How to Resize Image in Python
-
Resize an Image Using
resize()
Method ofPIL
Module in Python -
Resize an Image Using
resize()
Method ofOpenCV
Module in Python -
Resize an Image Using
resize()
Method ofscikit-image
Module in Python - Conclusion
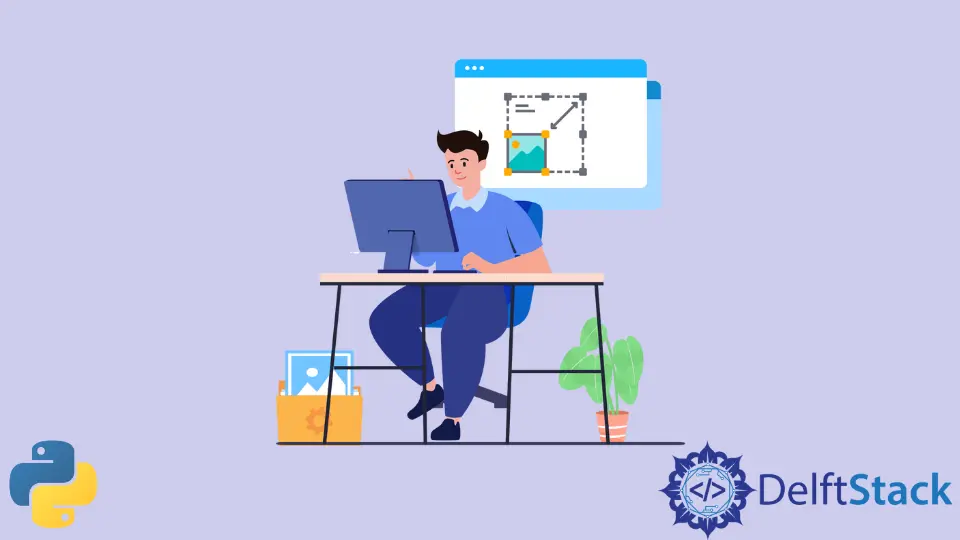
The ability to resize images, a fundamental aspect of this domain, is crucial for a myriad of applications - from web development to machine learning.
The imresize()
method is used to resize an image in Python, available in the scipy
module. Unfortunately, this method is now deprecated in scipy 1.0.0
and will be completely removed from scipy 1.3.0
.
This comprehensive article will guide you through alternative methods to resize images using Python, focusing on three powerful libraries: PIL
(Python Imaging Library), OpenCV
(Open Source Computer Vision Library), and Scikit-Image
.
Resize an Image Using resize()
Method of PIL
Module in Python
The PIL
is an acronym for Python Imaging Library, which contains some modules for image processing. We can resize an image using the resize()
method of the Image
class available in the PIL
module.
First, install the PIL
module:
pip install pillow
Pillow is an open-source library that adds support for opening, manipulating, and saving many different image file formats.
import cv2
# Read the image
img = cv2.imread("example.jpg")
# Compute the new size: half the original size
height, width = img.shape[:2]
new_size = (width // 2, height // 2)
# Resize the image
resized_img = cv2.resize(img, new_size, interpolation=cv2.INTER_AREA)
# Save the resized image
cv2.imwrite("resized_example.jpg", resized_img)
# Print a success message
print("Image resized and saved as resized_example.jpg")
We start by importing the Image
module from Pillow
, a fundamental step for any image operation. Next, we use the Image.open()
function to open the desired image file, example.jpg
.
Then, we define a tuple new_size
with the dimensions 300, 300
, setting the new size for our image. Following this, the resize()
function is called on our image object, resizing it to the dimensions we specified in new_size
.
After resizing, we save the modified image as resized_example.jpg
, ensuring our changes are preserved.
Finally, we print a confirmation message: The image has been resized and saved as resized_example.jpg
, signaling the successful completion of our image resizing process.
Output:
Resize an Image Using resize()
Method of OpenCV
Module in Python
OpenCV (Open Source Computer Vision Library) is a highly regarded tool in this domain. This library, written in C++ and with interfaces for Python, is designed for computational efficiency and real-time applications.
In this section, we’ll focus on how to resize images using OpenCV
in Python, a task crucial for applications ranging from image manipulation to machine learning.
First, install the OpenCV
module:
pip install opencv-python
import cv2
# Read the image
img = cv2.imread("resized.jpg")
# Compute the new size: half the original size
height, width = img.shape[:2]
new_size = (width // 2, height // 2)
# Resize the image
resized_img = cv2.resize(img, new_size, interpolation=cv2.INTER_AREA)
# Save the resized image
cv2.imwrite("resized_example.jpg", resized_img)
# Print a success message
print("Image resized and saved as resized_example.jpg")
In this section, we explore image resizing using OpenCV
, a robust library for image processing in Python. We begin by importing OpenCV
with import cv2
.
To process an image, we first read it into memory using cv2.imread("example.jpg")
. Next, we determine the new size for our image by extracting its dimensions with img.shape[:2]
and then halving these dimensions.
We resize the image using cv2.resize(img, new_size, interpolation=cv2.INTER_AREA)
, where INTER_AREA
interpolation is optimal for reducing size.
After resizing, we save the modified image to a new file with cv2.imwrite("resized_example.jpg", resized_img)
, ensuring our changes are preserved.
Finally, we print a confirmation message with print("Image resized and saved as resized_example.jpg")
, indicating the successful completion of the resizing process.
Output:
Resize an Image Using resize()
Method of scikit-image
Module in Python
In the field of image processing and computer vision in Python, Scikit-Image
, a part of the SciPy ecosystem, is a prominent library offering a wide array of algorithms and tools. Among its various functionalities, image resizing is a fundamental and frequently utilized operation.
This article delves into the methodology of resizing images using Scikit-Image
, exploring its significance, application, and technical aspects.
First, install the scikit-image
module:
pip install scikit-image
from skimage import io, transform, img_as_ubyte
# Load the image
image = io.imread("example.jpg")
# Define the desired output size (width, height)
output_size = (100, 100)
# Resize the image
resized_image = transform.resize(image, output_size, anti_aliasing=True)
# Convert to 8-bit (0 to 255) format
resized_image_ubyte = img_as_ubyte(resized_image)
# Save the resized image
io.imsave("resized_example.jpg", resized_image_ubyte)
# Print a success message
print("Image resized and saved as resized_example.jpg")
In this code snippet, we begin by importing necessary modules from skimage
. We load the image using io.imread
, a straightforward method for reading images into a NumPy array.
We define the output_size
, a tuple representing the desired dimensions width, height
. The transform.resize
function is then employed to resize the image; here, we enable anti_aliasing
to smooth the image, which is beneficial when reducing its size.
We then use the img_as_ubyte
function to convert the image data to an 8-bit format. This function handles the normalization and data type conversion, ensuring compatibility with common image formats.
Finally, we save the resized image using io.imsave
and print a confirmation message. This process exemplifies the ease and efficiency of using Scikit-Image
for basic image processing tasks like resizing.
Output:
Conclusion
We’ve explored how the PIL
, OpenCV
, and Scikit-Image
libraries each provide unique approaches to resizing images, demonstrating the flexibility and robustness of Python in handling image processing tasks. From the straightforward and user-friendly methods in PIL
, to the high-performance and feature-rich OpenCV
, and the scientific depth of Scikit-Image
, Python stands as a premier choice for developers and researchers in the field of image processing.
Whether preparing data in machine learning, optimizing images for web applications, or conducting advanced image analysis, the skills and insights gained from this article will undoubtedly be valuable assets in your future projects.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn