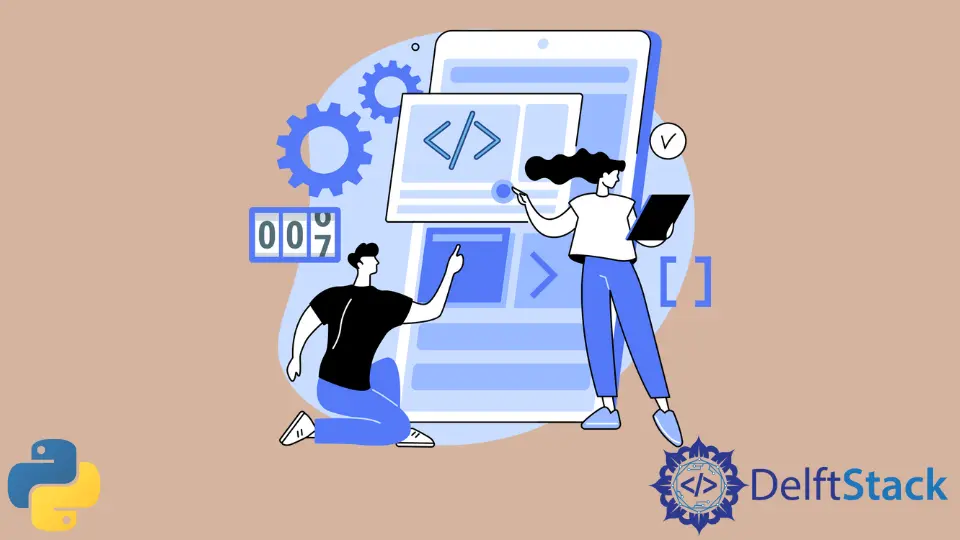
This article mentions several ways to find the most common elements of a list in Python. The following are the functions that we can use to find the most common list elements in Python.
- Use the
most_common()
function ofCounter
. - Use the
max()
function ofFreqDist()
. - Use the
unique()
function ofNumPy
.
Use the most_common()
of Counter
to Find the Most Common Elements of a List in Python
In Python 2.7+, use the Counter()
command to find the most common list elements in Python. For this, you need to import the Counter
class from the collections
standard library.
A Counter
is a collection where elements are stored as dictionary keys, and the key’s counts are stored as dictionary values. The example below illustrates this.
from collections import Counter
list_of_words = ["Cars", "Cats", "Flowers", "Cats", "Cats", "Cats"]
c = Counter(list_of_words)
c.most_common(1)
print("", c.most_common(1))
Here, the top one element is determined by using the most_common()
function as most_common(1)
.
Output:
[("Cats", 4)]
Use the max()
Function of FreqDist()
to Find the Most Common Elements of a List in Python
You can also use the max()
command of FreqDist()
to find the most common list elements in Python. For this, you import the nltk
library first. The example below demonstrates this.
import nltk
list_of_words = ["Cars", "Cats", "Flowers", "Cats"]
frequency_distribution = nltk.FreqDist(list_of_words)
print("The Frequency distribution is -", frequency_distribution)
most_common_element = frequency_distribution.max()
print("The most common element is -", most_common_element)
Here, the frequency distribution list is first built using the FreqDist()
function, and then the most common element is determined by using the max()
function.
Output:
The Frequency distribution is - <FreqDist with 3 samples and 4 outcomes>
The most common element is - Cats
Use the unique()
Function of NumPy
to Find the Most Common Elements of a List in Python
Lastly, you can use the NumPy
library’s unique()
function to find the most common elements of a list in Python. The following example below illustrates this.
import numpy
list_of_words = [
"Cars",
"Cats",
"Flowers",
"Cats",
"Horses",
"",
"Horses",
"Horses",
"Horses",
]
fdist = dict(zip(*numpy.unique(list_of_words, return_counts=True)))
print("The elements with their counts are -", fdist)
print("The most common word is -", list(fdist)[-1])
The output of this operation is a dictionary of key-value pairs, where the value is the count of a particular word. Use the unique()
function to find the unique elements of an array. Next, the zip()
command is used to map the similar index of multiple containers. In this example, we use it to get the frequency distribution. Since the output lists the key-value pairs in ascending order, the most common element is determined by the last element.
Output:
The elements with their counts are - {'': 1, 'Cars': 1, 'Cats': 2, 'Flowers': 1, 'Horses': 4}
The most common word is - Horses
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python