How to Append List to Another List in Python
-
Use a
for
Loop to Append List to Another List in Python -
Use the
extend()
Method to Append List to Another List in Python -
Use the
chain()
Function From theitertools
Module to Append List to Another List in Python -
Use the Concatenation
+
Operator to Append List to Another List in Python - Use List Comprehension to Append List to Another List in Python
-
Use Unpacking Generalizations
*
to Append List to Another List in Python - Use List Slicing to Append List to Another List in Python
- Conclusion
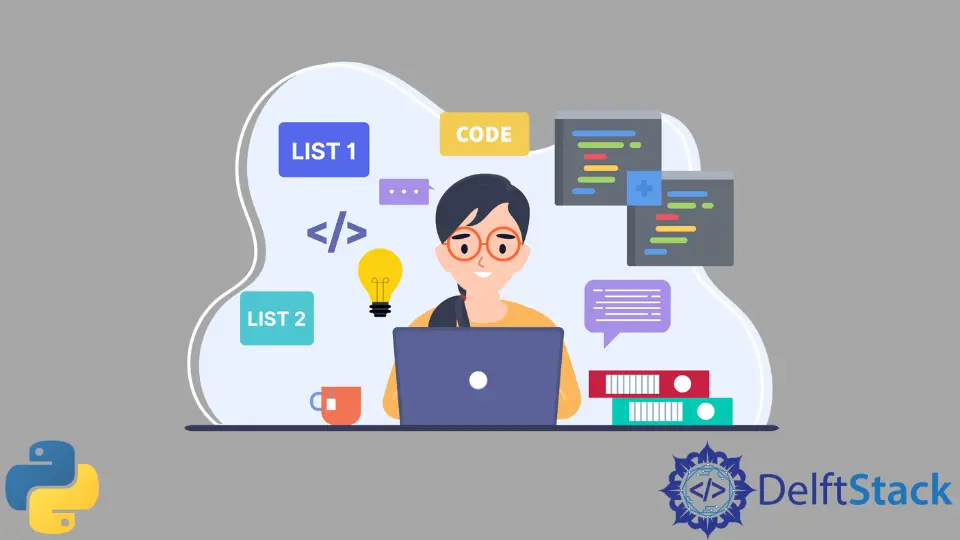
In Python programming, combining or appending lists is a common task that allows you to merge multiple lists into one.
Understanding how to append lists in Python is essential for data manipulation and organization. This comprehensive guide will explore different methods to append one list to another.
Through code examples and explanations, you will gain a solid understanding of how these techniques work and when to use them. By the end of this article, you will be equipped with the knowledge to append lists in your Python projects efficiently.
Use a for
Loop to Append List to Another List in Python
The for
loop can append the original list into another list by appending the elements individually. A single loop can implement this task without divulging into nesting or any other concept.
Here’s an example.
l1 = [1, 2, 3]
l2 = ["Football", "Basketball", "Cricket"]
# using for loop with append() function
for x in l2:
l1.append(x)
print(l1)
Output:
Use the extend()
Method to Append List to Another List in Python
Python has a built-in method for lists named extend()
that accepts an iterable as a parameter and adds it to the last position of the current iterable. Using it for lists will append the list parameter after the last element of the main list.
For example, declare two lists and add the second list to the main list using the method extend()
.
l1 = [1, 2, 3]
l2 = ["Football", "Basketball", "Cricket"]
l1.extend(l2)
print(l1)
Output:
The extend()
method provides a straightforward way to append a list to an existing list with a simple function call.
Use the chain()
Function From the itertools
Module to Append List to Another List in Python
The itertools
is a Python module containing fast and efficient utility methods for iterables. This module has the function chain()
that accepts a variable number of same-type iterables and concatenates them together in sequence based on the parameters.
We can use the chain()
function to append multiple lists and form them into a single list.
For this example, declare three lists and set them as parameters for the itertools.chain()
function. We then wrap the function with another function, list()
, which initializes a single list from the return value of the chain()
function.
import itertools
l1 = [1, 2, 3]
l2 = ["Football", "Basketball", "Cricket"]
lst_all = list(itertools.chain(l1, l2))
print(lst_all)
Output:
Using itertools.chain()
, the parameters can be as many or as few as you want, and you will be provided with an efficient way of concatenating lists together and forming them into a single list.
Use the Concatenation +
Operator to Append List to Another List in Python
Another simple method of appending multiple lists together is to use the +
operator, which supports list concatenation in Python.
Perform the concatenation +
operation on existing list variables, and the output will be a single combined list in order of the operands inputted in the code.
l1 = [1, 2, 3]
l2 = ["Football", "Basketball", "Cricket"]
lst_all = l1 + l2
print(lst_all)
Output:
Use List Comprehension to Append List to Another List in Python
List comprehension provides a concise way to append one list to another while performing any necessary transformations or filtering.
Consider the following example.
l1 = [x for x in range(1, 4)]
l2 = ["Football", "Basketball", "Cricket"]
combined_list = l1 + l2
print(combined_list)
Output:
Use Unpacking Generalizations *
to Append List to Another List in Python
We can use the unpacking generalizations to disperse or unpack a given list into multiple variables. The *
operator can unpack a list into several variables.
This is a relatively newer process and is only available for the newer versions of Python.
Consider the following example code.
l1 = [x for x in range(1, 4)]
l2 = ["Football", "Basketball", "Cricket"]
# unpacking generalizations
# unpack both lists in a list literal
l3 = [*l1, *l2]
print(l3)
Output:
We should note that the unpacking generalizations method only works for Python 3.5 and above versions.
Use List Slicing to Append List to Another List in Python
List slicing is usually utilized to get a part of the list from an existing list. However, we can use list slicing to append a certain part of the list into another list.
Consider the following example.
l1 = [1, 2, 3]
l2 = ["Football", "Basketball", "Cricket"]
# using slicing assignment
l1[len(l1) :] = l2
print(l1)
Output:
Conclusion
Appending one list to another is a fundamental operation in Python, allowing you to combine multiple lists into one entity. In this comprehensive guide, we explored different methods to achieve this.
Each method has advantages, and the choice depends on your requirements. You can efficiently manipulate and organize data in your Python projects by mastering these techniques.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python