How to Get Range Backwards in Python
-
Range Backwards in Python Using the
range()
Function -
Range Backwards in Python Using the
reversed()
Function - Range Backward in Python Using Extra Variable
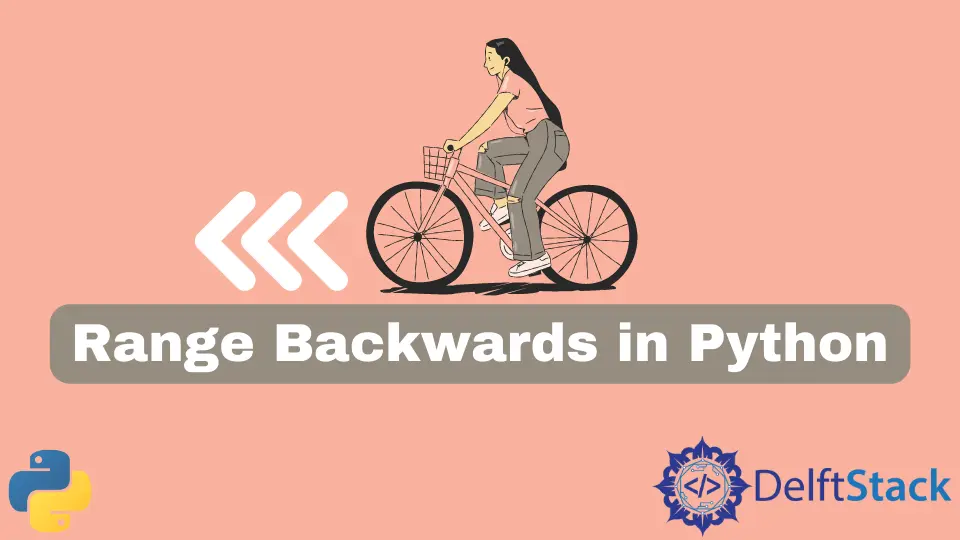
This tutorial will explain multiple ways to range or loop backward in Python. Backward range means starting the loop from the largest index and iterating backward till the smallest index.
Range Backwards in Python Using the range()
Function
To range backward, we can use the range()
method and pass starting index like 100
as the first argument, stopping index like -1
(as we want to iterate till 0
) as the second argument, and step size of -1
as the iteration is backward.
100
to 50
.The example code to implement backward loop is below:
for i in range(100, -1, -1):
# do something
pass
Range Backwards in Python Using the reversed()
Function
Another way to range backward in Python is to use the reversed()
function that takes the range()
as the input. The example code below demonstrates how to implement a backward loop using the reversed()
function.
for i in reversed(range(100)):
# do something
pass
The above code will start from 99
and iterate till 0
.
Range Backward in Python Using Extra Variable
A simple approach is to initialize another variable and subtract it with the range()
variable to loop backward.
Example code:
for x in range(100):
i = 100 - x
# do something