How to Do Exponents in Python
-
Use
**
Operator to Do Exponent in Python -
Use
pow()
ormath.power()
to Do Exponent in Python -
Use
numpy.np()
to Do Exponent in Python - Compare Runtimes for Each Solution
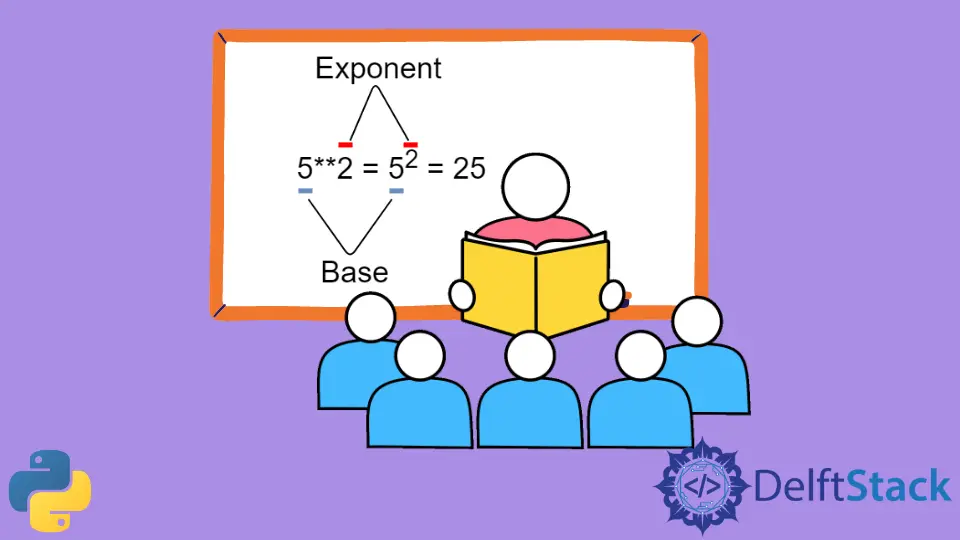
This tutorial will demonstrate how to do exponentiations in Python.
In mathematics, exponentiation is an operation where a number is multiplied several times with itself.
Python provides built-in operations and functions to help perform exponentiation.
Use **
Operator to Do Exponent in Python
Most developers seem to think the caret symbol ^
is the operator for getting the power of a number because the caret is used as a symbol for exponents in mathematics. However, in most programming languages, the caret symbol is reserved for the bit-wise xor
operator.
In Python, the exponent operator is symbolized by two consecutive asterisks **
between the base and exponent number.
The exponent operator’s functionality supplements the behavior of the multiplication operator *
; the difference is the second operand in the exponent operator is for setting the number of times the first operand is multiplied by itself.
print(5 ** 6)
To multiply the number 5
by itself 6
times, use the operator **
in between the base operand 5
and the exponent operand 6
.
Output:
15625
Let’s test this operator on different types of values.
We will initialize a whole number, a whole negative number, zero, and two float
values lesser than 1
and greater than 1
. Then we’ll assign random integers as their exponents.
num1 = 2
num2 = -5
num3 = 0
num4 = 1.025
num5 = 0.5
print(num1, "^12=", num1 ** 12)
print(num2, "^4=", num2 ** 4)
print(num3, "^9999=", num3 ** 9999)
print(num4, "^-3=", num4 ** -3)
print(num5, "^8=", num5 ** 8)
Output:
2^12= 4096
-5^4= 625
0^9999= 0
1.025^-3= 0.928599410919749
0.5^8= 0.00390625
Use pow()
or math.power()
to Do Exponent in Python
Another way to do exponent in Python is to use the function pow()
designed to exponentiate values given the base and the exponent. The math
module also has its own implementation of pow()
for the same purpose.
Both these functions have 2 arguments, the first argument is for the base number, and the second is for the exponent.
Let’s try calling both functions multiple times with the same arguments so we can compare their outputs.
import math
print(pow(-8, 7))
print(math.pow(-8, 7))
print(pow(2, 1.5))
print(math.pow(2, 1.5))
print(pow(4, 3))
print(math.pow(4, 3))
print(pow(2.0, 5))
print(math.pow(2.0, 5))
Output:
-2097152
-2097152.0
2.8284271247461903
2.8284271247461903
64
64.0
32.0
32.0
The only difference in the results is math.pow()
always returns a float
value even if whole number arguments are passed, while pow()
will only return float
if there is at least one float
argument.
Use numpy.np()
to Do Exponent in Python
The module NumPy
also has its own function power()
for exponentiation. power()
accepts the same arguments as the pow()
functions, where the first argument is the base value and the 2nd argument is the exponent value.
To use NumPy
, we should install it via pip
or pip3
.
- Python 2:
pip install numpy
- Python 3:
pip3 install numpy
Let’s print out the same set of examples in pow()
using numpy.power()
.
print(np.power(-8, 7))
print(np.power(2, 1.5))
print(np.power(4, 3))
print(np.power(2.0, 5))
Output:
-2097152
2.8284271247461903
64
32.0
power()
produces the same output as the built-in Python function pow()
where it will return a whole number if there are no float
arguments.
Compare Runtimes for Each Solution
Let’s compare the time it takes for these 3 functions and the **
operator to run with a large exponent value. For timing functions, we’ll import the timeit
module to print out each of the solutions’ runtime.
The base’s value will be 2
, and the value for the exponent will be 99999
.
import numpy as np
import math
import time
start = time.process_time()
val = 2 ** 99999
print("** took", time.process_time() - start, "ms")
start = time.process_time()
val = pow(2, 99999)
print("pow() took", time.process_time() - start, "ms")
start = time.process_time()
val = np.power(2, 99999)
print("np.power() took", time.process_time() - start, "ms")
start = time.process_time()
val = math.pow(2, 99999)
print("math.pow() took", time.process_time() - start, "ms")
Output:
** took 0.0006959999999999744 ms
pow() took 0.00039000000000000146 ms
np.power() took 1.6999999999989246e-05 ms
Traceback (most recent call last):
File "/Users/rayven/python/timeit.py", line 15, in <module>
val = math.pow(2,99999)
OverflowError: math range error
The most obvious thing to note is math.pow()
resulted in an OverflowError
. This means that math.pow()
can’t support large-valued exponents, most likely because of the way that this module has implemented exponentiation.
The difference between the 3 other methods are trivial, but from this example, np.power()
is the fastest function to perform exponentiation.
What if we try reducing the exponent to 9999
? Let’s see what math.pow()
outputs.
** took 1.0000000000010001e-05 ms
pow() took 4.000000000004e-06 ms
np.power() took 2.0000000000020002e-05 ms
math.pow() took 2.9999999999752447e-06 ms
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn