How to Get Unique Values From a List in Python
-
Use
dict.fromkeys
to Get Unique Values From a List in Python - Use a New List to Get Unique Values From the Original List in Python
-
Use
set()
to Get Unique Values From a List in Python
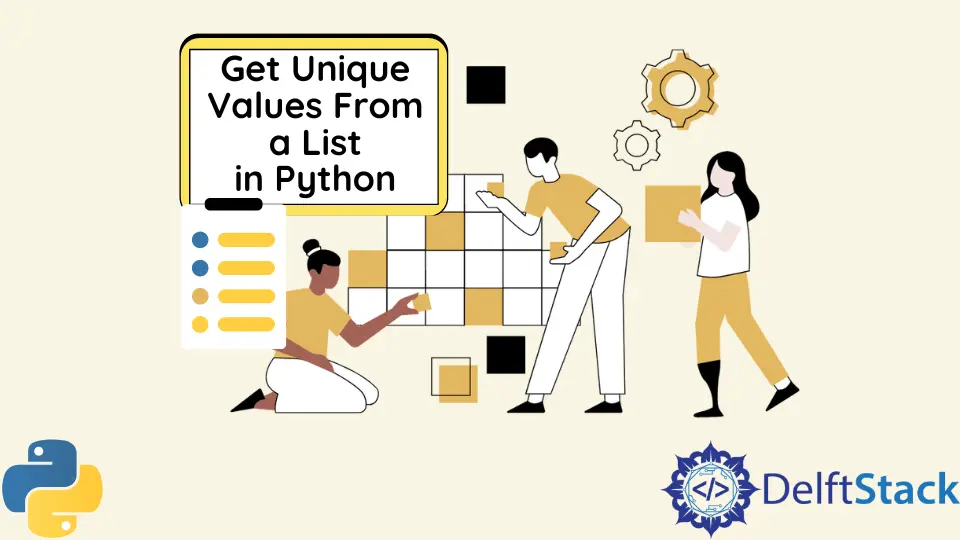
This tutorial discusses methods to get unique values from a list in Python.
Use dict.fromkeys
to Get Unique Values From a List in Python
We can use the dict.fromkeys
method of the dict
class to get unique values from a Python list. This method preserves the original order of the elements and keeps only the first element from the duplicates. The below example illustrates this.
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = list(dict.fromkeys(inp_list))
print(unique_list)
Output:
[2, 3, 1, 4, 5]
Use a New List to Get Unique Values From the Original List in Python
Another method to get unique values from a list in Python is to create a new list and add only unique elements to it from the original list. This method preserves the original order of the elements and keeps only the first element from the duplicates. The below example illustrates this.
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = []
[unique_list.append(x) for x in inp_list if x not in unique_list]
unique_list
Output:
[2, 3, 1, 4, 5]
Use set()
to Get Unique Values From a List in Python
Set
in Python holds unique values only. We can insert the values from our list to a set
to get unique values. However, this method does not preserve the order of the elements. The below example illustrates this.
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = list(set(inp_list))
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Note that the order of elements is not preserved as in the original list.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python