How to Fix AttributeError: __Exit__ in Python
-
What Is the
__exit__()
-
How the
AttributeError: __exit__
Occurs -
How to Resolve the
AttributeError: __exit__
in Python
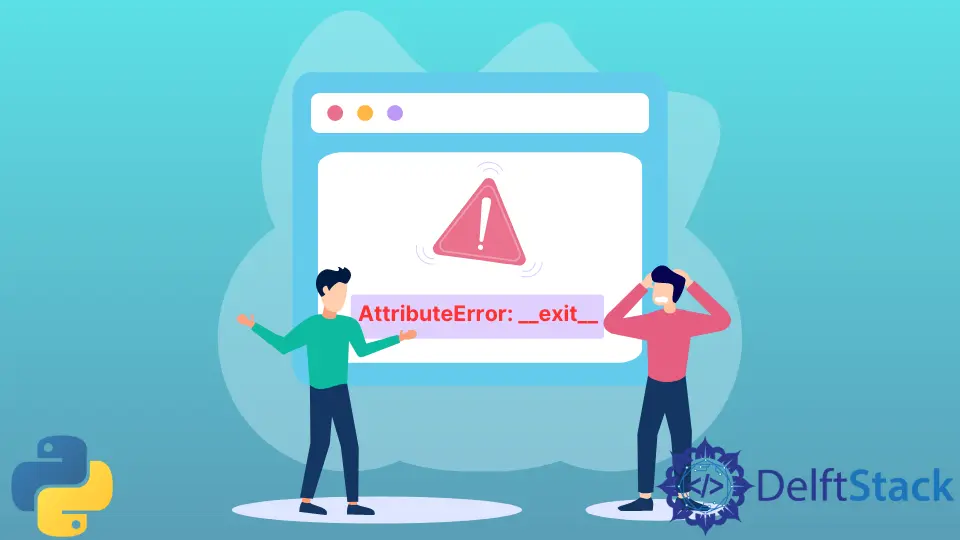
It is common to get errors when trying to develop a new program in Python. The AttributeError
is one of the most common errors in Python.
This error occurs when an attribute reference or assignment fails.
Sometimes when working with a Python program, you may get an error message like the below.
Traceback (most recent call last):
File "<string>", line 6, in <module>
AttributeError: __exit__
This is an error regarding the __exit__()
method. This is a type of AttributeError
.
In this article, we are going to see how we can resolve this AttributeError: __exit__
error, and also, we are going to discuss this topic by using relevant examples and explanations.
What Is the __exit__()
To understand this error, we first need to know what the __exit__()
is and how it works.
The __exit__()
is a method of ContextManager
class. It is used to release the resources occupied by the current code.
This method contains the instructions for closing the property of the resource handler so that the resource becomes free for the next use.
You need to supply the type, value, and traceback as a parameter for this method. These parameters are used by the method if any exception occurs.
If an exception or error occurs, the method __exit__()
returns a True
value; otherwise, it will return a False
.
How the AttributeError: __exit__
Occurs
We need to look at our example code to understand how this error occurs. On the code shared below we didn’t created the __exit__()
method inside the AttributeError()
class.
This class is a ContextManager
class.
class AttributeError:
def __enter__(self):
return "This is __Enter__, if you remove this, it will generate an error."
Error = AttributeError()
with Error as Obj:
print(Obj)
Now, if you try to execute the above example code, you will get the below error when executing.
Traceback (most recent call last):
File "main.py", line 6, in <module>
with Error as Obj:
AttributeError: __exit__
How to Resolve the AttributeError: __exit__
in Python
Now we understood how the AttributeError: __exit__
occurs. Now we need to learn how we can solve that error.
As we already discussed that the method __exit__()
is a method of the ContextManager
class, so we need to define the __exit__()
inside the class to resolve this error. Now our fixed version of the code will look like the below.
class AttributeError:
def __enter__(self):
return "This is __Enter__; if you remove this, it will generate an error."
def __exit__(self, exc_type, exc_val, exc_tb):
print("This is __Exit__; if you remove this, it will generate an error.")
Error = AttributeError()
with Error as Obj:
print(Obj)
Now, if you execute the above example code, you will see that the code is successfully executed and provide you with the below output.
This is __Enter__; if you remove this, it will generate an error.
This is __Exit__; if you remove this, it will generate an error.
Please take note that the commands and programs discussed here are written in the Python programming language.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python