How to Catch the KeyboardInterrupt Error in Python
-
Use the
try...except
Statement to Catch theKeyboardInterrupt
Error in Python -
Use Signal Handlers to Catch the
KeyboardInterrupt
Error in Python
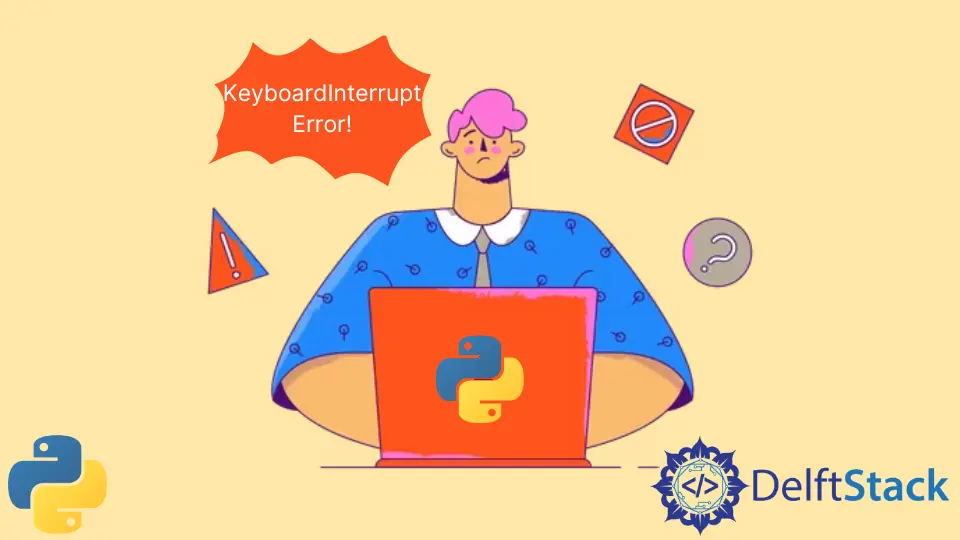
The KeyboardInterrupt
error occurs when a user manually tries to halt the running program by using the Ctrl + C or Ctrl + Z commands or by interrupting the kernel in the case of Jupyter Notebook. To prevent the unintended use of KeyboardInterrupt
that often occurs, we can use exception handling in Python.
In this guide, you’ll learn how to catch the KeyboardInterrupt
error in Python.
Use the try...except
Statement to Catch the KeyboardInterrupt
Error in Python
The try...except
statement is used when it comes to the purpose of exception handling in Python. The try...except
statement has a unique syntax; it is divided into three blocks, all of which have a different purpose and function in the Python code.
- The
try
block contains the cluster of code that has to be checked for any error by the interpreter. - The
except
block is utilized to add the needed exceptions and bypass the errors of the code. - The
finally
block contains the statements that need to be executed without checking and ignored by thetry
andexcept
blocks.
To explain the code for KeyboardInterrupt
in Python, we take a simple program that asks the user for input while manually handling the KeyboardInterrupt
exception.
The following code uses the try...except
statement to catch the KeyboardInterrupt
error in Python.
try:
x = input()
print("Try using KeyboardInterrupt")
except KeyboardInterrupt:
print("KeyboardInterrupt exception is caught")
else:
print("No exceptions are caught")
The program above provides the following output.
KeyboardInterrupt exception is caught
In the code above, the input function resides between the try
block and is left empty as further details are not needed in this case. Then, the except
block handles the KeyboardInterrupt
error. The KeyboardInterrupt
error is manually raised so that we can identify when the KeyboardInterrupt
process occurs.
Python allows the definition of as many except
blocks as the user wants in a chunk of code.
Use Signal Handlers to Catch the KeyboardInterrupt
Error in Python
The signal
module is utilized to provide functions and mechanisms that use signal handlers in Python. We can catch the SIGINT
signal, which is basically an interrupt from the keyboard Ctrl+C. Raising the KeyboardInterrupt
is the default action when this happens.
The sys
module in Python is utilized to provide several necessary variables and functions used to manipulate distinct parts of the Python runtime environment.
The signal
and sys
modules need to be imported to the Python code to use this method successfully without any error.
The following code uses signal handlers to catch the KeyboardInterrupt
error in Python.
import signal
import sys
def sigint_handler(signal, frame):
print("KeyboardInterrupt is caught")
sys.exit(0)
signal.signal(signal.SIGINT, sigint_handler)
The code above provides the following output.
KeyboardInterrupt is caught
In the code above, the signal.signal()
function is utilized to define custom handlers to be executed when a signal of a certain type is received.
We should note that a handler, once set for a particular signal, remains installed until the user manually resets it. In this case, the only exception is the handler for SIGCHLD
.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn