How to Loop Through Files in Directory in Python
-
Loop Through Files in a Directory in Python Using the
os.listdir()
Method -
Loop Through Files in a Directory in Python Using the
pathlib.path().glob()
Method -
Loop Through the Files in a Directory in Python Using the
os.walk()
Method -
Loop Through the Files in a Directory in Python Using the
iglob()
Method
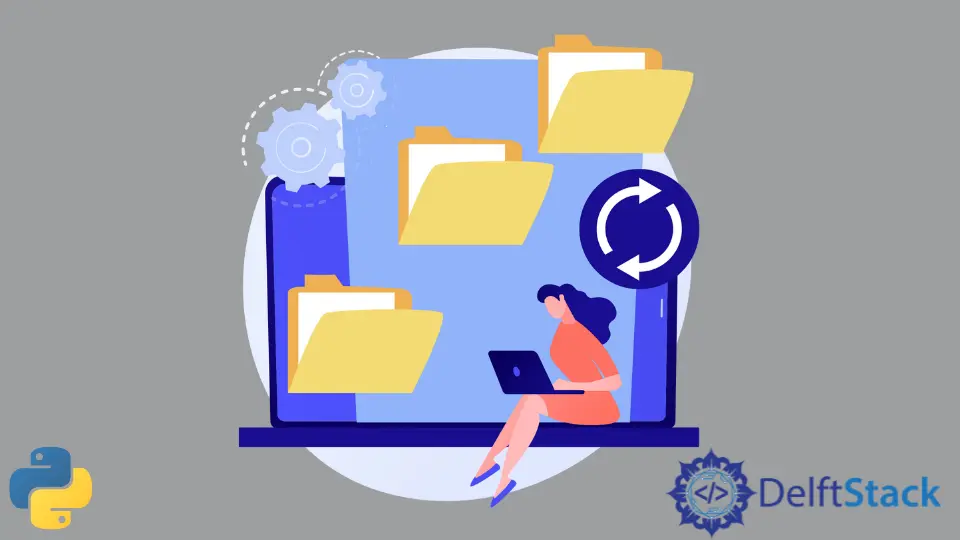
This tutorial will explain different ways to loop through the files in a directory in Python. We need to look for a file in a directory to implement features like file sharing, file viewing, or file loading to perform some action on the data saved in it.
Suppose we have a file name and need to read data from that file, we need some method to loop through the files in the directory to find the file we need. The various methods used to loop through the files in a directory in Python are explained below.
Loop Through Files in a Directory in Python Using the os.listdir()
Method
The listdir()
method of the os
module takes the directory path as input and returns a list of all the files in that directory. As we want to find the specific file in the directory, we will have to loop through the files’ names to find the required file. The code example below demonstrates how to find a specific file by iterating through the file using the listdir()
method in Python.
import os
files = os.listdir("Desktop/myFolder")
myfile = "filename.txt"
for filename in files:
if filename == myfile:
continue
Loop Through Files in a Directory in Python Using the pathlib.path().glob()
Method
The path()
method of the pathlib
module takes the directory path string as input and returns the list of all the files’ paths in the directory and subdirectories.
Suppose the file we want to find is .txt
file, we can get paths of all the .txt
files by using the path().glob()
method. The below code example demonstrates how to loop through all the .txt
files in a directory using the path
method in Python.
from pathlib import Path
pathlist = Path("Desktop/myFolder").glob("**/*.txt")
myfile = "filename.txt"
for path in pathlist:
if path.name == myfile:
continue
The pattern **/*.txt
returns all the files with the txt
extension in the current folder and its subfolders. path.name
returns the file name only but not the full path.
Loop Through the Files in a Directory in Python Using the os.walk()
Method
The walk()
method of the os
module also takes the directory path string as input and returns the path of the root directory as a string, the list of the subdirectories, and the list of all the files in the current directory and its subdirectories.
To find the file with the name filename.txt
, we can first get all the files in the directory and then loop through them to get the desired file. The below code example demonstrates how to find a file by looping through the files in a directory.
import os
myfile = "filename.txt"
for root, dirs, files in os.walk("Desktop/myFolder"):
for file in files:
if file == myfile:
print(file)
Loop Through the Files in a Directory in Python Using the iglob()
Method
The iglob()
method of the glob()
module takes the directory path and extension of the required file as input and returns all the files’ path with the same extension. The below code example demonstrate how to loop through the files in a directory and find the required file in Python using the iglob()
method.
import glob
for filepath in glob.iglob("drive/test/*.txt"):
if filepath.endswith("/filename.txt"):
print(filepath)