Dictionary With Multiple Values in Python
- Use a User-Defined Function to Add Multiple Values to a Key in a Dictionary
-
Use the
defaultdict
Module to Add Multiple Values to a Key in a Dictionary -
Use the
setdefault()
Method to Add Multiple Values to a Specific Key in a Dictionary
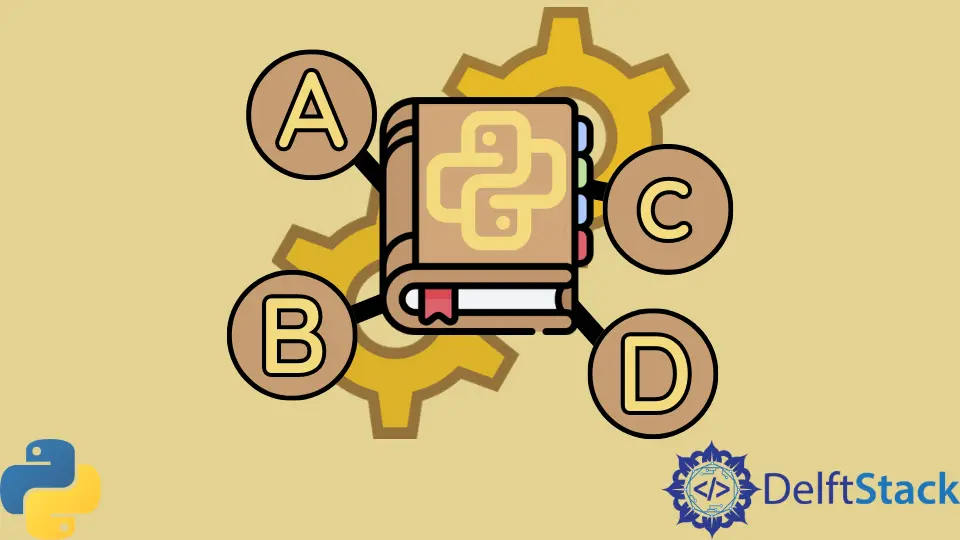
A dictionary in Python constitutes a group of elements in the form of key-value pairs. Usually, we have single values for a key in a dictionary.
However, we can have the values for keys as a collection like a list. This way, we can have multiple elements in the dictionary for a specific key.
For example,
d = {"a": [1, 2], "b": [2, 3], "c": [4, 5]}
print(d)
Output:
{'a': [1, 2], 'b': [2, 3], 'c': [4, 5]}
This tutorial will discuss different methods to append multiple values as a list in a dictionary in Python.
Use a User-Defined Function to Add Multiple Values to a Key in a Dictionary
We can create a function to append key-value pairs in a dictionary, with the value being a list.
For example,
def dict1(sample_dict, key, list_of_values):
if key not in sample_dict:
sample_dict[key] = list()
sample_dict[key].extend(list_of_values)
return sample_dict
word_freq = {
"example": [1, 3, 4, 8, 10],
"for": [3, 10, 15, 7, 9],
"this": [5, 3, 7, 8, 1],
"tutorial": [2, 3, 5, 6, 11],
"python": [10, 3, 9, 8, 12],
}
word_freq = dict1(word_freq, "tutorial", [20, 21, 22])
print(word_freq)
Output:
{'example': [1, 3, 4, 8, 10], 'for': [3, 10, 15, 7, 9], 'this': [5, 3, 7, 8, 1], 'tutorial': [2, 3, 5, 6, 11, 20, 21, 22], 'python': [10, 3, 9, 8, 12]}
Note that, In the above code, we have created a function dict1
with sample_dict
, key
and list_of_values
as parameters within the function. The extend()
function will append a list as the value for a key in the dictionary.
Use the defaultdict
Module to Add Multiple Values to a Key in a Dictionary
defaultdict
is a part of the collections module. It is also a sub-class of dict
class that returns dictionary-like objects. For a key that does not exist, it will give a default value.
Both defaultdict
and dict
have the same functionality except that defaultdict
never raises a keyerror
.
We can use it to convert a collection of tuples to a dictionary. We will specify the default value as a list. So whenever the same key is encountered, it will append the value as a list.
For example,
from collections import defaultdict
s = [("rome", 1), ("paris", 2), ("newyork", 3), ("paris", 4), ("delhi", 1)]
d = defaultdict(list)
for k, v in s:
d[k].append(v)
sorted(d.items())
print(d)
Output:
defaultdict(<class 'list'>,
{'rome': [1],
'paris': [2, 4],
'newyork': [3],
'delhi': [1]
})
Use the setdefault()
Method to Add Multiple Values to a Specific Key in a Dictionary
setdefault()
method is used to set default values to the key. When the key is present, it returns some value. Else it inserts the key with a default value. The default value for the key is none.
We will append the list as values for keys using this method.
For example,
s = [("rome", 1), ("paris", 2), ("newyork", 3), ("paris", 4), ("delhi", 1)]
data_dict = {}
for x in s:
data_dict.setdefault(x[0], []).append(x[1])
print(data_dict)
Output:
{'rome': [1], 'paris': [2, 4], 'newyork': [3], 'delhi': [1]}